A Django model is the built-in feature that Django uses to create tables, their fields, and various constraints. In short, Django Models is the SQL Database one uses with Django. SQL (Structured Query Language) is complex and involves a lot of different queries for creating, deleting, updating, or any other stuff related to a database. Django models simplify the tasks and organize tables into models. Generally, each model maps to a single database table.
Django Models
Create a model in Django to store data in the database conveniently. Moreover, we can use the admin panel of Django to create, update, delete, or retrieve fields of a model and various similar operations. Django models provide simplicity, consistency, version control, and advanced metadata handling. The basics of a model include –
- Each model is a Python class that subclasses django.db.models.Model.
- Each attribute of the model represents a database field.
- With all of this, Django gives you an automatically generated database-access API; see Making queries.
Example
Python3
from django.db import models
class GeeksModel(models.Model):
title = models.CharField(max_length = 200 )
description = models.TextField()
|
This program defines a Django model called “GeeksModel” which has two fields: “title” and “description”. “title” is a character field with a maximum length of 200 characters and “description” is a text field with no maximum length. This model can be used to create and interact with a corresponding database table for storing “GeeksModel” objects. Without any additional code or context, running this program will not produce any visible output.
Django maps the fields defined in Django models into table fields of the database as shown below.
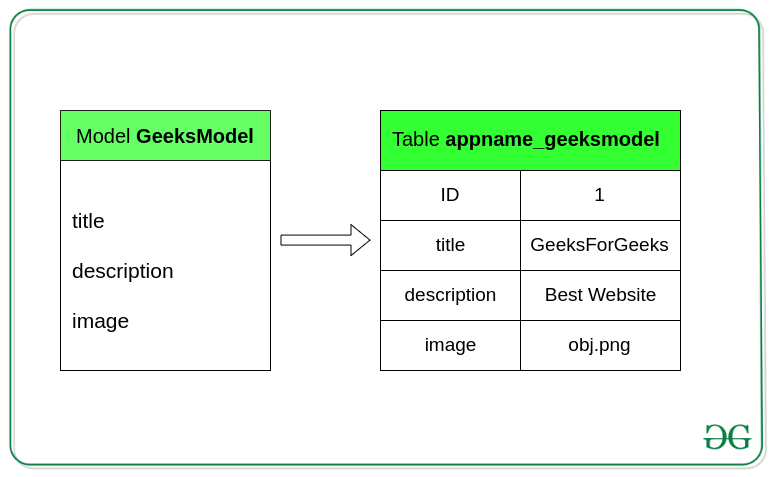
Model in Django
To create Django Models, one needs to have a project and an app working in it. After you start an app you can create models in app/models.py. Before starting to use a model let’s check how to start a project and create an app named geeks.py
Refer to the following articles to check how to create a project and an app in Django.
Create Model in Django
Syntax
from django.db import models
class ModelName(models.Model):
field_name = models.Field(**options)
To create a model, in geeks/models.py Enter the code,
Python3
from django.db import models
class GeeksModel(models.Model):
title = models.CharField(max_length = 200 )
description = models.TextField()
last_modified = models.DateTimeField(auto_now_add = True )
img = models.ImageField(upload_to = & quot
images / "
)
def __str__( self ):
return self .title
|
This code defines a new Django model called “GeeksModel” which has four fields: “title” (a character field with a maximum length of 200), “description” (a text field), “last_modified” (a date and time field that automatically sets the date and time of creation), and “img” (an image field that will be uploaded to a directory called “images”). The __str__ method is also defined to return the title of the instance of the model when the model is printed.
This code does not produce any output. It is defining a model class which can be used to create database tables and store data in Django.
Whenever we create a Model, Delete a Model, or update anything in any of models.py of our project. We need to run two commands makemigrations and migrate. makemigrations basically generates the SQL commands for preinstalled apps (which can be viewed in installed apps in settings.py) and your newly created app’s model which you add in installed apps whereas migrate executes those SQL commands in the database file.
So when we run,
Python manage.py makemigrations
SQL Query to create above Model as a Table is created and
Python manage.py migrate
creates the table in the database.
Now we have created a model we can perform various operations such as creating a Row for the table or in terms of Django Creating an instance of Model. To know more visit – Django Basic App Model – Makemigrations and Migrate
Render a Model in Django Admin Interface
To render a model in Django admin, we need to modify app/admin.py. Go to admin.py in geeks app and enter the following code. Import the corresponding model from models.py and register it to the admin interface.
