Benefits of using useCallback Hook in React
Last Updated :
28 Mar, 2024
The “useCallback” is a React Hook for optimizing performance in React functional components. “useCallback” can be utilized to enhance the performance of React applications by memorizing the callback function and minimizing unnecessary re-renders of components.
What is useCallback Hook?
In React, the “useCallback” hook memorizes a callback function. Memoization is a technique used to optimize performance by storing the result of expensive function calls and returns the cached result when the same input occurs again. In React, the “useCallback” is to optimize the performance of functional components by ensuring that the callback function reference remains stable across re-renders, as long as its dependencies remain unchanged.
How to use useCallaback in a ReactJS project?
import { useCallback } from 'react';
Benefits of using “useCallback” Hook:
There are the several benefits provide by the “useCallback” Hook in React. I have explained some important benefits.
- Performance Optimization: One of the primary benefits of “useCallback” is performance optimization. “useCallback” memozies the provided function instance so that it is not recreated on each render unless its dependencies change. It can be especially useful when you want to prevent unnecessary re-renders of child components that rely on callback function passed from the parent component.
- Optimzing Dependency Arrays: The second argument of “useCallback” is an array of dependencies. By specifying dependencies, you can control when the memoied callback function is recreated.
- Enhancing Code Readablity: By explicitly using “useCallback”, you make it clear to other developers that function being memorized is intended to be stable and should not change unnecessarily. This can improve code readablity and maintainability, especially in larger codebases or when working in a team.
Where to “useCallback” Hook:
The “useCallback” hook should generally be used in scenarios where you need to memorize callback functions to optimize performance and prevent unnecessary re-renders of components. I have explained some use case of “useCallback” Hook in React.
- Passing Callback to Child Components: When you passing callback props to child components, It’s often a good idea to use “useCallback” Hook to memorize those callbacks. This will ensures that child components only re-renders when necessary, improving performance.
- Event Handlers: If you are passing event handelrs to event listeners ( such as “onClick”, “onChange” etc), using ‘useCallback” can optimize performance by preventing unnecessary function recreations.
- Preventing Unwanted Effects in useEffect: When using the useEffect hook, you might need to pass a callback function as a dependency. In such cases, using useCallback can prevent unintended effects caused by the recreation of the callback function.
Steps to Create the React App:
Step 1: Set up React Project using the following command:
npx create-react-app project_name
Step 2: Navigate to the project folder using the following command.
cd client
Step 3: Run the Project using the following command.
npm start
Project Structure:
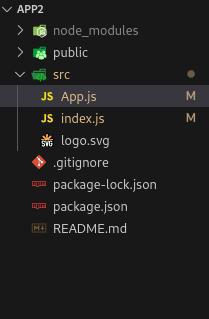
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: In this example, we create a state using the “useState” Hook and update the state using the handleClick function by “useCallback” hook., which increments the ‘count’ state when called.
JavaScript
import React, { useState, useCallback } from "react";
const ExampleComponent = () => {
const [count, setCount] = useState(0);
// Define a callback function using useCallback
const handleClick = useCallback(() => {
setCount((prevCount) => prevCount + 1);
}, []);
return (
<div>
<p>Count: {count}</p>
{/* Pass the callback function to a child component */}
<ChildComponent onClick={handleClick} />
</div>
);
};
// ChildComponent receives the callback function as a prop
const ChildComponent = ({ onClick }) => {
return <button onClick={onClick}>Increment Count</button>;
};
export default ExampleComponent;
Steps to Run the App using the following command.
npm start
Output:
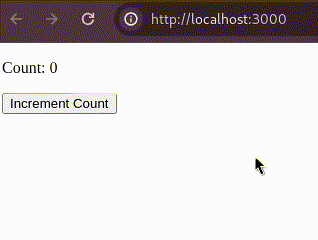
Share your thoughts in the comments
Please Login to comment...