Provide an example of using the useParams hook in React Router.
Last Updated :
28 Apr, 2024
React Router is a popular library for routing in React applications. One of its powerful features is the useParams
hook, which allows developers to access parameters from the URL in functional components. In this article, we’ll explore the useParams
hook in React Router with a practical example.
Prerequisites:
What is useParams hook?
The useParams
hook is a part of React Router’s hooks API. It provides a simple way to access URL parameters in functional components without the need for class components or prop drilling.
Approach
- Import the useParams hook from the ‘react-router-dom’ library at the beginning of your file where you’ll be using it. You’ll typically need to do this in the file where you define the component that will utilize the URL parameters.
- Within your functional component, call the useParams hook. This hook returns an object containing all the parameters from the URL.
- If your URL has named parameters (e.g., ‘/users/:id’), you can access them directly from the object returned by useParams. Use dot notation or bracket notation to access the specific parameter you need.
- Once you have accessed the parameter value, you can use it in your component logic or JSX as needed.
Steps to create the application
Step 1: Create a React application by using the following command in the terminal.
npx create-react-app myapp
Step 2: Now, go to the project folder i.e. myapp by running the following command.
cd mypp
Step 3:Â Install the necessary packages/libraries in your project using the following commands.
npm i react-router-dom
Project Structure:
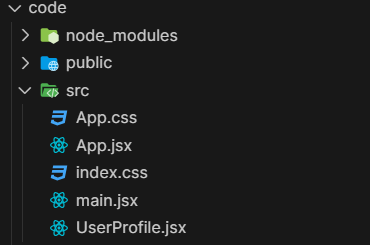
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.16.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: In this example we have a user profile page that displays userId. We’ll employ the useParams hook to extract the user’s ID from the URL and display it on the profile page.
JavaScript
// App.jsx
import React from 'react';
import {
BrowserRouter as Router,
Routes, Route,
Link
} from 'react-router-dom';
import UserProfile from './UserProfile';
const App = () => {
return (
<Router>
<div>
<nav style={styles.nav}>
<ul style={styles.navList}>
<li style={styles.navItem}>
<Link to="/users/123">
User 123
</Link>
</li>
<li style={styles.navItem}>
<Link to="/users/456">
User 456
</Link>
</li>
<li style={styles.navItem}>
<Link to="/users/789">
User 789
</Link>
</li>
</ul>
</nav>
<Routes>
<Route path="/users/:id"
element={<UserProfile />} />
</Routes>
</div>
</Router>
);
};
const styles = {
nav: {
backgroundColor: '#f4f4f4',
padding: '10px 0',
},
navList: {
listStyleType: 'none',
margin: 0,
padding: 0,
textAlign: 'center',
},
navItem: {
display: 'inline-block',
margin: '0 10px',
},
};
export default App;
JavaScript
// UserProfile.jsx
import React from 'react'
import { useParams } from 'react-router-dom'
const UserProfile = () => {
const { id } = useParams()
return (
<div style={styles.container}>
<h2>User Profile</h2>
<p>User ID: {id}</p>
</div>
)
}
const styles = {
container: {
padding: '20px',
border: '1px solid #ccc',
borderRadius: '5px',
margin: '20px auto',
width: '300px',
textAlign: 'center'
}
}
export default UserProfile
Output: In UserProfile.jsx file, This component extracts the id
parameter from the URL using the useParams
hook and displays it in the user profile page and App.jsx file is the main component where React Router is configured. It sets up navigation links
(Link
components) to different user profiles and defines a route (Route
component) that renders the UserProfile
component when the URL matches the pattern /users/:id
.
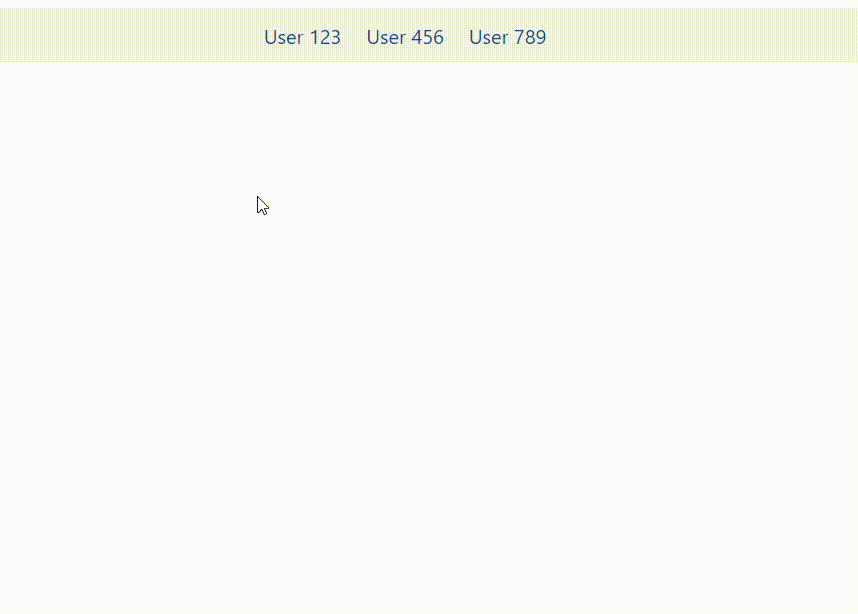
Conclusion
In this article, we explored the useParams
hook in React Router by building a simple user profile page example. We learned how to access URL parameters in functional components and use them to render dynamic content based on the URL. The useParams
hook simplifies URL parameter handling in React applications, making it easier to build dynamic and interactive web pages.
Share your thoughts in the comments
Please Login to comment...