Fetching Data from an API with useEffect and useState Hook
Last Updated :
26 Feb, 2024
In modern web development, integrating APIs to fetch data is a common task. In React applications, the useEffect and useState hooks provide powerful tools for managing asynchronous data fetching. Combining these hooks enables fetching data from APIs efficiently.
This article explores how to effectively utilize these hooks to fetch data from an API, focusing on the Random Data API for practical examples
useState Hook:
React useState Hook allows to store and access of the state in the functional components. State refers to data or properties that need to be stored in an application.
Syntax of useState Hook:
const [state, setState] = useState(initialState);
useEffect Hook:
React useEffect hook handles the effects of the dependency array. It is called every time any state if the dependency array is modified or updated.
Syntax of useEffect Hook:
useEffect(() => {
// Effect code here
return () => {
// Cleanup code here (optional)
};
}, [dependencies]);
Approach to Fetch Data from API:
- Initialize state variables using useState.
- Utilize useEffect to trigger data fetching.
- Handle API calls within the useEffect callback.
- Update state with fetched data.
Fetching Random User Data
In this example, we’ll create a React component called RandomUserData that fetches random user data from the Random Data API. The component will utilize the useState and useEffect hooks to manage state and handle the data fetching process respectively. Once the data is fetched, it will be displayed on the screen.
- We import React, useState, and useEffect from the ‘react’ package.
- The RandomUserData functional component is created to encapsulate the logic for fetching and displaying random user data.
- We define a state variable userData using the useState hook, initialized to null as we initially don’t have any user data.
- Within the useEffect hook, we perform a fetch request to the Random Data API endpoint that provides random user data.
- Upon receiving a response, we convert it to JSON format and update the userData state with the fetched data using the setUserData function.
- In the component’s return statement, we conditionally render user information once it’s available. This prevents errors due to accessing undefined data before the fetch completes.
Example: This example implements the above-mentioned approach
Javascript
import React,
{
useState,
useEffect
} from 'react' ;
function RandomUserData() {
const [userData, setUserData] = useState( null );
useEffect(() => {
.then(response => response.json())
.then(data => setUserData(data));
}, []);
return (
<div>
{userData && (
<div>
<h2>User Information</h2>
<p>
Name:
{userData.first_name}
{userData.last_name}
</p>
<p>
Email: {userData.email}
</p>
{ }
</div>
)}
</div>
);
}
export default RandomUserData;
|
Output:
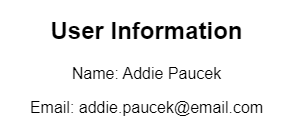
Fetching Random User Data Output
Fetching Random User Data List
In this example, we’ll create a React component called RandomUserList that fetches a list of random users from the Random Data API. The component will utilize the useState and useEffect hooks to manage state and handle the data fetching process respectively. Once the data is fetched, it will be displayed on the screen as a list of users.
- The RandomUserList component is created as a functional component to encapsulate the logic for fetching and displaying random user data.
- We define a state variable userList using the useState hook, initialized to an empty array as we initially don’t have any user data.
- Within the useEffect hook, a fetch request is made to the Random Data API endpoint that provides a list of random users. We use the query parameter size=5 to specify that we want to fetch 5 random users.
- Upon receiving the response, we parse the JSON and update the userList state with the fetched user data using the setUserList function.
- In the component’s return statement, we map through the userList array and display each user’s information in a list format.
Example: This example implements the above-mentioned approach
Javascript
import React,
{
useState,
useEffect
} from 'react' ;
function RandomUserList() {
const [userList, setUserList] = useState([]);
useEffect(() => {
.then(response => response.json())
.then(data => setUserList(data));
}, []);
return (
<div>
<h2>Random User List</h2>
<ul>
{userList.map(user => (
<li key={user.id}>
<p>
Name:
{user.first_name}
{user.last_name}
</p>
<p>
Email:
{user.email}
</p>
{ }
</li>
))}
</ul>
</div>
);
}
export default RandomUserList;
|
Output:
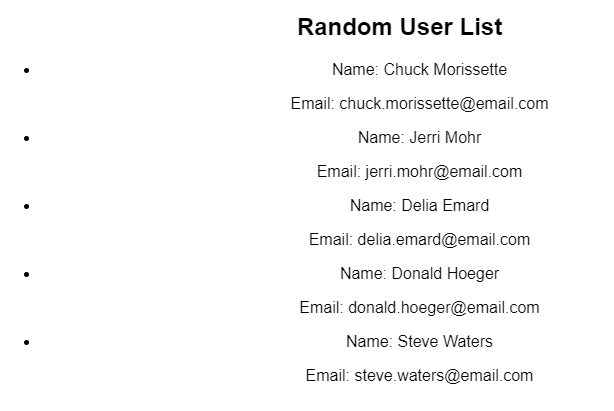
Fetching Random User Data List Output
Share your thoughts in the comments
Please Login to comment...