How can you use useCallback to memoize functions in a React component?
Last Updated :
23 Feb, 2024
In ReactJS, the useCallback hook is used to memoize functions so that they are not recreated on every render unless their dependencies change. This can be useful for optimizing performance in scenarios where we are passing functions down to child components as props, especially when those functions rely on props or state values.
Prerequisites:
Steps to Create a React Application:
Step 1: Create a React application using the following command:
npx create-react-app react-app
Step 2: After creating your project folder, i.e., react-app, move to it using the following command:
cd react-app
Approach to use useCallback to memoize functions:
- We have a List component that renders a list of items.
- The Item component receives id and onDelete as props.
- Inside the Item component, we define handleDelete function using useCallback. This function calls onDelete with the current id when the delete button is clicked.
- We include id and onDelete in the dependency array to ensure that handleDelete is recreated only if they change.
- In the List component, handleDeleteItem function is defined using useCallback to remove an item from the list. It relies on the items state.
- We pass handleDeleteItem function as a prop onDelete to each Item component.
Example: Below is the code of example of Item list to delete using useCallback hook.
Javascript
import React, { useState, useCallback } from 'react' ;
const Item = ({ id, onDelete }) => {
const [itemName, setItemName] = useState(`Item ${id}`);
const handleDelete = useCallback(() => {
onDelete(id);
}, [id, onDelete]);
return (
<div>
<p>{itemName}</p>
<button onClick={handleDelete}>Delete</button>
</div>
);
};
const List = () => {
const [items, setItems] = useState([1, 2, 3, 4, 5]);
const handleDeleteItem = useCallback((id) => {
setItems(items.filter(item => item !== id));
}, [items]);
return (
<div>
{items.map(item => (
<Item key={item} id={item} onDelete={handleDeleteItem} />
))}
</div>
);
};
export default List;
|
CSS
body {
margin : 0 ;
padding : 0 ;
display : flex;
justify- content : center ;
align-items: center ;
text-align : center ;
height : 100 vh;
}
h 1 {
color : green ;
}
.main{
color : blue ;
}
.container
{
border : 2px solid blue ;
border-radius: 20px ;
background-color : rgba( 195 , 240 , 224 , 0.904 );
font-size : large ;
width : 60 vh;
height : auto ;
padding : 20px ;
}
|
Steps to Run Application:
npm start
Output: Type the following link in your browser http://localhost:3000/
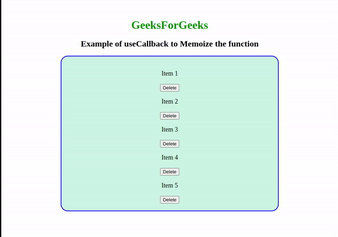
Output
Share your thoughts in the comments
Please Login to comment...