Getting Started with Competitive Programming in Python
Last Updated :
01 Nov, 2023
Python is a great option for programming in Competitive Programming. First off, its easy-to-understand and concise grammar enables quicker development and simpler debugging. The huge standard library of Python offers a wide range of modules and functions that can be used to effectively address programming difficulties. Python also offers dynamic typing, intelligent memory management, and high-level data structures, which speed up development and simplify coding.
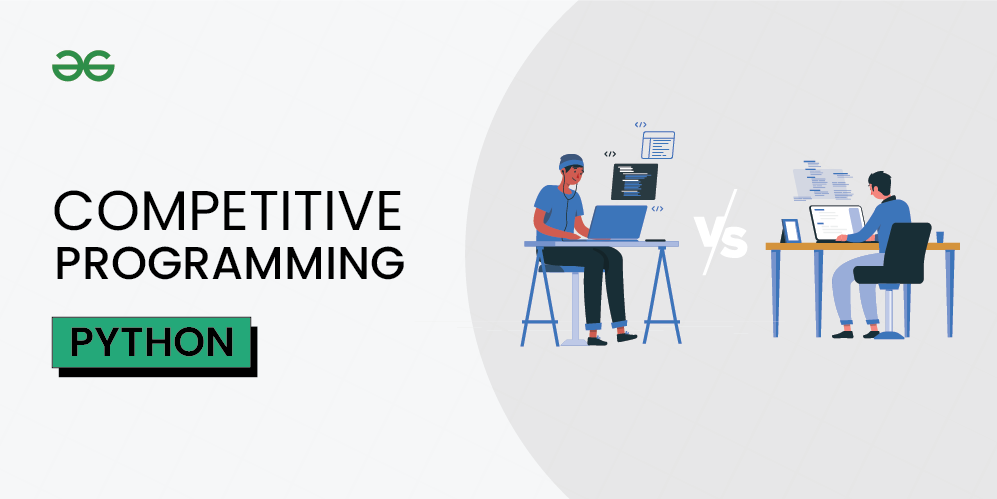
Table of Contents:
1. How to start Competitive Programming using Python:
- Learn Python language
- Python list and string manipulation:
- Learn Data Structures and Algorithms
- Implementing a simple linked list:
- Use Python Libraries
- Using NumPy for array manipulation:
- Optimize code step by step
- Brute-force approach to find the maximum element in a list:
- Take part in contests
- Solving a simple problem on GeeksForGeeks:
- Look for Better solutions
- Comparing two solutions to find the maximum element in a list:
- Practice Time Management
2. Examples:
- Binary Search Algorithm in Python:
- Solving the Knapsack Problem:
- DFS algorithm for graph traversal:
- Longest Increasing Subsequence problem using Dynamic Programming:
- Trie data structure:
3. Tips for improving in Competitive Programming: 4. Pros of Using Python for CP: 5. Cons of Using Python for CP: 6. Conclusion:
|
How to start Competitive Programming using Python:
1. Learn Python language
We should learn the basics of the Python language which contain syntax, datatypes, list, string, tuples, etc;
Python list and string manipulation:
Python3
numbers = [ 1 , 2 , 3 , 4 , 5 ]
print (numbers[ 0 ])
name = "John"
greeting = "Hello, " + name + "!"
print (greeting)
|
2. Learn Data Structures and Algorithms
Learn data structures and algorithms like arrays, linked lists, stacks, queues, trees, graphs, sorting, searching, and dynamic programming.
Implementing a simple linked list:
Python3
class Node:
def __init__( self , data):
self .data = data
self . next = None
class LinkedList:
def __init__( self ):
self .head = None
def append( self , data):
new_node = Node(data)
if not self .head:
self .head = new_node
else :
current = self .head
while current. next :
current = current. next
current. next = new_node
def print_list( self ):
current = self .head
while current:
print (current.data, end = " - > ")
current = current. next
print (" None ")
linked_list = LinkedList()
linked_list.append( 1 )
linked_list.append( 2 )
linked_list.append( 3 )
linked_list.print_list()
|
Output
1 -> 2 -> 3 -> None
3. Use Python Libraries
Python libraries such as NumPy, Pandas, SciPy, and Matplotlib are a few libraries for programming competitions.
