How to Open a New Tab using Selenium WebDriver in Java?
Last Updated :
15 Apr, 2024
An object-oriented platform-independent language that is used for creating applications is known as Java. Sometimes the applications are so robust that we need some automation to test their application. This can be done using various automation tools such as Selenium. While doing automation, there also occurs the need to open a new tab using the Selenium web driver in Java. In this article, we will discuss the same.
Opening a New tab using Selenium WebDriver in Java
We can open a new tab using Selenium WebDriver in Java by 2 methods given below:
Using newWindow API
Selenium allows the web driver to open a new tab and switch to that tab automatically using switchTo and newWindow functions. Here, we will use both functions simultaneously to open a new tab and switch to that.
- switchTo: The function which allows the webdriver to switch from one tab to another is known as ttswitchTo function.
- newWindow: The function that lets the user create a new window in the current browser session is known as newWindow.
Syntax:
driver.switchTo().newWindow(WindowType.TAB);
Example:
In this example, we have imported the WebDriver, ChromeDriver, and WindowType modules. Further, we have opened the Geeks For Geeks website (link) and then we have opened a new tab using the newWindow API.
Java
// Import selenium libraries
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WindowType;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium2 {
public static void main(String[] args)
{
// State the chromedriver URL
System.setProperty(
"webdriver.chrome.driver",
"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe");
// Define and initiate the chrome driver
WebDriver driver = new ChromeDriver();
// Open the Geeks For Geeks website
driver.get("https://www.geeksforgeeks.org/");
// Maximize the screen
driver.manage().window().maximize();
// Open a new tab using newWindow API
driver.switchTo().newWindow(WindowType.TAB);
}
}
Output:
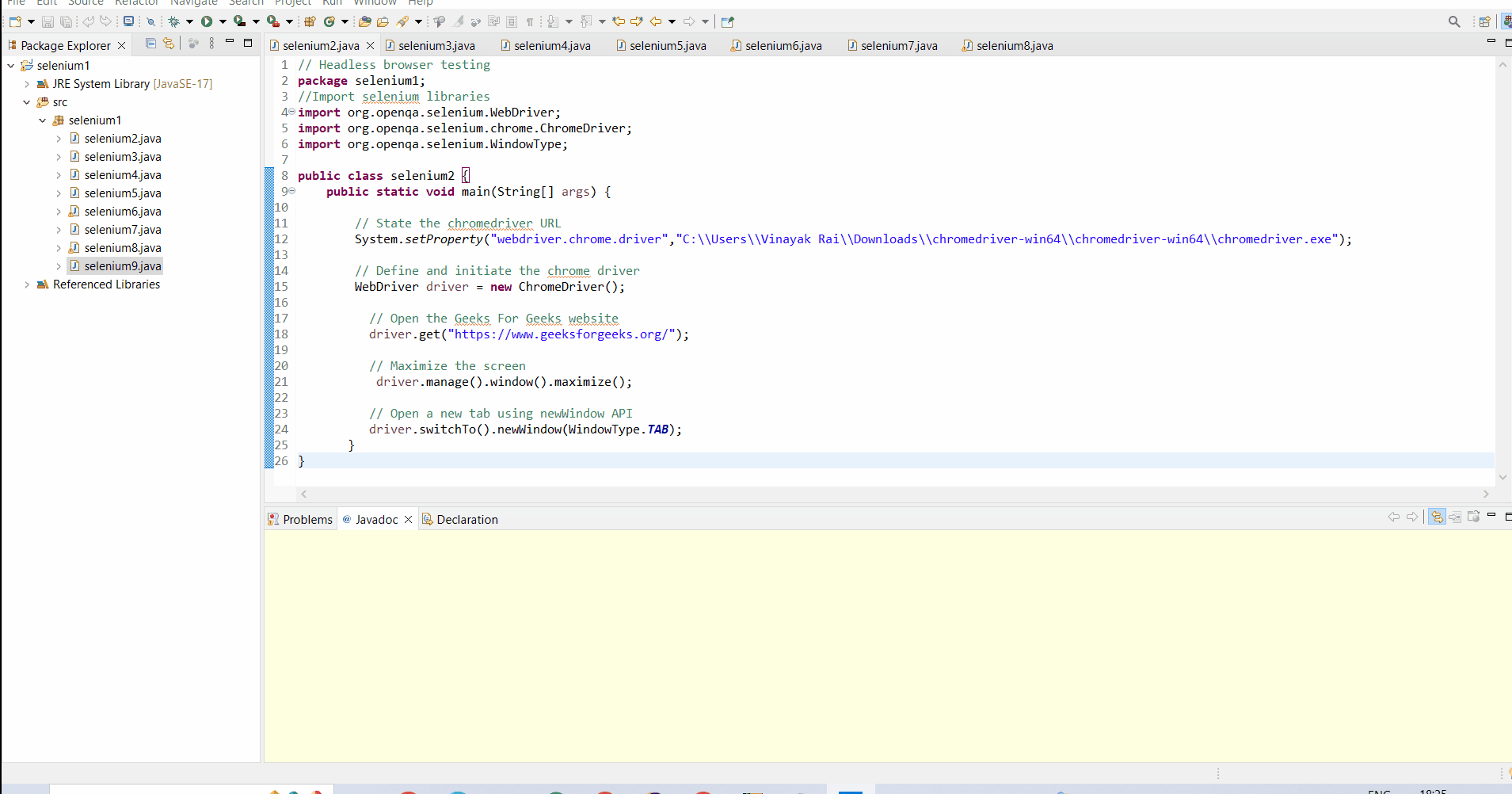
Using JavascriptExecutor
The module that allows the web driver to execute Javascript code within the current browser is known as JavascriptExecutor. The new tab can be opened using executeScript and window. open functions respectively.
- executeScript: The function that allows the user to execute JavaScript code within the context of the current browser window or frame is known as executeScript.
- window.open(): The function that is used to open a new browser window is known as a window.open() function.
There are Two Ways:
- Open a new tab
- Open a new tab with a specific URL
1. Open a new tab
When the user doesn’t provide any arguments to the window.open() function, then just a new tab is opened.
Syntax:
((JavascriptExecutor) driver).executeScript(“window.open()”);
Example:
