Can you explain what the useState hook does in React Hooks?
Last Updated :
19 Feb, 2024
The useState hook is a feature in React Hooks that allows you to add state to functional components. It creates state variables and manages their values within a functional component.
Why is useState important?
Before introducing hooks in React, state management was only possible in class components. However, with the useState hook, users can now incorporate state into functional components, simplifying component logic and making managing state easier.
How do you use the useState hook?
To use the useState hook, you import it from the React library. Then, within your functional component, you call useState and pass in the initial value for your state variable. This hook returns an array containing the current state value and a function to update that value. You can use array destructuring to assign these values to variables.
Example: Below is the example of useState hook.
Javascript
import React, { useState } from 'react' ;
import './Counter.css' ;
function Counter() {
const [count, setCount] = useState(0);
return (
<div className= "counter-container" >
<p className= "counter-text" >
You clicked {count} times
</p>
<button className= "counter-button"
onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
|
CSS
.counter-container {
text-align : center ;
}
.counter-text {
font-size : 18px ;
margin-bottom : 10px ;
}
.counter-button {
padding : 10px 20px ;
font-size : 16px ;
background-color : #007bff ;
color : #fff ;
border : none ;
border-radius: 5px ;
cursor : pointer ;
}
.counter-button:hover {
background-color : #0056b3 ;
}
|
Output:
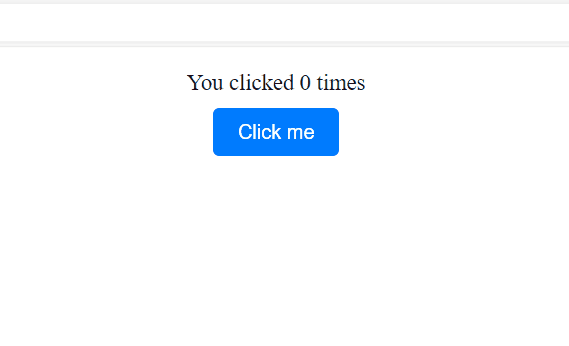
Output
How does useState differ from traditional class-based state?
In class components, state is managed using the this.state
object and this.setState()
method. With functional components and the useState hook, state is managed directly within the component function using the state variable and the function returned by useState to update it. This simplifies the syntax and makes state management more concise and readable.
Can you use useState multiple times in a single component?
Yes, you can use useState multiple times in a single component to manage different pieces of state independently. Each call to useState creates a separate state variable and update function pair, allowing you to manage multiple state values within the same component.
Share your thoughts in the comments
Please Login to comment...