Top Sorting Interview Questions and Problems
Last Updated :
21 Mar, 2024
Here is the collection of the Top 50 list of frequently asked interview questions on Sorting. Problems in this article are divided into three Levels so that readers can practice according to the difficulty level step by step.
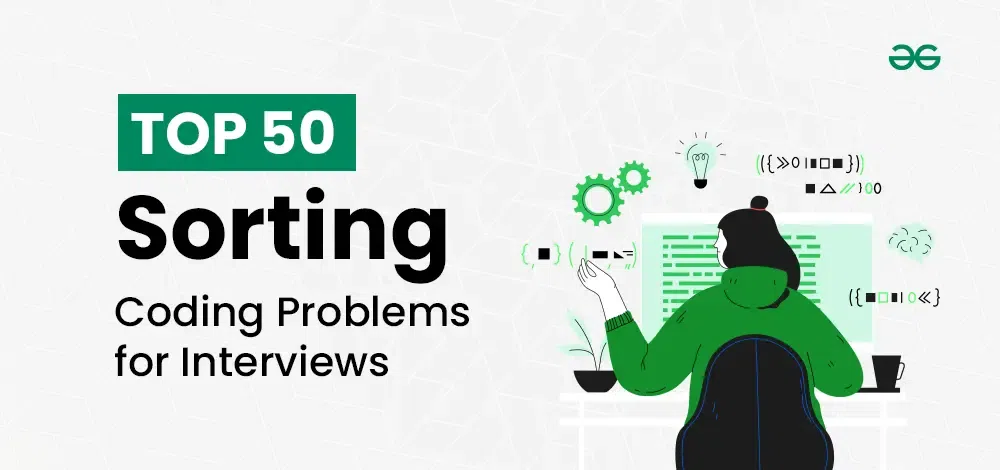
Top 50 Sorting Coding Problems for Interviews
Sorting Interview Question and Answers:
Question 1: What is a sorting algorithm?
Answer: A sorting algorithm is a method used to arrange elements in a specific order, often from smallest to largest or vice versa, making data easier to manage and search.
Question 2: What are the different types of sorting algorithms?
Answer: There are two types of Sorting algorithms: Comparison based sorting algorithms and non-comparison-based sorting algorithms. Comparison based sorting algorithms include Bubble Sort, Selection Sort, Insertion Sort, Merge Sort, Quick Sort, Heap Sort, etc. and non-comparison-based sorting algorithms include Radix Sort, Counting Sort and Bucket Sort.
Question 3: Why Sorting algorithms are important?
Answer: The effectiveness of other algorithms (like search and merge algorithms) that depend on input data being in sorted lists is enhanced by efficient sorting. Sorting is also frequently helpful for generating output that is readable by humans. Sorting is directly used in divide-and-conquer strategies, database algorithms, data structure algorithms, and many other applications.
Question 4: What is the difference between comparison-based and non-comparison-based sorting algorithms?
Answer: Comparison-based sorting algorithms compare elements to determine their order, while non-comparison-based algorithms use other techniques, like counting or bucketing, to sort elements without direct comparisons.
Question 5: Explain what is ideal Sorting Algorithm?
Answer: The Ideal Sorting Algorithm would have the following properties:
- Stable: Equal keys are not reordered.
- Operates in place: Requires O(1) extra space.
- Worst-case O(n log n) key comparisons: Guaranteed to perform no more than O(n log n) key comparisons in the worst case.
- Adaptive: Speeds up to O(n) when the data is nearly sorted or when there are few unique keys.
The choice of sorting algorithm depends on the specific requirements of the application. Some algorithms prioritize stability, while others prioritize speed or space efficiency.
Question 6: What is meant by “Sort in Place”?
Answer: In-place algorithms prioritize space efficiency by utilizing the same memory space for both input and output. This eliminates the need for additional storage, thereby reducing memory requirements. Selection Sort, Bubble Sort, Insertion Sort, Heap Sort and Quicksort are in-place sorting algorithms.
Question 7: Which sort algorithm works best on mostly sorted data?
Answer: For mostly sorted data, Insertion Sort typically works best. It’s efficient when elements are mostly in order because it only needs to make small adjustments to place each element in its correct position, making it faster than other sorting algorithms like Quick Sort or Merge Sort.
Question 8: Why is Merge sort preferred over Quick Sort for sorting linked lists?
Answer: Merge Sort is preferred for sorting linked lists because its divide-and-conquer approach easily divides the list into halves and merges them efficiently without requiring random access, which is difficult in linked lists. Quick Sort’s reliance on random access and potential worst-case time complexity makes it less suitable for linked lists.
Question 9: What is Stability in sorting algorithm and why it is important?
Answer: Stability in sorting algorithms means that the relative order of equal elements remains unchanged after sorting. Stable sorting algorithms ensure that equal elements maintain their original positions in the sorted sequence. Some of the stable sorting algorithms are: Bubble Sort, Insertion Sort, Merge Sort and Counting Sort.
Question 10: What is the best sorting algorithm for large datasets?
Answer: For large datasets, efficient sorting algorithms like Merge Sort, Quick Sort, or Heap Sort are commonly used due to their average time complexity of O(n log n), which performs well even with large amounts of data.
Question 11: How does Quick Sort work?
Answer: Quick Sort is a Divide and Conquer sorting algorithm. It chooses a pivot element and rearrange the array so that elements smaller than the pivot are on the left, and elements greater are on the right. Then, recursively apply the partitioning process to the left and right subarrays. Subarrays of size one or zero are considered sorted.
Question 12: What is the worst-case time complexity of Quick Sort?
Answer: In the worst case, Quick Sort may take O(N^2) time to sort the array. The worst case will occur when everytime the problem of size N, gets divided into 2 subproblems of size 1 and N – 1.
Sorting Interview Problems:
Easy Problems on Sorting:
Medium Problems on Sorting:
Hard Problems on Sorting:
Related Articles:
Share your thoughts in the comments
Please Login to comment...