In this example, we have imported the WebDriver, ChromeDriver, and JavascriptExecutor modules. Further, we have opened the Geeks For Geeks website (link) and then we have opened a new tab using JavascriptExecutor.
Java
//Import selenium libraries
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium2 {
public static void main(String[] args) {
// State the chromedriver URL
System.setProperty("webdriver.chrome.driver",
"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe");
// Define and initiate the chrome driver
WebDriver driver = new ChromeDriver();
// Open the Geeks For Geeks website
driver.get("https://www.geeksforgeeks.org/");
// Maximize the screen
driver.manage().window().maximize();
// Open a new tab using Javascript
((JavascriptExecutor) driver).executeScript("window.open()");
}
}
Output:
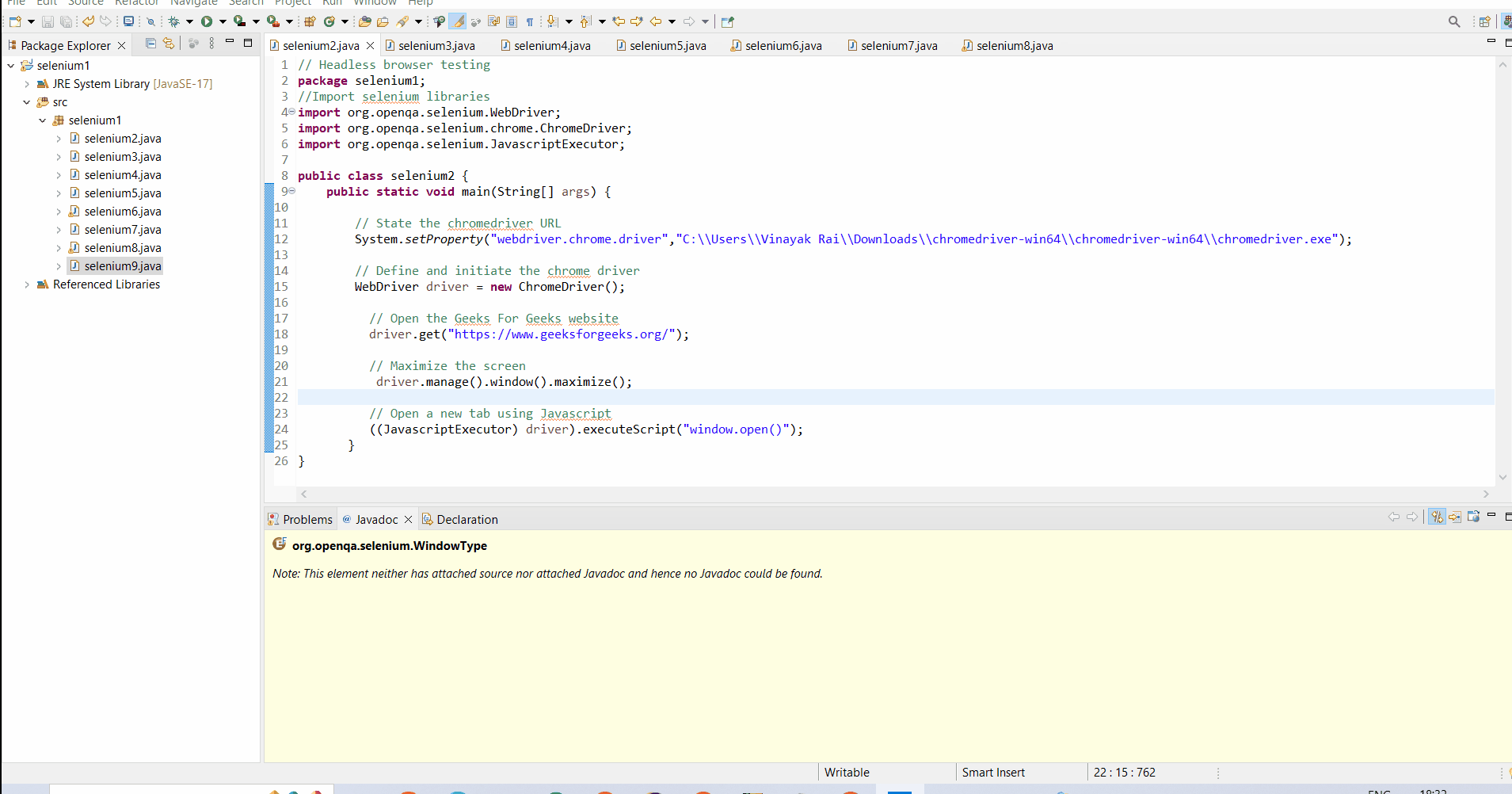
2. Open a new tab with a specific URL
When the user doesn’t provide a URL as the argument to the window.open() function, then a new tab with that specific URL is opened.
Syntax:
((JavascriptExecutor) driver).executeScript(“window.open(‘URL_of_webpage’)”);
Here, URL_of_webpage: It is the URL of the webpage which the user wants to open in a new tab.
Example:
In this example, we have imported the WebDriver, ChromeDriver, and JavascriptExecutor modules. Further, we have opened the Geeks For Geeks website (link) and then we have opened a new tab with a specific URL (link) using JavascriptExecutor.