Python3
from django.contrib import admin
from .models import GeeksModel
admin.site.register(GeeksModel)
|
This code imports the admin module from the django.contrib package and the GeeksModel class from the models module in the current directory. It then registers the GeeksModel class with the Django admin site, which allows the model to be managed through the Django admin interface. This means you can perform CRUD(Create, Read, Update, Delete) operations on the GeeksModel using the Django admin interface.
This code does not produce any output, it simply registers the GeeksModel with the admin site, so that it can be managed via the Django admin interface.
Now we can check whether the model has been rendered in Django Admin. Django Admin Interface can be used to graphically implement CRUD (Create, Retrieve, Update, Delete).
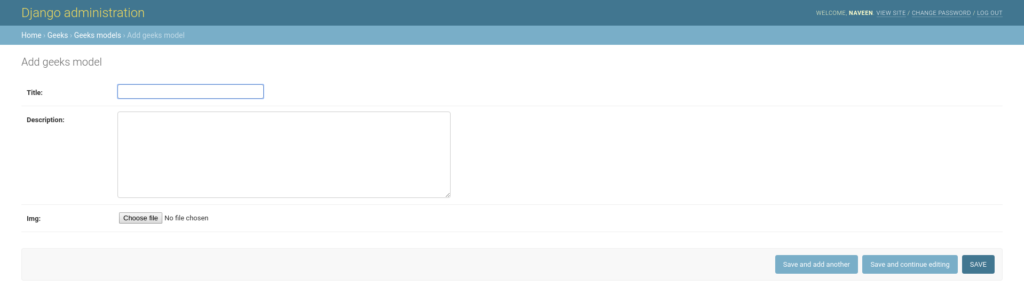
To check more on rendering models in django admin, visit – Render Model in Django Admin Interface
Django CRUD – Inserting, Updating and Deleting Data
Django lets us interact with its database models, i.e. add, delete, modify and query objects, using a database-abstraction API called ORM(Object Relational Mapper). We can access the Django ORM by running the following command inside our project directory.
python manage.py shell
Adding Objects
To create an object of model Album and save it into the database, we need to write the following command:
>>>> a = GeeksModel(
title = "GeeksForGeeks",
description = "A description here",
img = "geeks/abc.png"
)
>>> a.save()
Retrieving Objects
To retrieve all the objects of a model, we write the following command:
>>> GeeksModel.objects.all()
<QuerySet [<GeeksModel: Divide>, <GeeksModel: Abbey Road>, <GeeksModel: Revolver>]>
Modifying existing Objects
We can modify an existing object as follows:
>>> a = GeeksModel.objects.get(id = 3)
>>> a.title = "Pop"
>>> a.save()
Deleting Objects
To delete a single object, we need to write the following commands:
>>> a = Album.objects.get(id = 2)
>>> a.delete()
To check detailed post of Django’s ORM (Object) visit Django ORM – Inserting, Updating & Deleting Data
Validation on Fields in a Model
Built-in Field Validations in Django models are the default validations that come predefined to all Django fields. Every field comes in with built-in validations from Django validators. For example, IntegerField comes with built-in validation that it can only store integer values and that too in a particular range.
Enter the following code into models.py file of geeks app.
Python3
from django.db import models
from django.db.models import Model
class GeeksModel(Model):
geeks_field = models.IntegerField()
def __str__( self ):
return self .geeks_field
|
After running makemigrations and migrate on Django and rendering above model, let us try to create an instance using string “GfG is Best“.
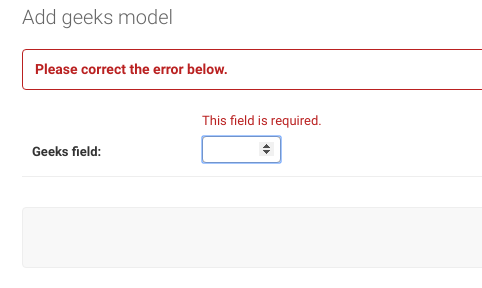
You can see in the admin interface, one can not enter a string in an IntegerField. Similarly every field has its own validations. To know more about validations visit, Built-in Field Validations – Django Models
More on Django Models –
Django Model data types and fields list
The most important part of a model and the only required part of a model is the list of database fields it defines. Fields are specified by class attributes. Here is a list of all Field types used in Django.
