Selenium Webdriver Handling Checkbox using Java
Last Updated :
28 Feb, 2024
A set of tools and libraries that allow the user to automate interactions with web browsers is known as Selenium. It is too diverse tool that can handle every operation of a webpage starting from opening the webpage to closing of webpage. In this article, we will see the various steps to handle the checkboxes using Selenium webdriver in Java.
Steps of Selenium Webdriver Handling Checkbox Using Java
Certain steps need to be followed to handle the checkbox using Selenium webdriver in Java.
Setting up the Selenium in Java
For setting up Selenium in Java, you need to download the Selenium JARs from the official website of Selenium. Add those JARs under the Add external JARs available under the Configure Build Path section.
Installing the Necessary Modules
Now, the crucial modules that are needed for scrolling a webpage in Java are WebElement, WebDriver, By, and ChromeDriver. We import all these modules now.
- WebDriver: An interface available in Selenium that is used to interact with webpages is called WebDriver.
- WebElement: A function that represents a way to interact with and manipulate the attributes and properties of the elements is known as WebElement.
- ChromeDriver: A module that establishes a connection between the Selenium web driver and Chrome browser is called ChromeDriver.
- By: The mechanism to find elements on the webpage currently opened is known as By.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
Initiating the Web Driver and Navigate to the Webpage
Further, we will define the location of ChromeDriver inside the setProperty function and initiate the ChromeDriver. Here, we have opened the Geeks for Geeks website (link) using the get() method.
get()
The get() method navigates the user to a certain webpage stated by him by opening that webpage in the respective browser.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium4 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
}
}
|
Finding an Element