Java
//Import selenium libraries
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium2 {
public static void main(String[] args) {
// State the chromedriver URL
System.setProperty("webdriver.chrome.driver",
"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe");
// Define and initiate the chrome driver
WebDriver driver = new ChromeDriver();
// Open the Geeks For Geeks website
driver.get("https://www.geeksforgeeks.org/");
// Maximize the screen
driver.manage().window().maximize();
// Open a new tab with specific URL using Javascript
((JavascriptExecutor) driver).executeScript("window.open('https://www.geeksforgeeks.org/explore?page=1&sortBy=submissions&itm_source=geeksforgeeks&itm_medium=main_header&itm_campaign=practice_header')");
}
}
Output:
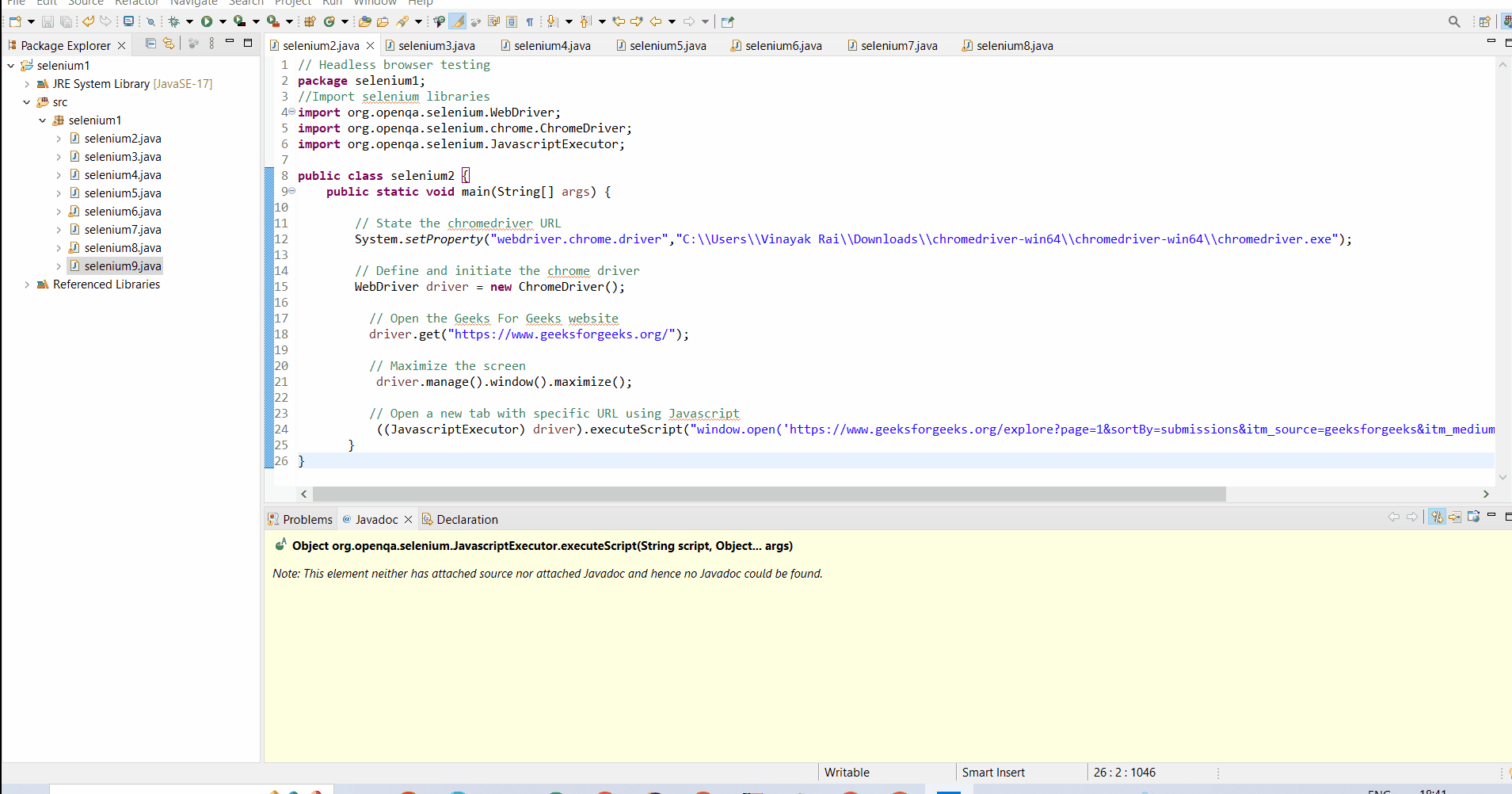
Conclusion
The opening of a new tab is a very important feature during automation and enhances the capability of automated testing. If the user wants to open a new tab with a specific URL, then opening a new tab with a specific URL using the JavascriptExecutor method is preferred. I hope after reading the above article, you will able to open a new tab using Selenium webdriver in Java.
Share your thoughts in the comments
Please Login to comment...