What is Reselect and how does it Works in React JS ?
Last Updated :
17 Dec, 2023
In React JS, Reselect is a library that enhances the efficiency of data computation through selectors. Primarily used in conjunction with Redux, a state management library for React, Reselect facilitates the creation of memoized selectors. This article explores Reselect and its various functions, with a focus on the `createSelector` function. `createSelector` is the primary function within the Reselect library, utilized for generating memoized selectors in Redux applications.
Prerequisites:
Syntax:
import { createSelector } from 'reselect';
const mySelector = createSelector(
[input1, input2, ...],
output1, output2, ...) => {
//compute output
};
);
Steps to Create React Application And Installing Module:
Step 1: Create a React Application using the following command:
npx create-react-app reselectdemo
Step 2: After creating your project folder i.e. reselectdemo, move to it using the following command:
cd reselectdemo
Step 3: Installing the Required Packages library in your project using the following command:
npm install reselect redux react-redux
Project Structure:
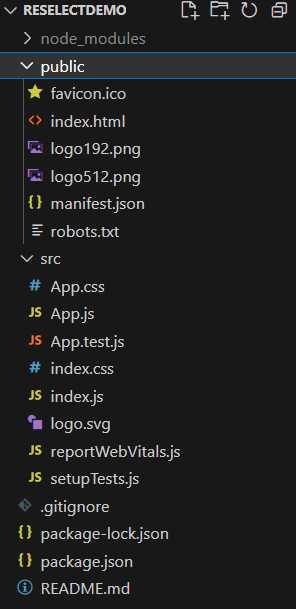
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^8.1.3",
"react-scripts": "5.0.1",
"redux": "^4.2.1",
"reselect": "^5.0.1",
"web-vitals": "^2.1.4",
}
Example 1: In the given illustration, the Counters component employs Reselect selectors, namely getSquare and getCube, to compute derived data from the Redux store’s calculated value.
Javascript
import React from "react" ;
import { Provider } from "react-redux" ;
import Counters from "./Counters" ;
import store from "./store" ;
function App() {
return (
<>
<Provider store={store}>
<Counters />
</Provider>
</>
);
}
export default App;
|
Javascript
import React from 'react' ;
import { connect } from 'react-redux' ;
import { getSquare, getCube } from './selectors' ;
const Counter = ({ count, square, cube, increment }) => {
return (
<div>
<p>Count: {count}</p>
<p>Square: {square}</p>
<p>Cube: {cube}</p>
<button onClick={increment}>
Increment
</button>
</div>
);
};
const mapStateToProps = state => {
return {
count: state.count,
square: getSquare(state),
cube: getCube(state)
};
};
const mapDispatchToProps = dispatch => {
return {
increment: () => dispatch({ type: 'INCREMENT' })
};
};
export default connect(mapStateToProps,
mapDispatchToProps)(Counter);
|
Javascript
import { createSelector } from 'reselect' ;
const getCount = state => state.count;
export const getSquare = createSelector(
[getCount],
count => count * count
);
export const getCube = createSelector(
[getCount],
count => count * count * count
);
|
Javascript
import { createStore } from 'redux' ;
import counterReducer from './counterReducer' ;
const store = createStore(counterReducer);
export default store;
|
Javascript
const initialState = {
count: 0
};
const counterReducer =
(state = initialState, action) => {
switch (action.type) {
case 'INCREMENT' :
return {
...state,
count: state.count + 1
};
default :
return state;
}
};
export default counterReducer;
|
Step to Run: Run the Application using the following command from the root directory of the project.
npm start
Output:
.gif)
Output
Example 2: In this example, we have a list of users with their names and ages. The initial filter value is set to 30 in the App.js
component using the setFilter
action. The getFilteredUsers
selector filters the users based on their age, only including users with an age greater than or equal to the filter value.
Javascript
import React from 'react' ;
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import { Provider } from 'react-redux' ;
import store from './store' ;
import App from './App' ;
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById( 'root' )
);
|
Javascript
import React, { useEffect } from 'react' ;
import { useSelector, useDispatch } from 'react-redux' ;
import { setFilter } from './actions' ;
import { getFilteredUsers } from './selectors' ;
const App = () => {
const dispatch = useDispatch();
useEffect(() => {
dispatch(setFilter(30));
}, [dispatch]);
const filteredUsers = useSelector(getFilteredUsers);
return (
<div>
<h1>Filtered Users:</h1>
<ul>
{filteredUsers.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
};
export default App;
|
Javascript
import { createStore } from 'redux' ;
import userReducer from './reducers' ;
const store = createStore(userReducer);
export default store;
|
Javascript
const initialState = {
users: [
{ id: 1, name: 'John' , age: 25 },
{ id: 2, name: 'Jane' , age: 30 },
{ id: 3, name: 'Alice' , age: 28 },
{ id: 4, name: 'Bob' , age: 32 }
],
filter: 30
};
export default function userReducer(
state = initialState, action) {
switch (action.type) {
case 'SET_FILTER' :
return {
...state,
filter: action.payload
};
default :
return state;
}
}
|
Javascript
export const setFilter = filter => ({
type: 'SET_FILTER' ,
payload: filter
});
|
Javascript
import { createSelector } from 'reselect' ;
const getUsers = state => state.users;
const getFilter = state => state.filter;
export const getFilteredUsers = createSelector(
[getUsers, getFilter],
(users, filter) => {
return users.filter(user => user.age >= filter);
}
);
|
Output:
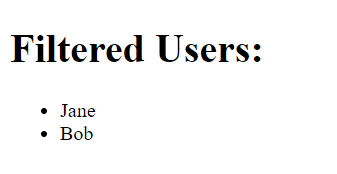
Share your thoughts in the comments
Please Login to comment...