.math-table { border-collapse: collapse; width: 100%; } .math-table td { border: 1px solid #5fb962; text-align: left !important; padding: 8px; } .math-table th { border: 1px solid #5fb962; padding: 8px; } .math-table tr>th{ background-color: #c6ebd9; vertical-align: middle; } .math-table tr:nth-child(odd) { background-color: #ffffff; }
AutoField |
It An IntegerField that automatically increments. |
BigAutoField |
It is a 64-bit integer, much like an AutoField except that it is guaranteed to fit numbers from 1 to 9223372036854775807. |
BigIntegerField |
It is a 64-bit integer, much like an IntegerField except that it is guaranteed to fit numbers from -9223372036854775808 to 9223372036854775807. |
BinaryField |
A field to store raw binary data. |
BooleanField |
A true/false field. The default form widget for this field is a CheckboxInput. |
CharField |
It is string filed for small to large-sized input |
DateField |
A date, represented in Python by a datetime.date instance |
|
It is used for date and time, represented in Python by a datetime.datetime instance. |
DecimalField |
It is a fixed-precision decimal number, represented in Python by a Decimal instance. |
DurationField |
A field for storing periods of time. |
EmailField |
It is a CharField that checks that the value is a valid email address. |
FileField |
It is a file-upload field. |
FloatField |
It is a floating-point number represented in Python by a float instance. |
ImageField |
It inherits all attributes and methods from FileField, but also validates that the uploaded object is a valid image. |
IntegerField |
It is an integer field. Values from -2147483648 to 2147483647 are safe in all databases supported by Django. |
GenericIPAddressField |
An IPv4 or IPv6 address, in string format (e.g. 192.0.2.30 or 2a02:42fe::4). |
NullBooleanField |
Like a BooleanField, but allows NULL as one of the options. |
PositiveIntegerField |
Like an IntegerField, but must be either positive or zero (0). |
PositiveSmallIntegerField |
Like a PositiveIntegerField, but only allows values under a certain (database-dependent) point. |
SlugField |
Slug is a newspaper term. A slug is a short label for something, containing only letters, numbers, underscores or hyphens. They’re generally used in URLs. |
SmallIntegerField |
It is like an IntegerField, but only allows values under a certain (database-dependent) point. |
TextField |
A large text field. The default form widget for this field is a Textarea. |
TimeField |
A time, represented in Python by a datetime.time instance. |
URLField |
A CharField for a URL, validated by URLValidator. |
UUIDField |
A field for storing universally unique identifiers. Uses Python’s UUID class. When used on PostgreSQL, this stores in a uuid datatype, otherwise in a char(32). |
Relationship Fields
Django also defines a set of fields that represent relations.
ForeignKey |
A many-to-one relationship. Requires two positional arguments: the class to which the model is related and the on_delete option. |
ManyToManyField |
A many-to-many relationship. Requires a positional argument: the class to which the model is related, which works exactly the same as it does for ForeignKey, including recursive and lazy relationships. |
OneToOneField |
A one-to-one relationship. Conceptually, this is similar to a ForeignKey with unique=True, but the “reverse” side of the relation will directly return a single object. |
Field Options
Field Options are the arguments given to each field for applying some constraint or imparting a particular characteristic to a particular Field. For example, adding an argument null = True to CharField will enable it to store empty values for that table in relational database.
Here are the field options and attributes that an CharField can use.
Null |
If True, Django will store empty values as NULL in the database. Default is False. |
Blank |
If True, the field is allowed to be blank. Default is False. |
db_column |
The name of the database column to use for this field. If this isn’t given, Django will use the field’s name. |
Default |
The default value for the field. This can be a value or a callable object. If callable it will be called every time a new object is created. |
help_text |
Extra “help” text to be displayed with the form widget. It’s useful for documentation even if your field isn’t used on a form. |
primary_key |
If True, this field is the primary key for the model. |
editable |
If False, the field will not be displayed in the admin or any other ModelForm. They are also skipped during model validation. Default is True. |
error_messages |
The error_messages argument lets you override the default messages that the field will raise. Pass in a dictionary with keys matching the error messages you want to override. |
help_text |
Extra “help” text to be displayed with the form widget. It’s useful for documentation even if your field isn’t used on a form. |
verbose_name |
A human-readable name for the field. If the verbose name isn’t given, Django will automatically create it using the field’s attribute name, converting underscores to spaces. |
validators |
A list of validators to run for this field. See the validators documentation for more information. |
Unique |
If True, this field must be unique throughout the table. |
Share your thoughts in the comments
Please Login to comment...