Using NumPy for array manipulation:
Python
import numpy as np
arr = np.array([ 1 , 2 , 3 , 4 , 5 ])
print (np. sum (arr))
sorted_arr = np.sort(arr)
print (sorted_arr)
|
4. Optimize code step by step
Optimize your code starting with a brute force approach, a better solution, and at last best solution.
Brute-force approach to find the maximum element in a list:
Python3
def find_max_element(arr):
max_element = arr[ 0 ]
for num in arr:
if num > max_element:
max_element = num
return max_element
|
5. Take part in contests
Once you feel comfortable solving problems, participate in competitions like GeeksForGeeks, Google Code Jam, HackerRank, or Codeforces.
Solving a simple problem on GeeksForGeeks:
Python3
def find_max_element_1(arr):
return max (arr)
def find_max_element_2(arr):
max_element = arr[ 0 ]
for num in arr:
if num > max_element:
max_element = num
return max_element
|
6. Look for Better solutions
Review other people’s solutions after each problem or contest. Search for more elegant or effective solutions to these problems. You will increase your expertise by reading about and understanding the methods used by skilled programmers.
Comparing two solutions to find the maximum element in a list:
Python3
def find_max_element_1(arr):
return max (arr)
def find_max_element_2(arr):
max_element = arr[ 0 ]
for num in arr:
if num > max_element:
max_element = num
return max_element
|
7. Practice Time Management
Practice time management skills because programming competitions have time restrictions. Set time constraints for each challenge and concentrate on enhancing your accuracy and speed.
Examples:
1. Binary Search Algorithm in Python:
To find the element present in a list or not with O(logN) time complexity. It efficiently divides the search space in half at each step and divides down the search until the element is found or the search range is finished.
Python3
def binary_search(arr, x):
low = 0
high = len (arr) - 1
mid = 0
while low < = high:
mid = (high + low) / / 2
if arr[mid] < x:
low = mid + 1
elif arr[mid] > x:
high = mid - 1
else :
return mid
return - 1
arr = [ 2 , 3 , 4 , 10 , 40 ]
x = 10
result = binary_search(arr, x)
if result ! = - 1 :
print ("Element is present at index", str (result))
else :
print ("Element is not present in array")
|
Output
Element is present at index 3
Time Complexity: O(log N)
Auxiliary Space: O(1)
2. Solving the Knapsack Problem:
Returns the maximum value that can be put in a knapsack of capacity W. It evaluates the weight and value of each item and recursively finds two possibilities: include the current item or exclude it. The function returns the maximum value that can be put by selecting a subset of items within the weight capacity of the knapsack.
Python
def knapSack(W, wt, val, n):
if n = = 0 or W = = 0 :
return 0
if (wt[n - 1 ] > W):
return knapSack(W, wt, val, n - 1 )
else :
return max (val[n - 1 ] + knapSack(W - wt[n - 1 ], wt, val, n - 1 ),
knapSack(W, wt, val, n - 1 ))
val = [ 60 , 100 , 120 ]
wt = [ 10 , 20 , 30 ]
W = 50
n = len (val)
print knapSack(W, wt, val, n)
|
Time Complexity: O(2N)
Auxiliary Space: O(N), Space used for auxiliary stack space in recursion calls
3. DFS algorithm for graph traversal:
For graph traversal of a directed graph. It visits each vertex of the graph and visits its adjacent vertices recursively until all vertices are visited. The DFS traversal order is printed as the output.
