How to use React Context with React-Redux ?
Last Updated :
19 Apr, 2024
React context with React-Redux is a popular state management library for React applications. Using React context with React-Redux is a powerful way to provide the Redux store to components deep within your component tree without manually passing it down through props.
Prerequisites
Approach:
- First, define a Redux store with a simple counter reducer to manage the state.
- Utilize React-Redux to connect Redux with React components.
- Create a context provider to make the Redux store available throughout the component tree.
- Use the useContext hook to access the Redux store within components.
- Dispatch actions to update the Redux state directly from React components.
Steps to Setup the Project:
Step 1:Â Create a reactJS application by using this command
npx create-react-app my-app
Step 2:Â Navigate to the project directory
cd my-app
Step 3:Â Install the necessary packages/libraries in your project using the following commands.
npm install react-redux redux
Project Structure:
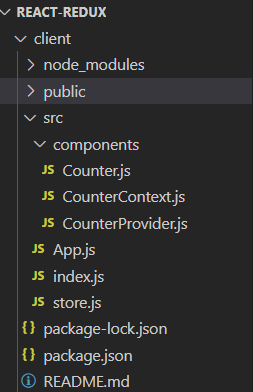
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"react-scripts": "5.0.1",
"redux": "^5.0.1",
"web-vitals": "^2.1.4"
},
Example: Implementation to show how to use react context with react redux using counter example.
JavaScript
// Counter.js
import React, { useContext } from 'react';
import { useDispatch, useSelector } from 'react-redux';
import CounterContext from './CounterContext';
const Counter = () => {
const store = useContext(CounterContext);
const count = useSelector(state => state.count);
const dispatch = useDispatch();
const handleIncrement = () => {
dispatch({ type: 'INCREMENT' });
};
const handleDecrement = () => {
dispatch({ type: 'DECREMENT' });
};
return (
<div>
<button onClick={handleDecrement}>-</button>
<span>{count}</span>
<button onClick={handleIncrement}>+</button>
</div>
);
};
export default Counter;
JavaScript
// store.js
import { createStore } from 'redux';
const initialState = {
count: 0,
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return {
...state,
count: state.count + 1,
};
case 'DECREMENT':
return {
...state,
count: state.count - 1,
};
default:
return state;
}
};
const store = createStore(counterReducer);
export default store;
JavaScript
// App.js
import React from 'react';
import Counter from './components/Counter';
import CounterProvider from './components/CounterProvider';
const App = () => {
return (
<CounterProvider>
<div>
<h1>Counter App</h1>
<Counter />
</div>
</CounterProvider>
);
};
export default App;
JavaScript
// CounterContext.js
import { createContext } from 'react';
const CounterContext = createContext(null);
export default CounterContext;
JavaScript
// CounterProvider.js
import React from 'react';
import { Provider } from 'react-redux';
import store from '../store';
import CounterContext from './CounterContext';
const CounterProvider = ({ children }) => {
return (
<CounterContext.Provider value={store}>
<Provider store={store}>
{children}
</Provider>
</CounterContext.Provider>
);
};
export default CounterProvider;
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(
<App />,
document.getElementById('root')
);
Step to Run Application:Â Run the application using the following command from the root directory of the project
npm start
Output:Â Your project will be shown in the URL http://localhost:3000/
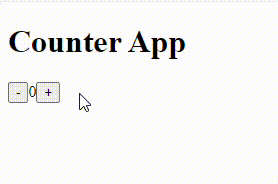
Share your thoughts in the comments
Please Login to comment...