How to handle Suspense with Hooks in React ?
Last Updated :
26 Feb, 2024
Suspense is a feature that allows components to suspend rendering while waiting for some asynchronous operation to resolve, such as data fetching. With React Hooks, you can use Suspense along with the useEffect
hook for handling asynchronous operations.
Handling Suspense with Hooks:
- We define a
fetchData
a function that returns a Promise, simulating data fetching with a delay of 2 seconds.
- We use the
useState
hook to manage the state of the fetched data (data
).
- We use the
useEffect
hook to fetch data when the component mounts.
- Inside the
useEffect
, we call the fetchData
function and update the state with the fetched data.
- We use the
Suspense
component from React to wrap the part of the UI that depends on the asynchronous operation (data
fetching).
- We provide a
fallback
prop to the Suspense
component, which specifies the UI to render while waiting for the data to be fetched. In this case, it’s a simple “Loading…” message.
- Inside the
Suspense
component, we conditionally render the data if it’s available (data ? <p>{data}</p> : null
).
Example: Below is an example of handling suspense with Hooks.
Javascript
import React, {
useState,
useEffect,
Suspense
} from 'react' ;
const fetchData = () => {
return new Promise(resolve => {
setTimeout(() => {
resolve([
{
id: 1,
name: 'GeeksforGeeks'
},
{
id: 2,
name: 'GeeksforGeeks Web Tech'
},
{
id: 3,
name: 'GFG Mern Stack'
}
]);
}, 2000);
});
};
const DataFetchingComponent = () => {
const [data, setData] = useState( null );
useEffect(() => {
fetchData()
.then(data => setData(data));
}, []);
const example =
data ? data[0] : null ;
return (
<div>
<h2>Data Fetching Component</h2>
<Suspense fallback={<div>Loading...</div>}>
{
example ? (
<div>
<h3>Example:</h3>
<p>ID: {example.id}</p>
<p>Name: {example.name}</p>
</div>
) : null
}
</Suspense>
</div>
);
};
export default DataFetchingComponent;
|
Output:
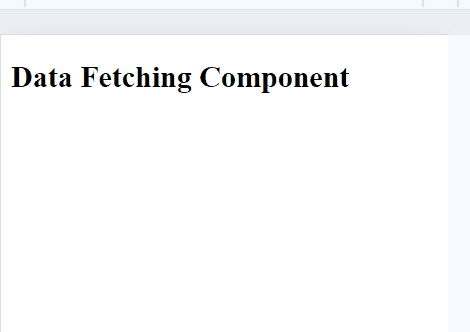
Output
Share your thoughts in the comments
Please Login to comment...