Spring – Remoting By Hessian
Last Updated :
24 Mar, 2022
We may use the HessianServiceExporter and HessianProxyFactoryBean classes to implement the hessian remoting service. The major advantage of Hessian’s is that Hessian works well on both sides of a firewall. Hessian is a portable language that may be used with other languages like PHP and.Net.
Implementation: To make a simple hessian application, you’ll need to make the following files:
- Calculation.java
- CalculationImpl.java
- web.xml
- hessian-servlet.xml
- client-beans.xml
- Client.java
The project structure looks as follows:
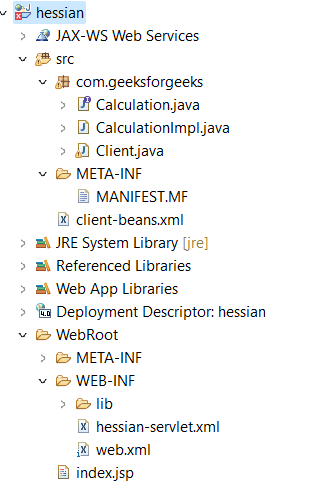
A. File: Calculation.java
Java
package com.geeksforgeeks;
public interface Calculation {
int cube( int number);
}
|
B. File: CalculationImpl.java
Java
package com.geeksforgeeks;
public class CalculationImpl implements Calculation {
public int cube( int number)
{
return number * number * number;
}
}
|
C. File: web.xml
The front controller is defined in this XML file called DispatcherServlet. Any request that ends in.http will be routed to DispatcherServlet.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< web-app >
< servlet >
< servlet-name >hessian</ servlet-name >
< servlet-class >org.springframework.web.servlet.DispatcherServlet</ servlet-class >
< load-on-startup >1</ load-on-startup >
</ servlet >
< servlet-mapping >
< servlet-name >hessian</ servlet-name >
< url-pattern >*.http</ url-pattern >
</ servlet-mapping >
</ web-app >
|
D. File: hessian-servlet.xml
It has to be placed in the WEB-INF folder. It must be called servletname-servlet.xml. It specifies CalculationImpl and HessianServiceExporter as beans.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< bean id = "calculationBean" class = "com.geeksforgeeks.CalculationImpl" ></ bean >
< bean name = "/Calculation.http" class = "org.springframework.remoting.caucho.HessianServiceExporter" >
< property name = "service" ref = "calculationBean" ></ property >
< property name = "serviceInterface" value = "com.geeksforgeeks.Calculation" ></ property >
</ bean >
</ beans >
|
E. File: client-beans.xml
The bean for HessianProxyFactoryBean is defined in this XML file. This class requires two attributes to be defined.
- serviceUrl
- serviceInterface
F. File: Client.java
This class obtains a Calculation object and runs the cube function.
Java
package com.geeksforgeeks;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Client
{
public static void main(String[] args)
{
ApplicationContext context = new ClassPathXmlApplicationContext( "client-beans.xml" );
Calculation calculation = (Calculation)context.getBean( "calculationBean" );
System.out.println(calculation.cube( 4 ));
}
}
|
Output:
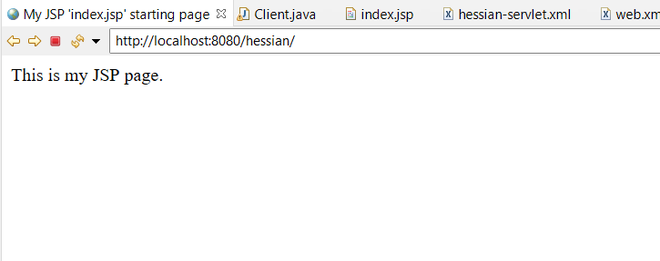
Note: In order to generate the above output,
- Start and deploy the project, assuming that the server is listening on port 8888. Change the serviceURL in client-beans.xml if the port number is changed.
- The Client.java file should then be compiled and run.
Share your thoughts in the comments
Please Login to comment...