Once the website is loaded, we will now find an element, i.e., checkboxes, that needs to be handled using the findElement, By.xpath and contains function.
- findElement: The function used to find the first occurrence of the element in the webpage is known as the findElement function.
- By.xpath: The function that is used to locate elements by the relative path is known as the By.xpath function.
- contains(): The way of finding an element based on a partial text in the webpage is known as the contains function.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium4 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.findElement(By.xpath( "/html/body/div[1]/div/div[3]/div[2]/div/div[2]/div/div/div/button" )).click();
WebElement check_box1 = driver.findElement(By.xpath( "// *[contains(text(),'Amazon')]" ));
WebElement check_box2 = driver.findElement(By.xpath( "// *[contains(text(),'Flipkart')]" ));
}
}
|
Output:
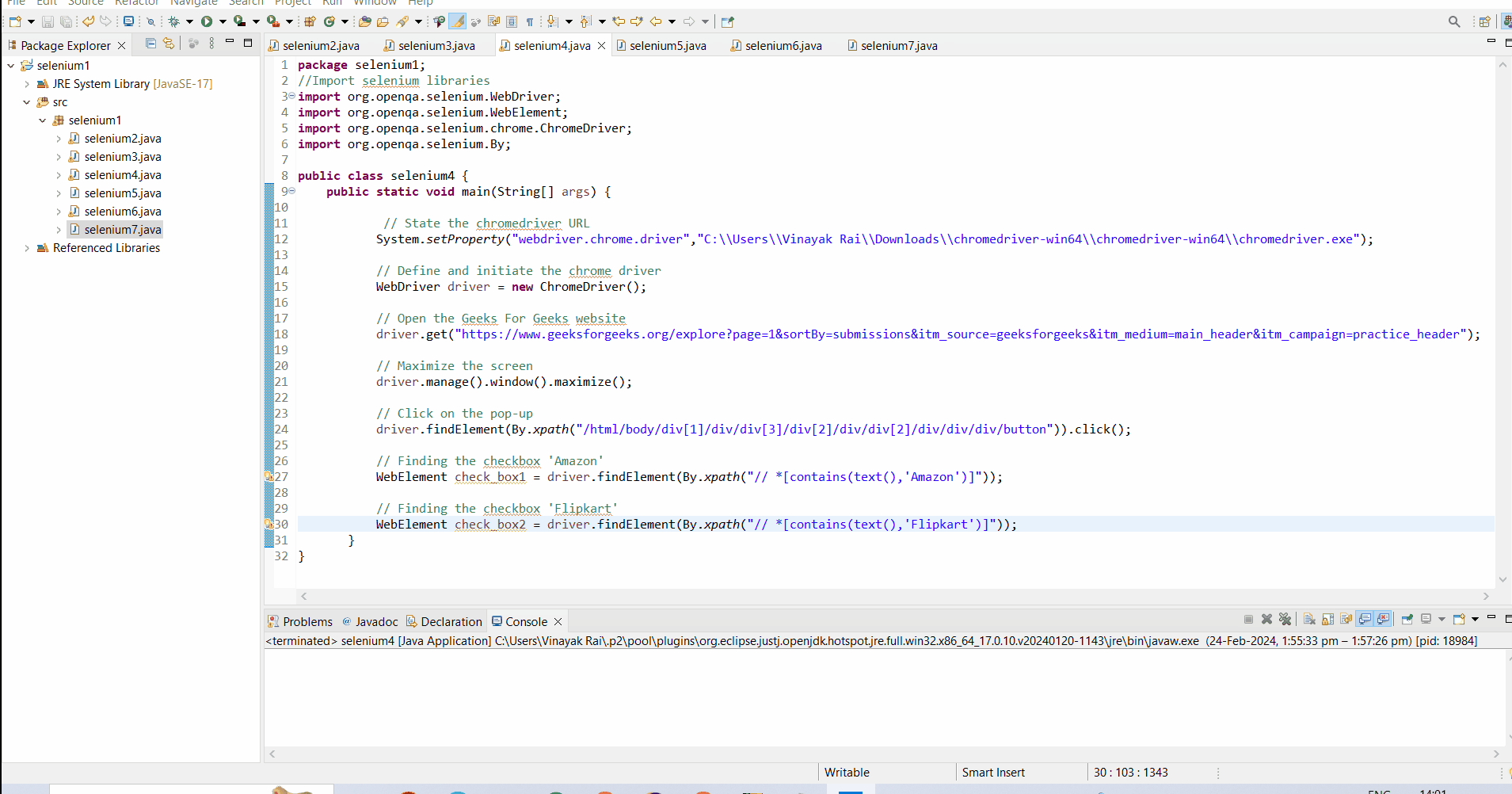
Click on an Element
Finally, we will click on the checkboxes check_box1 and check_box2, that we found in the previous step using the click function.
click()
The function that is used to interact with clickable objects, such as buttons, checkboxes, etc. is known as the click function. It is always executed in the center of the element.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium4 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.findElement(By.xpath( "/html/body/div[1]/div/div[3]/div[2]/div/div[2]/div/div/div/button" )).click();
WebElement check_box1 = driver.findElement(By.xpath( "// *[contains(text(),'Amazon')]" ));
WebElement check_box2 = driver.findElement(By.xpath( "// *[contains(text(),'Flipkart')]" ));
check_box1.click();
check_box2.click();
}
}
|
Output:
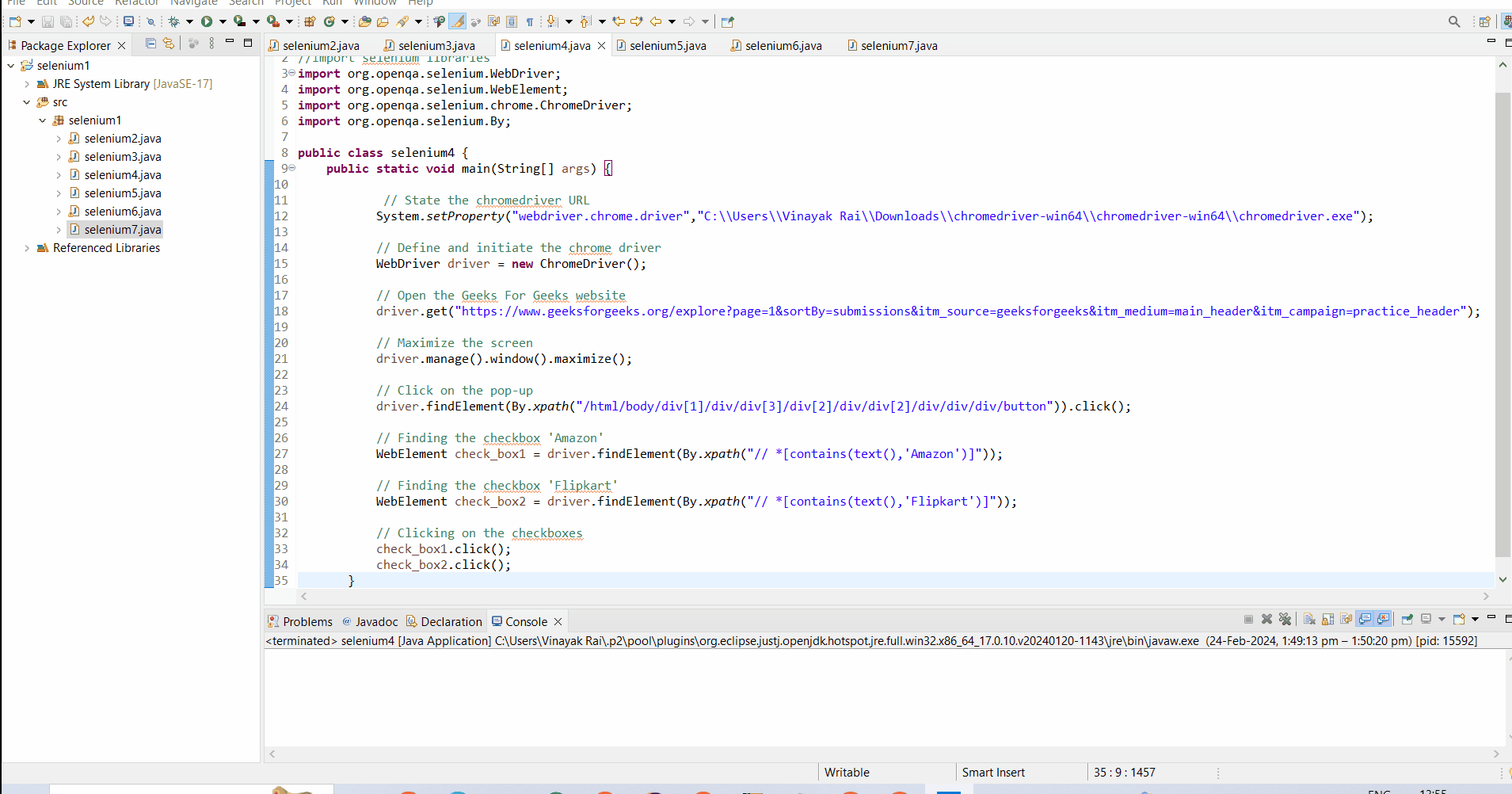
Check if the Element is Selected
This is an additional step, in case you want to check if the checkbox is selected or not and then perform the certain action. An element that is checked or not can be tested using the isSelected function.
isSelected()
