Spring – REST JSON Response
Last Updated :
03 Mar, 2022
REST APIs are becoming popular for the advantages they provide in the development of applications. REST APIs work like a client-server architecture. The client makes a request and a server (REST API) responds back by providing some kind of data. A client can be any front-end framework like Angular, React, etc, or Spring application ( internal/external ) itself. Data can be sent in various formats like plain text, XML, JSON, etc. Of these formats, JSON ( JavaScript Object Notation ) is a standard for transporting data between web applications.
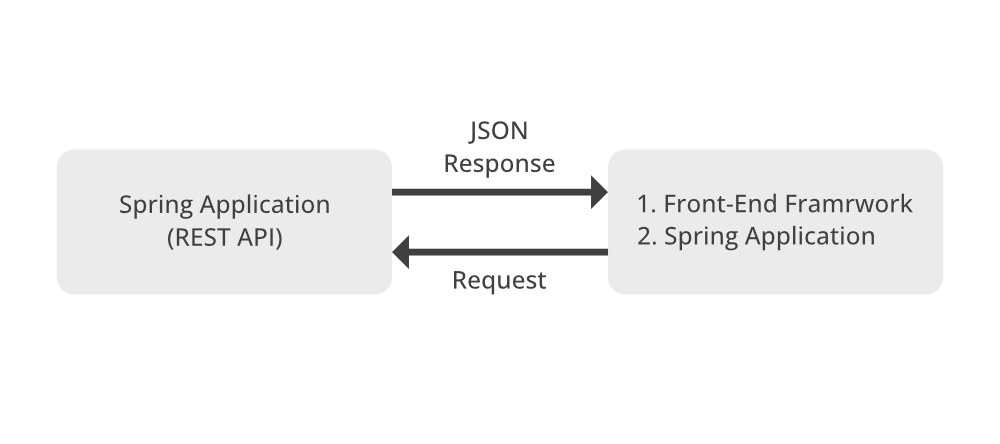
Pre-requisites required are as follows:
- JSON string can be stored in it own file with the ‘.json‘ extension.
- It has the MIME type of – ‘application/json‘.
JSON
JSON is an abbreviation for JavaScript Object Notation. It is a text-based data format following Javascript object syntax. It has syntax somewhat like a Javascript object literal and can be used independently from Javascript. Many programming environments have the ability to parse and generate JSON. It exists as a string and needs to be converted into a native Javascript object to access the data by available global JSON methods of Javascript.
Structure of the JSON
{
"id" : 07,
"framework" : "Spring",
"API" : "REST API",
"Response JSON" : true
}
On sending an array of objects, they are wrapped in the square brackets
[
{
"id" : 07,
"name" : "darshan"
},
{
"id" : 08,
"name" : "DARSHAN"
}
]
- Spring framework’s ‘Starter Web’ dependency provides you with the two essential features of Spring MVC – (Spring’s web framework) and the RESTful ( REST API ) methodology.
