The first argument is a URL pattern name. It can be a quoted literal or any other context variable. Additional arguments are optional and should be space-separated values that will be used as arguments in the URL.
url – Django template Tags Explanation
Illustration of How to use url tag in Django templates using an Example. Consider a project named geeksforgeeks
having an app named geeks
.
Refer to the following articles to check how to create a project and an app in Django.
Now create two views through which we will access the template,
In geeks/views.py
,
from django.shortcuts import render
def geeks_view(request):
return render(request, "geeks.html" )
def nav_view(request):
return render(request, "nav.html" )
|
Create a url path to map to this view. URLs need to have a name which then can be used in templates and with url tag. In geeks/urls.py
,
from django.urls import path
from .views import geeks_view, nav_view
urlpatterns = [
path( '1/' , geeks_view, name = "template1" ),
path( '2/' , nav_view, name = "template2" ),
]
|
Now we will create two templates to demonstrate now tag. Create a template in geeks.html
,
< html >
< h1 >Template 1</ h1 >
< a href = "{% url 'template2' %}" >Go to template 2</ a >
</ html >
|
Create a template in geeks.html
,
< html >
<< h2 >Template 2</ h2 >
< a href = "{% url 'template1' %}" >Go to template 1</ a >
</ html >
|
Now visit http://127.0.0.1:8000/1,
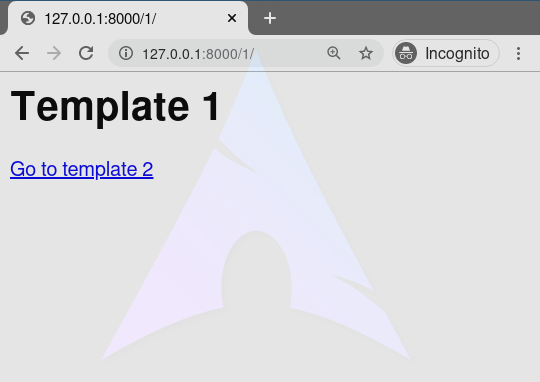
Click on the link and it will redirect to other url.
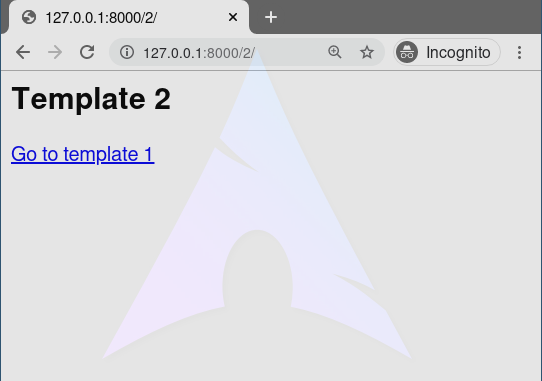
Advanced Usage
suppose you have a view, app_views.client, whose URLconf takes a client ID (here, client() is a method inside the views file app_views.py). The URLconf line might look like this:
path('client/<int:id>/', app_views.client, name='app-views-client')
If this app’s URLconf is included into the project’s URLconf under a path such as this:
path('clients/', include('project_name.app_name.urls'))
…then, in a template, you can create a link to this view like this:
{% url 'app-views-client' client.id %}
The template tag will output the string /clients/client/123/.
Share your thoughts in the comments
Please Login to comment...