The function that is used to check if a checkbox or radio button element is selected or not is known as the isSelected function. It returns a boolean value.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium4 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.findElement(By.xpath( "/html/body/div[1]/div/div[3]/div[2]/div/div[2]/div/div/div/button" )).click();
WebElement check_box1 = driver.findElement(By.xpath( "// *[contains(text(),'Amazon')]" ));
WebElement check_box2 = driver.findElement(By.xpath( "// *[contains(text(),'Flipkart')]" ));
check_box1.click();
System.out.println(check_box1.isSelected());
System.out.println(check_box2.isSelected());
}
}
|
Output:
true
false
Conclusion
In conclusion, Selenium can handle the checkboxes very easily and decrease the human effort of testing various operations related to checkboxes. The user can not only click the checkboxes but can also check if the checkboxes are clicked or not. I hope the various steps mentioned above will help you in handling the checkboxes using Selenium webdriver in Java.
FAQs
1. How can I handle checkboxes using Selenium WebDriver in Java?
To handle checkboxes in Selenium WebDriver with Java, you can use the isSelected() method to check if a checkbox is selected, and click() method to toggle its state.
2. How do I check if a checkbox is selected using Selenium WebDriver in Java?
You can use the isSelected() method on the WebElement representing the checkbox to determine if it’s selected or not.
3. How do I select a checkbox using Selenium WebDriver in Java?
Simply use the click() method on the WebElement representing the checkbox to toggle its state and select it.
4. How do I deselect a checkbox using Selenium WebDriver in Java?
To deselect a checkbox, you can again use the click() method on the WebElement representing the checkbox to toggle its state and deselect it.
5. Can I handle multiple checkboxes on a webpage using Selenium WebDriver in Java?
Yes, you can handle multiple checkboxes by locating each checkbox element individually and then performing actions on them using Selenium WebDriver methods like isSelected() and click().
Share your thoughts in the comments
Please Login to comment...