How to write a Pseudo Code?
Last Updated :
23 Nov, 2023
Pseudo code is a term which is often used in programming and algorithm based fields. It is a methodology that allows the programmer to represent the implementation of an algorithm. Simply, we can say that it’s the cooked up representation of an algorithm. Often at times, algorithms are represented with the help of pseudo codes as they can be interpreted by programmers no matter what their programming background or knowledge is. Pseudo code, as the name suggests, is a false code or a representation of code which can be understood by even a layman with some school level programming knowledge. Algorithm: It’s an organized logical sequence of the actions or the approach towards a particular problem. A programmer implements an algorithm to solve a problem. Algorithms are expressed using natural verbal but somewhat technical annotations. Pseudo code: It’s simply an implementation of an algorithm in the form of annotations and informative text written in plain English. It has no syntax like any of the programming language and thus can’t be compiled or interpreted by the computer.
Advantages of Pseudocode
- Improves the readability of any approach. It’s one of the best approaches to start implementation of an algorithm.
- Acts as a bridge between the program and the algorithm or flowchart. Also works as a rough documentation, so the program of one developer can be understood easily when a pseudo code is written out. In industries, the approach of documentation is essential. And that’s where a pseudo-code proves vital.
- The main goal of a pseudo code is to explain what exactly each line of a program should do, hence making the code construction phase easier for the programmer.
How to write a Pseudo-code?
- Arrange the sequence of tasks and write the pseudocode accordingly.
- Start with the statement of a pseudo code which establishes the main goal or the aim. Example:
This program will allow the user to check
the number whether it's even or odd.
- The way the if-else, for, while loops are indented in a program, indent the statements likewise, as it helps to comprehend the decision control and execution mechanism. They also improve the readability to a great extent.
Example:
if "1"
print response
"I am case 1"
if "2"
print response
"I am case 2"
- Use appropriate naming conventions. The human tendency follows the approach to follow what we see. If a programmer goes through a pseudo code, his approach will be the same as per it, so the naming must be simple and distinct.
- Use appropriate sentence casings, such as CamelCase for methods, upper case for constants and lower case for variables.
- Elaborate everything which is going to happen in the actual code. Don’t make the pseudo code abstract.
- Use standard programming structures such as ‘if-then’, ‘for’, ‘while’, ‘cases’ the way we use it in programming.
- Check whether all the sections of a pseudo code is complete, finite and clear to understand and comprehend.
