Other Hooks in React
Last Updated :
21 Feb, 2024
React provides specialized built-in hooks for building reusable components and libraries. While they are used less, these hooks are important in providing library authors to create robust and user-friendly applications. Among these specialized hooks are useDebugValue
and useId
, that provides unique functionalities that enhance the developer experience and facilitate the creation of reusable and accessible components. In this article, we’ll look into these hooks.
We will learn about the following Other Hooks in React
useDebugValue hooks
useDebugValue
is a React Hook that lets you add a label to a custom Hook in React DevTools. The hook allows library authors to customize the label displayed by React DevTools for custom hooks.
Syntax:
useDebugValue(value, format?)
ParametersÂ
value
: The value you want to display in React DevTools. It can have any type.
- optionalÂ
format
: A formatting function. When the component is inspected, React DevTools will call the formatting function with the value
 as the argument, and then display the returned formatted value.
Return Type:
useDebugValue
do not return anything.
Usage
- Adding a label to a custom Hook
- Deferring formatting of a debug value
Example: In this example, useDebugValue
is used inside the useFetchData
custom hook to provide debugging information about the loading status.
Javascript
import React, { useState, useEffect, useDebugValue } from 'react' ;
function useFetchData(url) {
const [data, setData] = useState( null );
const [loading, setLoading] = useState( true );
useEffect(() => {
async function fetchData() {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
} catch (error) {
console.error( 'Error fetching data:' , error);
} finally {
setLoading( false );
}
}
fetchData();
}, [url]);
useDebugValue(loading ? 'Loading...' : 'Data loaded' );
return data;
}
function App() {
const data = useFetchData(url);
if (!data) {
return (
<div style={{ display: 'flex' , justifyContent: 'center' , alignItems: 'center' , height: '100vh' }}>
<div style={{ fontSize: '24px' }}>Loading...</div>
</div>
);
}
return (
<div>
<h1>Data Loaded!</h1>
<pre>{JSON.stringify(data, null , 2)}</pre>
</div>
);
}
export default App;
|
Output:
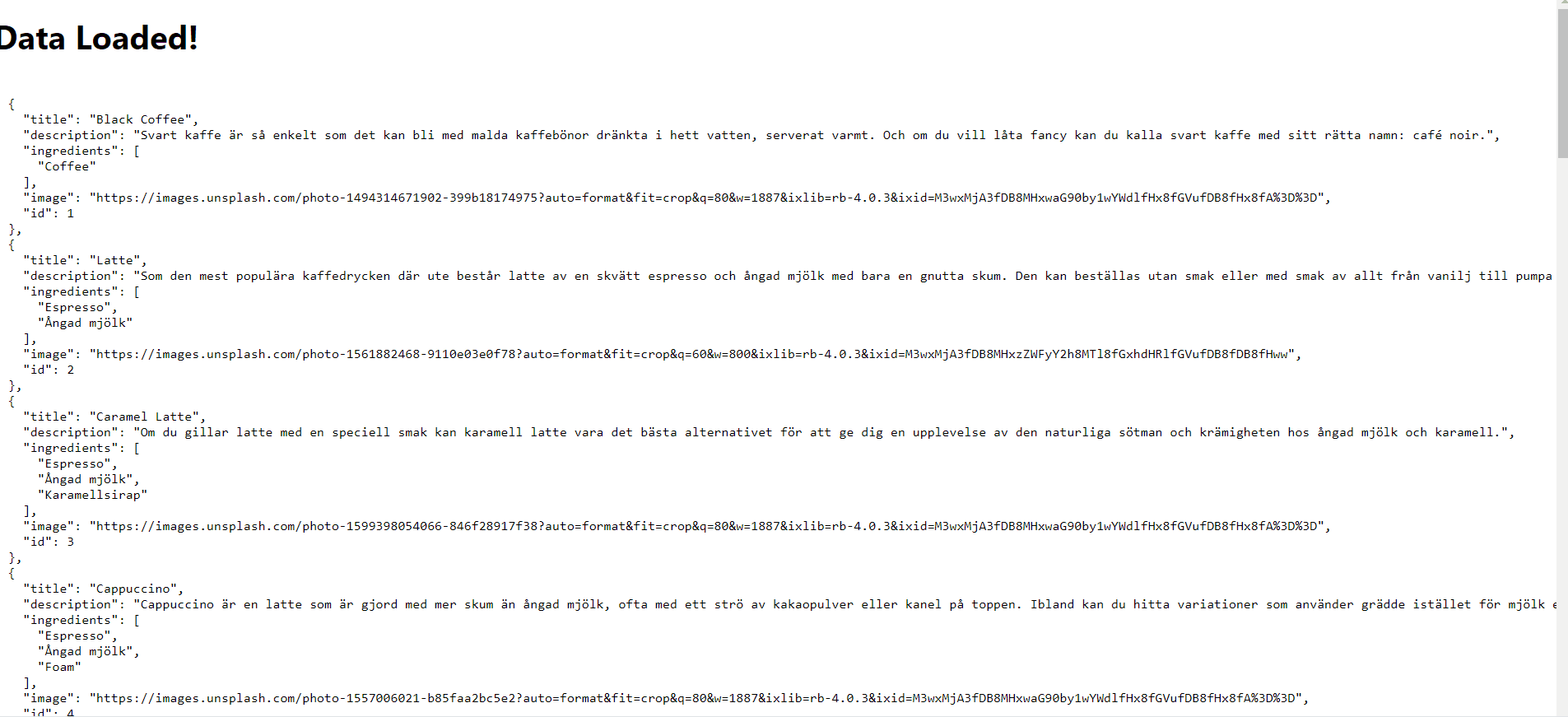
Output
useId hooks
useId
is a React Hook for generating unique IDs that can be passed to accessibility attributes. This hook helps components to become a unique identifier. By generating and managing unique IDs dynamically, components can enhance accessibility by ensuring proper labeling and identification, particularly for interactive elements.
Syntax:
const id = useId()
Parameters:
useId
does not take any parameters.
Returns:
useId
returns a unique ID string associated with this particular useId
call in this particular component.
Usage
- Generating unique IDs for accessibility attributes
- Generating IDs for several related elements
- Specifying a shared prefix for all generated IDs
- Using the same ID prefix on the client and the server
Example: In this example we are providing same id’s to both password and confirm password to display the same content.
Javascript
import { useId } from 'react' ;
function PasswordField() {
const passwordHintId = useId();
return (
<>
<label>
Password:
<input
type= "password"
aria-describedby={passwordHintId}
/>
</label>
<p id={passwordHintId}>
The password should contain at least 18 characters
</p>
</>
);
}
export default function App() {
return (
<>
<h2>Choose password</h2>
<PasswordField />
<h2>Confirm password</h2>
<PasswordField />
</>
);
}
|
Output:

Share your thoughts in the comments
Please Login to comment...