Purpose of useReducer hook in React
Last Updated :
04 Feb, 2024
The useReducer hook is a state management hook in React that provides an alternative to the useState hook. It is used when the state of a component is complex and requires more than one state variable.
Syntax:
const [state, dispatch] = useReducer(reducer, initialState, init)
- reducer: The reducer is a function responsible for defining how the state transitions from one state to another based on dispatched actions. It takes two arguments: the current state and the action being dispatched.
- initialSate: initialState is the initial state value for the state managed by the reducer. It can be of any data type: object, array, number, string, etc., depending on the requirements of your application. It represents the initial state of your component before any actions are dispatched.
- (optional) init : init is an optional initialization function that can be used to compute the initial state lazily. It is a function that returns the initial state value. If provided, it is called once during the initial render, and its return value is used as the initial state.
Steps to Create React Application :
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: After setting up react environment on your system, we can start by creating an App.js file and create a directory by the name of components in which we will write our desired function.
Project Structure:
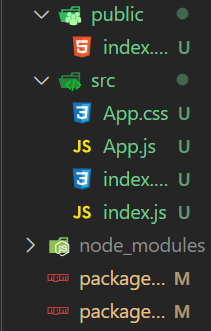
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: In this example, the reducer function takes two arguments:
- The state management involves a reducer function, where a switch statement handles different actions dispatched to it.
- The
initialState
is set to 0, serving as the starting point for the state.
- The
useReducer
hook is employed to create a state variable named count
and a corresponding dispatch function named dispatch
.
- The
count
variable is used to display the current count in the application.
- The
dispatch
function is utilized to send actions to the reducer function, triggering state updates based on the specified action.
Javascript
import React, { useReducer } from "react" ;
import "./App.css" ;
const initialState = 0;
const reducer = (state, action) => {
switch (action) {
case "add" :
return state + 1;
case "subtract" :
return state - 1;
case "reset" :
return 0;
default :
throw new Error( "Unexpected action" );
}
};
const Count = () => {
const [count, dispatch] = useReducer(reducer, initialState);
return (
<div className= "mainDiv" >
<h2>{count}</h2>
<div className= "btn" >
<button onClick={() => dispatch( "add" )}>Add</button>
<button onClick={() => dispatch( "subtract" )}>Sub</button>
<button onClick={() => dispatch( "reset" )}>Reset</button>
</div>
</div>
);
};
export default Count;
|
CSS
#root {
max-width : 1280px ;
margin : 0 auto ;
padding : 2 rem;
text-align : center ;
}
.mainDiv {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
.btn {
margin-top : 20px ;
}
button {
margin : 5px ;
}
|
Output:
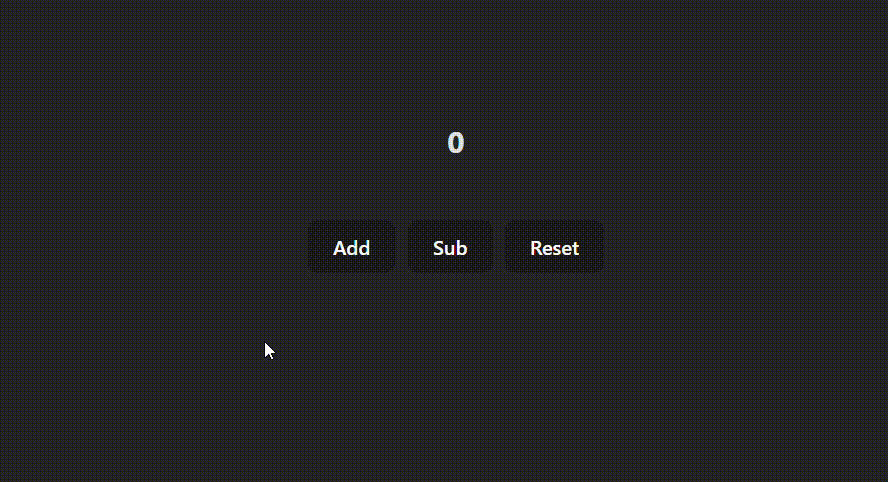
Output
Share your thoughts in the comments
Please Login to comment...