Chakra UI Typography Highlight
Last Updated :
12 Feb, 2024
Chakra UI Typography Highlight Component is used to highlight any part of text. It is a perfect component to use if you want to attract user’s attention to any specific part of the text or if you want to highlight some import parts of a paragraph.
The text to highlight can be selected using the query prop of the Highlight Component while its styles prop is used to style the highlighted section of the text. If you want to highlight more than one substring then an array can also be passed to the query prop. Also the value passed to the query prop is case insensitive, i.e both GeeksforGeeks and geeksforgeeks is same for it.
We will use the following approaches to implement Typography Highlight
Prerequisites:
Approach 1: Without using the useHighlight hook:
We will create Highlight Component to highlight specific part of text. We will also pass an array as query to highlight multiple parts of a text.
Setting up React Application and Installing Chakra UI:
Step 1: Create a react application using the create-react-app.
npx create-react-app my-chakraui-app
Step 2: Move to the created project folder (my-chakraui-app).
cd my-chakraui-app
Step 3: After Creating the react app install the needed packages using below command
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
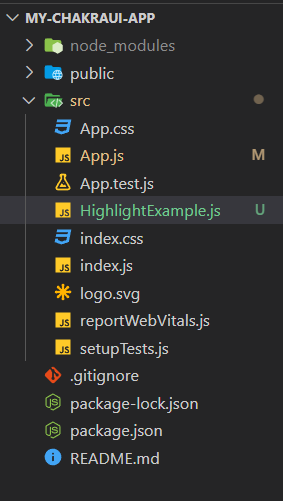
The updated dependencies in the package.json file are:
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example:Â Below are the code snippets for the respective files.
Javascript
import { ChakraProvider, Text } from "@chakra-ui/react" ;
import "./App.css" ;
import HighlightExample from "./HighlightExample" ;
function App() {
return (
<ChakraProvider>
<Text
color= "#2F8D46"
fontSize= "2rem"
textAlign= "center"
fontWeight= "600"
mt= "1rem"
>
GeeksforGeeks
</Text>
<Text
color= "#000"
fontSize= "1rem"
textAlign= "center"
fontWeight= "500"
mb= "2rem"
>
Chakra UI Typography Highlight
</Text>
<HighlightExample />
</ChakraProvider>
);
}
export default App;
|
Javascript
import React from "react" ;
import { Box, Highlight, Text } from "@chakra-ui/react" ;
export default function HighlightExample() {
return (
<Box
display= "flex"
flexDirection= "column"
gap= "40px"
alignItems= "center"
>
<Box>
<Text mb= "15px" fontWeight= "bold" >
Single Highlight:{ " " }
</Text>
<Highlight
query= "geeksforgeeks"
styles={{
px: "1" ,
py: "1" ,
bg: "green.200" ,
}}
>
GeeksforGeeks is awesome
</Highlight>
</Box>
<Box>
<Text mb= "15px" fontWeight= "bold" >
Multiple Highlights:{ " " }
</Text>
<Highlight
query={[ "geeksforgeeks" , "awesome" ]}
styles={{
px: "1" ,
py: "1" ,
bg: "green.200" ,
}}
>
GeeksforGeeks is awesome
</Highlight>
</Box>
</Box>
);
}
|
Start your application using the following command.
npm start
Output: Go to http://localhost:3000 in your browser to see the live app.
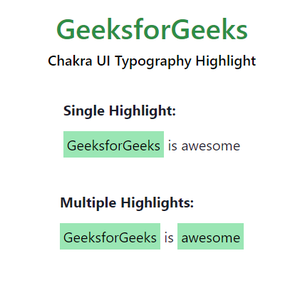
Approach 2: Using the useHighlight hook:
In this approach, we will use the useHighlight hook. This hook is used when we want to use the same type of highlights at many places. This helps us to write less redundant code. Project structure and setup is going to be same for this approach. We will change the contents of the HightlightExample.js file.
Javascript
import React from "react" ;
import { Box, useHighlight } from "@chakra-ui/react" ;
export default function HighlightExample2() {
const hookHighlight = useHighlight({
text: `We will be using the useHighlight
hook to highlight some words in this example.`,
query: [ "useHighlight" , "highlight" , "example" ],
});
return (
<Box display= "flex" justifyContent= "center" >
{hookHighlight.map(({ match, text }) => {
if (match === false ) return text;
return (
<Box
bgColor= "green"
color= "white"
padding= "0 2px"
margin= "0 2px"
>
{ " " }
{text}
</Box>
);
})}
</Box>
);
}
|
Output:
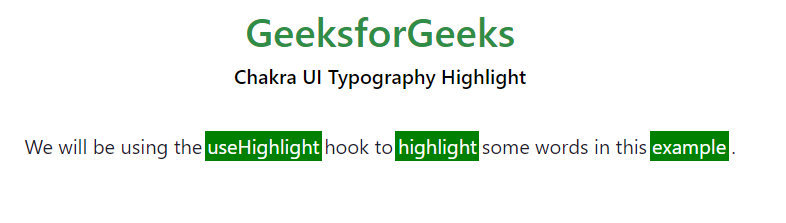
Share your thoughts in the comments
Please Login to comment...