Replacement of useHistory hook in React
Last Updated :
16 Nov, 2023
In this article, we will learn how to replace the useHistory hook in React. With the introduction of new versions of React Hooks, useNavigate in React replaced the useHistory hook. useNavigate hook is a React hook that allows you to navigate your application imperatively.
What is useHistory hook?
The useHistory hook is a React Router hook that provides access to the history object that manages the browser history stack. This hook allows us to navigate between routes within the component of the React application, unlocking a world of possibilities for dynamic navigation experiences.
What is useNavigate hook?
The useNavigate hook is a React hook that allows you to navigate your application imperatively. It was introduced in React Router v6 to replace the useHistory hook, which is now deprecated.
Why useHistory hook was deprecated?
The useHistory hook was deprecated in React Router v6 in favor of the useNavigate hook. This was done for a few reasons:
- The useNavigate hook is more explicit about what it does. The useHistory hook can be used to do a lot more than just navigate, so it can be confusing for developers who are new to React Router.
- The useNavigate hook is more performant. The useHistory hook can be slow, especially in large applications. The useNavigate hook is much faster, which can improve the performance of your application.
Importing useNavigate hook:
To import the useHistory hook, write the following code at the top of the component
import { useNavigate } from "react-router-dom"
Structure of useNavigate hook:
After importing the useHistory hook, we can call it inside the functional component to get a reference to the history object.
Syntax:
const navigate = useNavigate();
The history
object returned by the useHistory
hook contains some methods that we can use to navigate between routes within the component. Here are some methods:
- navigate(): Navigates to the specified delta in the history stack. The
delta
argument is a number that represents the number of pages to go forward or backward in the history stack. A positive delta
value will go forward in the history stack, and a negative delta
value will go backward in the history stack.
Example: Below is the basic implementation of the useNavigation hook.
Javascript
import * as React from 'react' ;
import { BrowserRouter as Router, Route, Routes }
from 'react-router-dom' ;
import Home from './Component/TempConvert' ;
import About from './Component/newcom' ;
const App = () => (
<Router>
<Routes>
<Route exact path= "/" element={<Home />} />
<Route path= "/about" element={<About />} />
</Routes>
</Router>
);
export default App;
|
Javascript
import * as React from 'react' ;
import { useNavigate } from 'react-router-dom' ;
import '../App.css'
const Home = () => {
const navigate = useNavigate();
const handleClick = () => navigate( '/about' );
return (
<>
<h1>
Home Page
</h1>
<button type= "button" onClick={handleClick}>
About Page
</button>
</>
);
};
export default Home;
|
Javascript
import * as React from 'react' ;
import { useNavigate } from 'react-router-dom' ;
const About = () => {
const navigate = useNavigate();
const handleClick = () => navigate( '/' );
return (
<div>
<h1>About Page</h1>
<button type= "button" onClick={handleClick}>
Home Page
</button>
</div>
)
}
export default About;
|
Output:
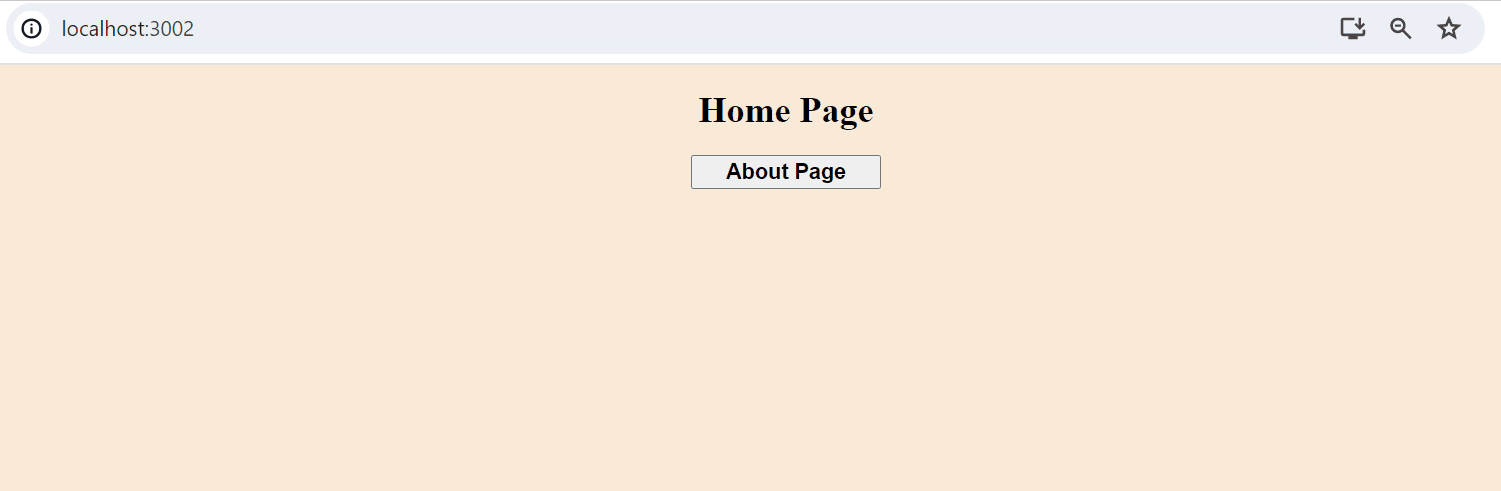
Output
Share your thoughts in the comments
Please Login to comment...