Why to use React Hooks Instead of Classes in React JS ?
Last Updated :
14 Dec, 2023
The introduction of React Hooks has changed the way we are managing states and lifecycle features. They offer more easy and functional way as compared to class based components. In this article, we will learn why to use React Hooks Instead of Classes in ReactJS, but lets first discuss about both React hooks and class based components.
What are React Hooks?
React hooks are functions added in React 16.8 that allow functional components to hold state, manage lifecycle events, and leverage other React features that were previously exclusively available in class-based components. Hooks make it easier to develop reusable and modular code in a more clear and straightforward manner.
Example:Â In this example, we’ve used the useState hook to manipulate the state.
Javascript
import React, { useState } from 'react' ;
const Counter = () => {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={incrementCount}>
Increment
</button>
</div>
);
};
export default Counter;
|
Output:
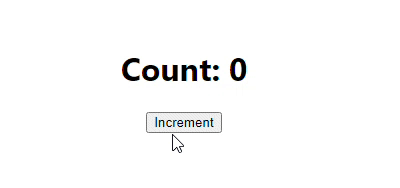
What are class-based Components in React?
Class-based components in React are an alternate technique to create reusable UI components using JavaScript classes. They are defined by extending React. Component class and implementing methods such as `render()` to define the component’s UI. Class-based components have their own state and lifecycle techniques.
Example:Â In this example, we’ve manipulated the state with the use of Class.
Javascript
import React, { Component } from 'react' ;
class Counter extends Component {
constructor(props) {
super (props);
this .state = {
count: 0,
};
}
incrementCount() {
this .setState({
count: this .state.count + 1,
});
}
render() {
return (
<div>
<h1>Count: { this .state.count}</h1>
<button onClick={() => this .incrementCount()}>
Increment
</button>
</div>
);
}
}
export default Counter;
|
Output:
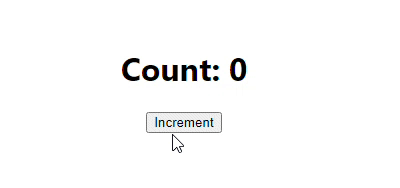
Advantages of Using Hooks over Classes:
- Readability and simplicity In comparison to class-based components, hooks offer a simpler and shorter syntax. The use of hooks enables the creation of functional components without the requirement for classes, making the code simpler to read and comprehend. Hooks produce better code by removing the hassle of handling the `this` keyword, constructor, and lifecycle functions.
- Code Reusability By allowing you to extract and reuse logic across several components, hooks improve code reuse. Without using higher-order components or render properties, you may isolate and interact with stateful behavior with custom hooks.
- More Effective Management With the use of hooks, you may more precisely group and manage related code. To handle distinct concerns individually, such as state management, side effects, and context, you can use various hooks inside of a single component.
- Preventing issues associated with classes The use of classes in React components might result in unclear circumstances regarding the binding of functions, the use of lifecycle methods, and performance improvements. These problems are resolved with hooks, which offer a simpler method of handling state and effects.
- Enhancing Performance Hooks make it easier to improve performance. Utilizing hooks like `useCallback` and `useMemo` allows you to cache functions and values, reducing the need for extra rendering iterations and enhancing component performance.
- Flexibility in the Future React has been promoting hooks as the primary method of writing components in the past few years. The hooks technique is the one that the React team recommends for creating components in React since they are investing in enhancing and increasing their capabilities. Using hooks guarantees compatibility with upcoming React releases.
Differences between React Hooks and Classes:
Syntax
|
Hooks use regular JavaScript functions
|
Classes use the class syntax with `extends React.Component` .
|
State Management
|
The useState hook is used with hooks to define and update state variables.
|
In classes, the state is defined using the `this.state` object and updated with `this.setState()`.
|
Lifecycle Methods
|
The `useEffect` hook in hooks is used to handle related lifecycle events.
|
To handle component lifecycle events, classes contain lifecycle methods such as `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount`.
|
Code Organization
|
Hooks allow you to group together relevant logic by breaking it into smaller custom hooks.
|
With classes, relevant logic and state are frequently dispersed over many lifecycle methods and class methods.
|
Reusability
|
Hooks encourage reuse by allowing you to design custom hooks that encapsulate a group of related functionalities.
|
Classes can be reused by using higher-order components (HOCs) or render properties.
|
Learning Curve
|
Hooks came into use later and offer a simpler and more logical approach to writing components.
|
React has had classes since its early versions, therefore there are more instructional materials and code samples to choose from.
|
Conclusion
React hooks make component development easier while also improving readability and organization. They allow for code reuse, improve performance, and are compatible with functional programming. Hooks provide clearer and more maintainable code by reducing class-related complications and ensuring future compatibility.
Share your thoughts in the comments
Please Login to comment...