- Don’t write the pseudo code in a complete programmatic manner. It is necessary to be simple to understand even for a layman or client, hence don’t incorporate too many technical terms.
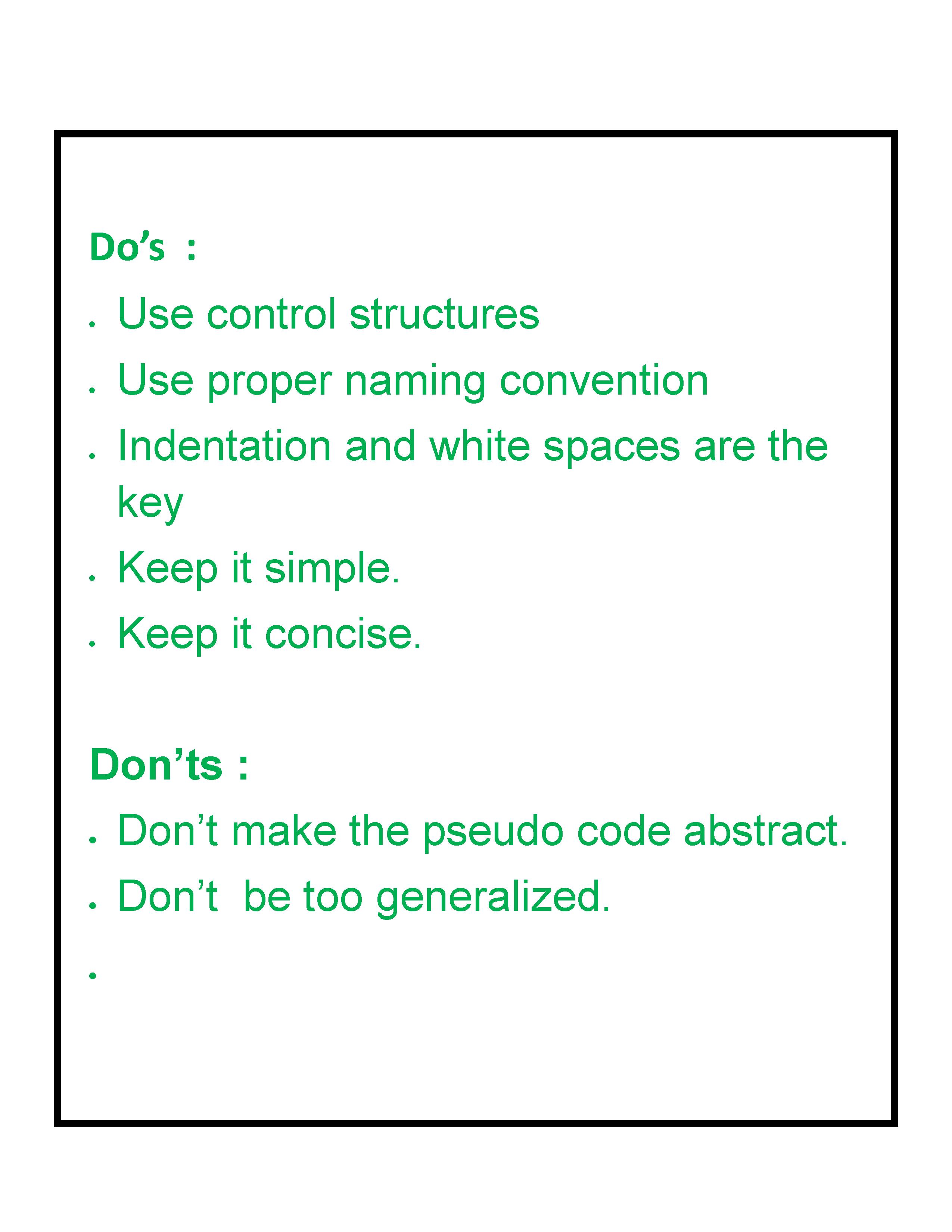
Dos and Don’ts to Pseudo Code Writing
Example:
Let’s have a look at this code
C++
#include <iostream>
long long gcd( long long numberOne, long long numberTwo) {
if (numberTwo == 0)
return numberOne;
return gcd(numberTwo, numberOne % numberTwo);
}
long long lcmNaive( long long numberOne, long long numberTwo) {
long long lowestCommonMultiple = (numberOne * numberTwo) / gcd(numberOne, numberTwo);
return lowestCommonMultiple;
}
int main() {
std::cout << "Enter the inputs" << std::endl;
long long numberOne, numberTwo;
std::cin >> numberOne >> numberTwo;
std::cout << lcmNaive(numberOne, numberTwo) << std::endl;
return 0;
}
|
Java
import java.util.*;
public class LowestCommonMultiple {
private static long
lcmNaive( long numberOne, long numberTwo)
{
long lowestCommonMultiple;
lowestCommonMultiple
= (numberOne * numberTwo)
/ greatestCommonDivisor(numberOne,
numberTwo);
return lowestCommonMultiple;
}
private static long
greatestCommonDivisor( long numberOne, long numberTwo)
{
if (numberTwo == 0 )
return numberOne;
return greatestCommonDivisor(numberTwo,
numberOne % numberTwo);
}
public static void main(String args[])
{
Scanner scanner = new Scanner(System.in);
System.out.println( "Enter the inputs" );
long numberOne = scanner.nextInt();
long numberTwo = scanner.nextInt();
System.out.println(lcmNaive(numberOne, numberTwo));
}
}
|
Python
def gcd(numberOne, numberTwo):
if numberTwo = = 0 :
return numberOne
return gcd(numberTwo, numberOne % numberTwo)
def lcmNaive(numberOne, numberTwo):
lowestCommonMutliple = (numberOne * numberTwo) / gcd(numberOne, numberTwo)
return lowestCommonMutliple
numberOne = 5
numberTwo = 2
print (lcmNaive(numberOne, numberTwo))
|
C#
using System;
public class LowestCommonMultiple {
private static long
lcmNaive( long numberOne, long numberTwo)
{
long lowestCommonMultiple;
lowestCommonMultiple
= (numberOne * numberTwo)
/ greatestCommonDivisor(numberOne,
numberTwo);
return lowestCommonMultiple;
}
private static long greatestCommonDivisor( long numberOne, long numberTwo)
{
if (numberTwo == 0)
return numberOne;
return greatestCommonDivisor(numberTwo,numberOne % numberTwo);
}
public static void Main()
{
Console.WriteLine( "Enter the inputs" );
long numberOne = Convert.ToInt64(Console.ReadLine());
long numberTwo = Convert.ToInt64(Console.ReadLine());
Console.WriteLine(lcmNaive(numberOne, numberTwo));
}
}
|
Javascript
function gcd(numberOne, numberTwo) {
if (numberTwo === 0) {
return numberOne;
}
return gcd(numberTwo, numberOne % numberTwo);
}
function lcmNaive(numberOne, numberTwo) {
return (numberOne * numberTwo) / gcd(numberOne, numberTwo);
}
const numberOne = 5;
const numberTwo = 2;
console.log( 'Lowest Common Multiple:' , lcmNaive(numberOne, numberTwo));
|
Share your thoughts in the comments
Please Login to comment...