- To include ‘Starter Web’ in the Spring application, add the following dependency –
Maven -> pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
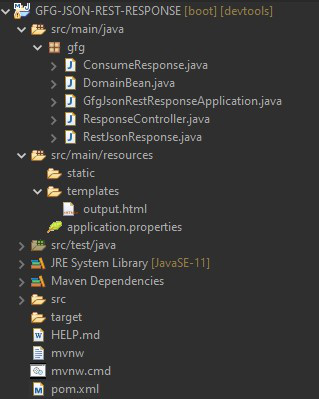
Project structure – Maven
File: pom.xml (Configurations)
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< parent >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-parent</ artifactId >
< version >2.6.3</ version >
< relativePath />
</ parent >
< groupId >sia</ groupId >
< artifactId >GFG-JSON-REST-RESPONSE</ artifactId >
< version >0.0.1-SNAPSHOT</ version >
< name >GFG-JSON-REST-RESPONSE</ name >
< description >REST API Response</ description >
< properties >
< java.version >11</ java.version >
</ properties >
< dependencies >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-thymeleaf</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-web</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-test</ artifactId >
< scope >test</ scope >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter</ artifactId >
</ dependency >
< dependency >
< groupId >org.projectlombok</ groupId >
< artifactId >lombok</ artifactId >
< optional >true</ optional >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-devtools</ artifactId >
< scope >runtime</ scope >
< optional >true</ optional >
</ dependency >
</ dependencies >
< build >
< plugins >
< plugin >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-maven-plugin</ artifactId >
< configuration >
< excludes >
< exclude >
< groupId >org.projectlombok</ groupId >
< artifactId >lombok</ artifactId >
</ exclude >
</ excludes >
</ configuration >
</ plugin >
</ plugins >
</ build >
</ project >
|
File: GfgJsonRestResponseApplication.java ( Bootstrapping of the application )
Java
package gfg;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GfgJsonRestResponseApplication {
public static void main(String[] args)
{
SpringApplication.run(
GfgJsonRestResponseApplication. class , args);
}
}
|
File: DomainBean.java ( User properties – The data to be transferred )
- Java Beans requires the Getter/Setter methods.
- One can generate them automatically with the ‘@Data’ annotation of the ‘Lombok’ library.
- Dependency is as depicted below as follows:
Maven -> pom.xml
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
Java
package gfg;
import lombok.Data;
@Data
public class DomainBean {
String id;
String name;
String data;
}
|
File: RestJsonResponse.java ( REST API )
- This class provides RESTful services.
- Essential annotations are –
Annotation
|
Description
|
@RestController |
