AngularJS | Forms
Last Updated :
31 May, 2019
Forms are collection of controls that is input field, buttons, checkbox and these can be validated
real time. As soon as a user of the form completes writing a field and moves to the next one it
gets validated and suggests the user where he might have went wrong.
So a form can be consisting of the many number of controls
However we will be focusing on
- Input field
- Checkbox
- Radiobox
- Button
- SelectBox(Dropdowns)
1) Input fields:
Syntax:
< input type = "text" value = "name" ng-model = "name" placeholder = "name" >
|
Various constraints can be added to make the necessary validations, also we can use place-
holders to predefine the input box for the users convenience so that if the form has an
error than the user does not have to put the right details all over again.
In app.module.ts
import { FormsModule } from '@angular/forms';
in the imports list at the beginning and also add the Formsmodule in the following:
imports: [
BrowserModule,
FormsModule,
],
Include the above code always while creating forms.
Example #1:
In app.component.html
< form >
< div class = "form-group" >
< label for = "firstName" >Name</ label >
< input type = "text"
id = "firstName"
placeholder = "Name" >
</ div >
< div class = "form-group" >
< label for = "email" >Email</ label >
< input
type = "text"
id = "email"
placeholder = "Email" >
</ div >
< div class = "form-group" >
< label for = "password" >Password</ label >
< input
type = "password"
id = "password"
placeholder = "Password" >
</ div >
< div class = "form-group" >
< label for = "phone" >mobile</ label >
< input
type = "text"
id = "phone"
ngModel name = "phone"
# phone = "ngModel"
placeholder = "Mobile" >
</ div >
</ form >
< p >{{ phone.value }}</ p >
|
To use the values written in the input box we store it in a variable by two way binding.
Output:
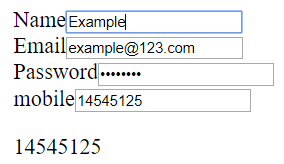
Checkbox and Select(Dropdowns):
In a form, the variable defined in ngModel is stored with true on getting selected otherwise false.
In Select, the selected value gets stored in the variable defined in the ngModel.
Example #2:
In app.component.html
< form >
< input id = "myVar" type = "checkbox" ngModel name = "myVar" # myVar = "ngModel" >
< p >The checkbox is selected: {{myVar.value}}</ p >
< br />
< select ngModel name = "mychoice" # myChoice = "ngModel" >
< option >A</ option >
< option >E</ option >
< option >I</ option >
< option >O</ option >
< option >U</ option >
</ select >
< p >The selected option from Dropdown {{ myChoice.value }}</ p >
</ form >
|
Output
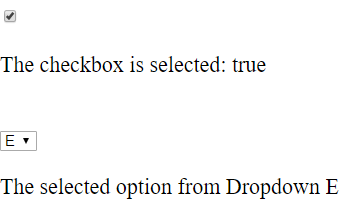
Radio Buttons and Buttons
Radio buttons used in the forms should allow only one field to be selected at a time to make sure this is the
case we should associate it with only ngModel.
Example #3(Radio Button):
In app.component.html
< form >
< p >Select a radio button to know which Vowel it is associated to:</ p >
< input value = "A" type = "radio" ngModel name = "myVar" # myVar = "ngModel" >
< input value = "E" type = "radio" ngModel name = "myVar" # myVar = "ngModel" >
< input value = "I" type = "radio" ngModel name = "myVar" # myVar = "ngModel" >
< input value = "O" type = "radio" ngModel name = "myVar" # myVar = "ngModel" >
< input value = "U" type = "radio" ngModel name = "myVar" # myVar = "ngModel" >
< br />< button * ngIf = 'myVar.touched' >Submit</ button >
</ form >
< p >You have selected: {{myVar.value}}</ p >
|
In this code, the button will be visible only after selecting one of the radio buttons. However it won’t be visible as soon as you select it since when you on its selection the field touched(being selected atleast once) turns to
true and however it is not getting reflected in the buttons if condition as it is preloaded.
So this button will only be visible once we click anywhere on the screen or we change our selection.
Before Selecting:
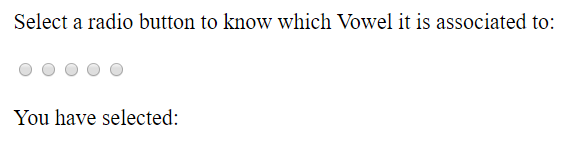
After Selecting:
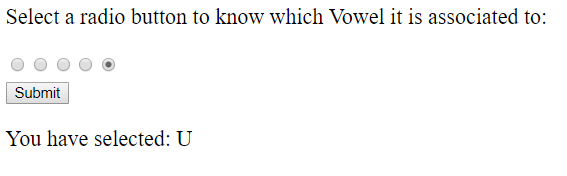
We can add validations to all these input types and make our forms responsive.
Share your thoughts in the comments
Please Login to comment...