Python
from collections import defaultdict
class Graph:
def __init__( self ):
self .graph = defaultdict( list )
def addEdge( self ,u,v):
self .graph[u].append(v)
def DFSUtil( self , v, visited):
visited[v] = True
print v,
for i in self .graph[v]:
if visited[i] = = False :
self .DFSUtil(i, visited)
def DFS( self ):
V = len ( self .graph)
visited = [ False ] * (V)
for i in range (V):
if visited[i] = = False :
self .DFSUtil(i, visited)
g = Graph()
g.addEdge( 0 , 1 )
g.addEdge( 0 , 2 )
g.addEdge( 1 , 2 )
g.addEdge( 2 , 0 )
g.addEdge( 2 , 3 )
g.addEdge( 3 , 3 )
print "Following is Depth First Traversal"
g.DFS()
|
Output
Following is Depth First Traversal
0 1 2 3
Time Complexity: O(V+E), where V is the number of vertices and E is the number of edges
Auxiliary Space: O(V)
4. Longest Increasing Subsequence problem using Dynamic Programming:
It calculates the length of the longest increasing subsequence in a given array using a bottom-up approach, where each LIS value is updated by considering all previous elements.
Python
def lis(arr):
n = len (arr)
lis = [ 1 ] * n
for i in range ( 1 , n):
for j in range ( 0 , i):
if arr[i] > arr[j] and lis[i]< lis[j] + 1 :
lis[i] = lis[j] + 1
maximum = 0
for i in range (n):
maximum = max (maximum, lis[i])
return maximum
arr = [ 10 , 22 , 9 , 33 , 21 , 50 , 41 , 60 ]
print "Length of list is ", lis(arr)
|
Output
Length of list is 5
Time Complexity: O(N2)
Auxiliary space: O(N)
5. Trie data structure:
Here is the implementation of a Trie data structure for efficient insertion and search operations. It creates a Trie, inserts keys into it, and performs searches to check the presence of keys in the Trie.
Python3
class TrieNode:
def __init__( self ):
self .children = [ None ] * 26
self .isEndOfWord = False
class Trie:
def __init__( self ):
self .root = self .getNode()
def getNode( self ):
return TrieNode()
def _charToIndex( self ,ch):
return ord (ch) - ord ( 'a' )
def insert( self ,key):
pCrawl = self .root
length = len (key)
for level in range (length):
index = self ._charToIndex(key[level])
if not pCrawl.children[index]:
pCrawl.children[index] = self .getNode()
pCrawl = pCrawl.children[index]
pCrawl.isEndOfWord = True
def search( self , key):
pCrawl = self .root
length = len (key)
for level in range (length):
index = self ._charToIndex(key[level])
if not pCrawl.children[index]:
return False
pCrawl = pCrawl.children[index]
return pCrawl.isEndOfWord
def main():
keys = ["the","a","there","anaswe"," any ",
"by","their"]
output = ["Not present in trie",
"Present in trie"]
t = Trie()
for key in keys:
t.insert(key)
print ("{} - - - - {}". format ("the",output[t.search("the")]))
print ("{} - - - - {}". format ("these",output[t.search("these")]))
print ("{} - - - - {}". format ("their",output[t.search("their")]))
print ("{} - - - - {}". format ("thaw",output[t.search("thaw")]))
if __name__ = = '__main__' :
main()
|
Output
the ---- Present in trie
these ---- Not present in trie
their ---- Present in trie
thaw ---- Not present in trie
Time Complexity: O(N)
Auxiliary Space: O(N)
Tips for improving in Competitive Programming:
- Practice regularly and consistently.
- Focus on problem-solving, not just coding.
- Use built-in functions and libraries to save time.
- Write clean, readable, and efficient code
Pros of Using Python for CP:
- Python’s clear syntax and simple-to-follow code make it the perfect language for competitive programming, allowing for speedy implementation and troubleshooting.
- Python has a sizable standard library that offers ready-to-use modules and functions, reducing the time and effort required to implement common tasks.
- Python has built-in data structures like dictionaries, lists, and sets that make it easier to manipulate and store data effectively while solving problems.
- Python’s interpreted nature enables speedy experimentation and prototyping, facilitating quicker algorithm development iterations.
Cons of Using Python for CP:
- Python’s interpreted nature can make it slower to execute than lower-level languages like C++ or Java. This could have an impact on performance in time-sensitive competitive programming scenarios.
- Python’s automatic memory management can result in greater memory use as compared to languages with human memory control, which can be problematic for issues requiring a lot of memory.
Conclusion:
By learning Python and practicing algorithmic problem-solving techniques, programmers can know the power of Python for competitive programming and enhance their coding skills.
Share your thoughts in the comments
Please Login to comment...