Handling Error using React useState and useEffect Hooks
Last Updated :
08 Mar, 2024
In React, handling error states is very important to provide a smooth user experience and handle unexpected errors that may occur during data fetching or asynchronous operations. By using the useState and useEffect hooks, you can easily manage error states in your React applications.
In this article, we’ll explore how to handle error states using the useState
and useEffect
hooks in React.
What are Error States?
Error states represent situations where something unexpected happens in an application. These errors commonly occur during asynchronous operations such as fetching data from an API, processing user input, or rendering components. Common reasons of errors include network failures, server errors, and unexpected data formats.
Using useState and useEffect Hooks
React provides the useState
and useEffect
hooks, which are fundamental for managing state and side effects in functional components. These hooks helps us to handle error states efficiently by updating component state in response to errors and triggering error-related side effects.
Approach to handle Error States:
To use useState and useEffect , import it from the react library at the top of your component file:
import React, { useState,useEffect } from 'react';
Within your functional component, call useState with the initial state value as an argument. It returns an array containing two elements:
- The Current state value: Use this in your JSX to display the data dynamically.
- A State update function: Call this function to modify the state and trigger a re-render of the component.
React useState Hook Syntax:
const [error, setError] = useState(null);
The useEffect hook syntax accepts two arguments where the second argument is optional
React useEffect Hook Syntax:
useEffect(<FUNCTION>, <DEPENDECY>)
- FUNCTION: contains the code to be executed when useEffect triggers.
- DEPENDENCY: is an optional parameter, useEffect triggers when the given dependency is changed.
Steps to Create React App and handle Errors:
Step 1: Create a react application using the following command.
npx create-react-application my-react-application
Step 2: Navigate to the root directory of your application using the following command.
cd my-react-application
Project Structure:
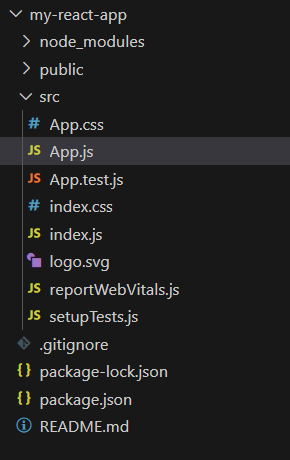
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example:In case of an error, it will render an error message with details. Once data is successfully fetched, it will render the fetched data or UI components accordingly.
Javascript
import React, { useState, useEffect } from 'react' ;
const ErrorHandling = () => {
const [data, setData] = useState( null );
const [error, setError] = useState( null );
const [loading, setLoading] = useState( true );
useEffect(() => {
fetchData()
.then(data => {
setData(data);
setLoading( false );
})
. catch (error => {
setError(error);
setLoading( false );
});
}, []);
const fetchData = async () => {
try {
const response = await fetch
if (!response.ok) {
throw new Error( 'Failed to fetch data' );
}
return response.json();
} catch (error) {
throw new Error( 'Failed to fetch data' );
}
};
if (loading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error.message}</div>;
}
if (!data) {
return <div>No data available</div>;
}
return (
<div>
<h1>Fetched Data</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.title}</li>
))}
</ul>
</div>
);
};
export default ErrorHandling;
|
Output:
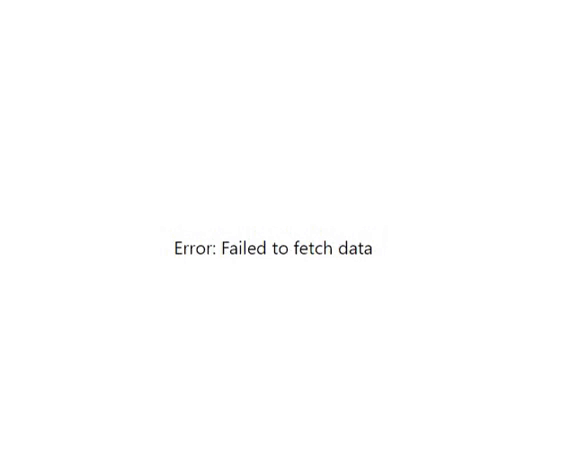
OutPut for Error State
- If Request is Succeed then list of data is shown:
.gif)
OutPut if request succeeds
Share your thoughts in the comments
Please Login to comment...