Combines @Controller and @ResponseBody |
@RequestMapping |
Maps web requests with ‘path’ attribute and response format with ‘produces’ attribute |
@CrossOrigin |
Permits cross-origin web requests on the specific handler classes/methods |
@GetMapping |
Maps GET request on specific handler method |
@PathVariable |
Binds the URI template variables to method parameters |
Java
package gfg;
import java.util.ArrayList;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping (path= "/JSON" , produces= "application/json" )
@CrossOrigin (origins= "*" )
public class RestJsonResponse {
@GetMapping ( "/data" )
public ArrayList<DomainBean> get() {
ArrayList<DomainBean> arr = new ArrayList<>();
DomainBean userOne = new DomainBean();
userOne.setId( "1" );
userOne.setName( "@geek" );
userOne.setData( "GeeksforGeeks" );
DomainBean userTwo = new DomainBean();
userTwo.setId( "2" );
userTwo.setName( "@drash" );
userTwo.setData( "Darshan.G.Pawar" );
arr.add(userOne);
arr.add(userTwo);
return arr;
}
@GetMapping ( "/{id}/{name}/{data}" )
public ResponseEntity<DomainBean> getData( @PathVariable ( "id" ) String id,
@PathVariable ( "name" ) String name,
@PathVariable ( "data" ) String data) {
DomainBean user = new DomainBean();
user.setId(id);
user.setName(name);
user.setData(data);
HttpHeaders headers = new HttpHeaders();
ResponseEntity<DomainBean> entity = new ResponseEntity<>(user,headers,HttpStatus.CREATED);
return entity;
}
}
|
Outputs:
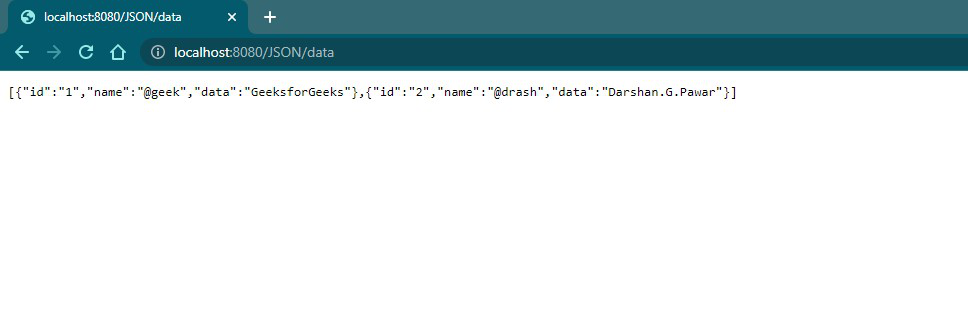
An array of JSON object literals
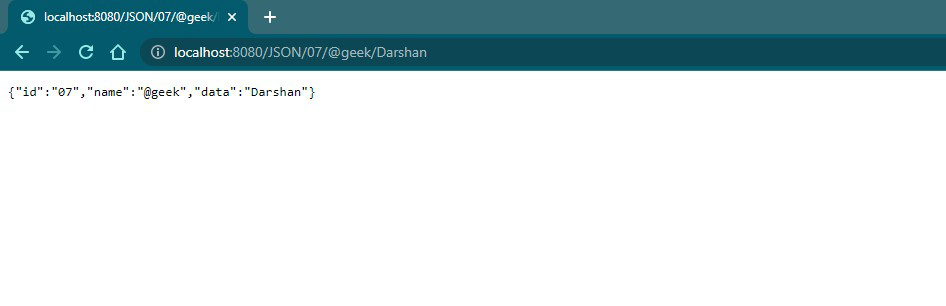
JSON object literal
REST API’s JSON response can be consumed by:
- Spring application itself.
- Front-end application/framework
A: Spring Application
- Spring offers the ‘RestTemplate‘ – a convenient way of handling a REST response.
- It has the HTTP method-specific handler methods.
File: ConsumeResponse.java ( Consume REST API response )
Java
package gfg;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class ConsumeResponse {
RestTemplate rest = new RestTemplate();
public ResponseEntity<DomainBean> get()
{
return rest.getForEntity(
DomainBean. class , "007" , "geek@drash" ,
"Darshan.G.Pawar" );
}
}
|
File: ResponseController.java ( Regular Controller )
A normal Spring controller is used to retrieve the responses from the ‘RestTemplate’ method and returns a view.
Example:
Java
package gfg;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping ( "/ConsumeResponse" )
public class ResponseController {
@GetMapping ( "/get" ) public String get(Model model)
{
ConsumeResponse data = new ConsumeResponse();
model.addAttribute( "response" ,
data.get().getBody());
model.addAttribute( "headers" ,
data.get().getHeaders());
return "output" ;
}
}
|
File: Output.html ( output of API: Thymeleaf template)
HTML
<!DOCTYPE html>
< head >
< title >GeeksforGeeks</ title >
</ head >
< body >
< h1 style = "color:forestgreen" th:text = "${response.id}" >attributeValue will be placed here</ h1 >
< h1 style = "color:forestgreen" th:text = "${response.name}" >attributeValue will be placed here</ h1 >
< h1 style = "color:forestgreen" th:text = "${response.data}" >attributeValue will be placed here</ h1 >
< h2 style = "color:forestgreen; width : 350px" th:text = "${headers}" >attributeValue will be placed here</ h2 >
</ body >
</ html >
|
Output:
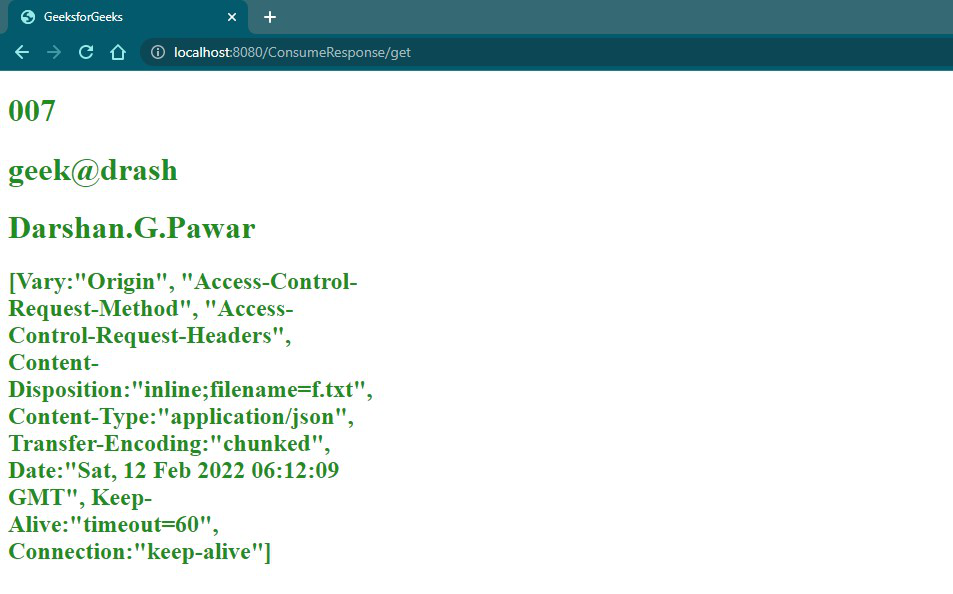
Response with JSON as content type
B: Front-end Application/Framework – Angular
File: consume-json.component.ts ( Angular component )
- This component retrieves the JSON data from the specified URL targeting REST API.
- Retrieved data is stored in a variable.
import { Component, OnInit, Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-consume-json',
templateUrl: './consume-json.component.html',
styleUrls: ['./consume-json.component.css']
})
export class ConsumeJsonComponent implements OnInit {
restData : any;
constructor(private http:HttpClient) { }
ngOnInit() {
this.http.get('http://localhost:8080/JSON/data')
.subscribe(data => this.restData = data);
}
}
File: consume-json.component.html ( view of component)
- Get the stored data by dot( . ) notation.
- ‘ngFor’ is an Angular template directive used for iterating through the collection of objects.
HTML
< h1 >Rest API Response : JSON</ h1 >
< div >
< table * ngFor = "let json of restData" >
< tr >
< td >{{json.id}}</ td >
< td >{{json.name}}</ td >
< td >{{json.data}}</ td >
</ tr >
</ table >
</ div >
|
File: consume-json.component.css ( Style file )
CSS
h 1 , tr{
color : green ;
font-size : 25px ;
}
|
Add - '<app-consume-json></app-consume-json>' in the 'app.component.html' file
Output:
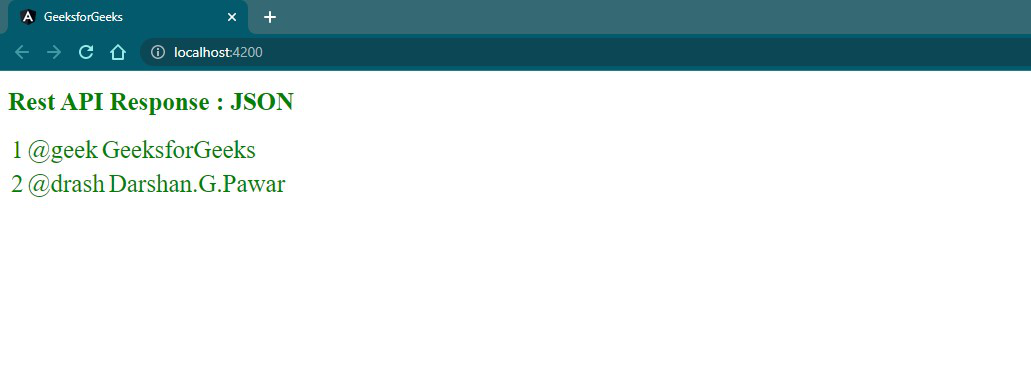
Response retrieved by Angular component
- You can additionally map an object to JSON object literal using the Jackson API.
- Add the following dependency:
Maven -> pom.xml
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.5.3</version>
</dependency>
Note: This dependency will also automatically add the following libraries to the classpath:
- jackson-annotations
- jackson-core
Share your thoughts in the comments
Please Login to comment...