How to Click on a Hyperlink Using Java Selenium WebDriver?
Last Updated :
15 Apr, 2024
An open-source tool that is used to automate the browser is known as Selenium. Automation reduces human effort and makes the work comparatively easier. There are numerous circumstances in which the user wants to open a new page or perform a certain action with the click of the hyperlink. In this article, we will discuss how we can click on a hyperlink using Java Selenium WebDriver.
Steps to Click on a Hyperlink Using Java Selenium WebDriver
In this section, we will explore the step-by-step process of clicking on a Hyperlink Using Java Selenium WebDriver.
Step 1: Setting up the Selenium in Java
Install the IDE of your choice for running Java and download the latest Selenium Jars available from Selenium’s official website. Create the Java project, and add the external Jars.
Right click on project -> Select Build Path -> Choose Configure Build Path -> Go to libraries tab -> Click on Classpath -> Select Add external JARs -> Choose all Selenium JARs -> Click on Apply and Close
Step 2: Installing the necessary modules
- By: The library used to locate elements within the document is known as the By module.
- WebDriver: The module used for creating robust browser-based regression test suites is known as the WebDriver module.
- ChromeDriver: An open-source tool used to control Chrome autonomously is called ChromeDriver.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver
Step 3: Initiating the webdriver and navigate to a webpage
In this step, we have stated the location of ChromeDriver inside the setProperty function and further initiated the ChromeDriver. Here, we have opened the Geeks for Geeks website (link) using the get() method.
Note: Here, we have used Chrome for the automation, you can use any other browser too and initialize the browser driver here.
Java
// Import selenium libraries
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium2 {
public static void main(String[] args) {
// State the chromedriver URL
System.setProperty("webdriver.chrome.driver"
,"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe");
// Define and initiate the chrome driver
WebDriver driver = new ChromeDriver();
// Open the Geeks For Geeks website
driver.get("https://www.geeksforgeeks.org/");
}
}
Step 4: Finding an element
In the website opened, we find the hyperlink text ‘Data Structures & Algorithms‘ which you want to click using By.LinkText and findElement functions.
- findElement: The function used to locate a web element on a webpage on the distinct locators like Id, xpath, etc. is known as the findElement function. It returns the matching element found on the webpage.
- By.LinkText: The By.LinkText function searches for the exact hyperlink on the webpage, which can be further used for various actions.
Java
//Import selenium libraries
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium2 {
public static void main(String[] args) {
// State the chromedriver URL
System.setProperty("webdriver.chrome.driver"
,"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe");
// Define and initiate the chrome driver
WebDriver driver = new ChromeDriver();
// Open the Geeks For Geeks website
driver.get("https://www.geeksforgeeks.org/");
// Maximize the screen
driver.manage().window().maximize();
// State the hyperlink which you want to click
WebElement link_text = driver.findElement(By.linkText("Data Structures & Algorithms"));
}
}
Output:
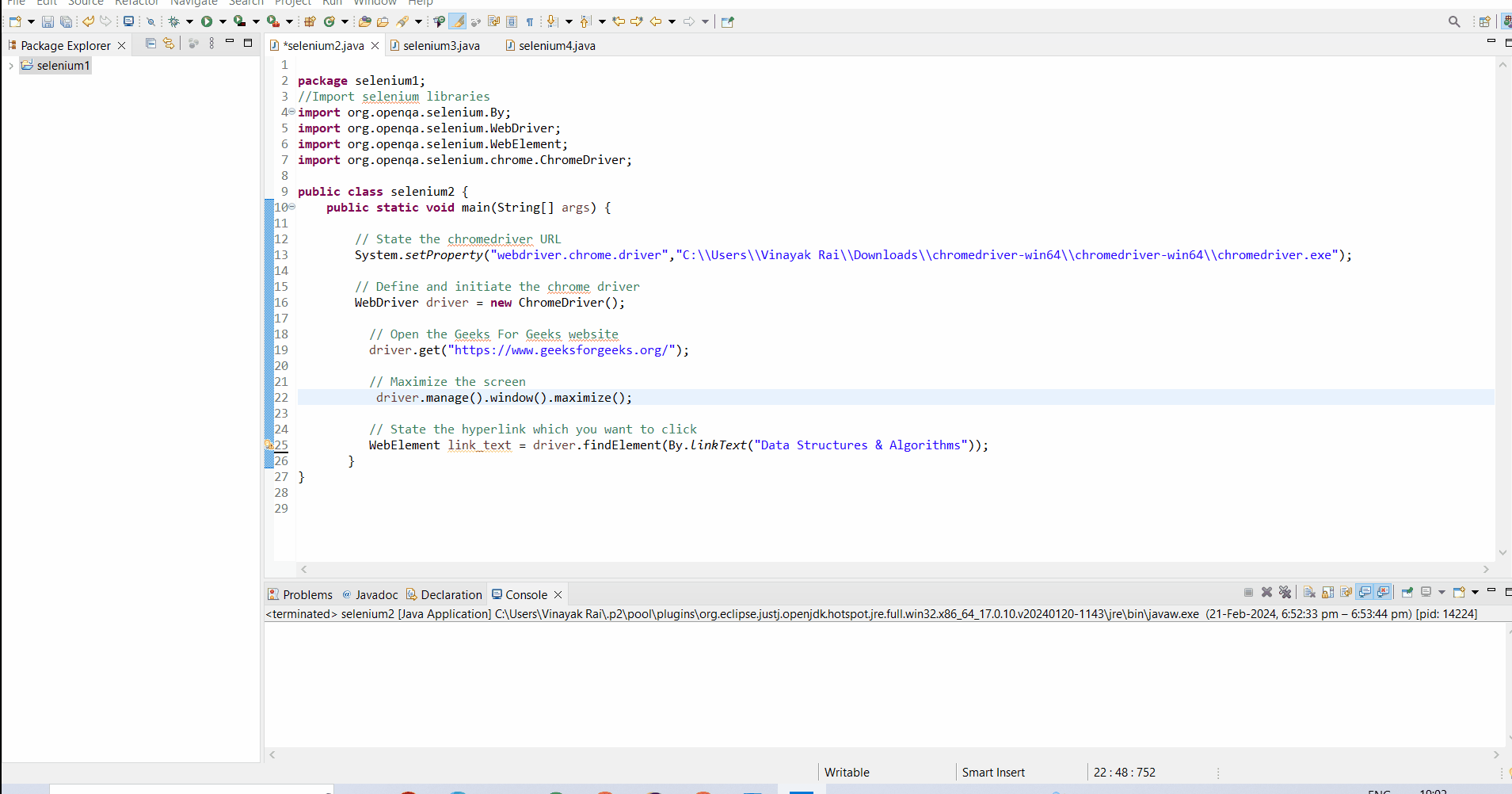
Step 5: Click on an element
Once we have found the element on the respective page, click on that hyperlinked text using the click() function.
- click(): The function that is used to simulate a mouse click on a web element is called the click() function. It is used with clickable objects, such as buttons, checkboxes, etc.
Java
//Import selenium libraries
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium2 {
public static void main(String[] args) {
// State the chromedriver URL
System.setProperty("webdriver.chrome.driver"
,"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe");
// Define and initiate the chrome driver
WebDriver driver = new ChromeDriver();
// Open the Geeks For Geeks website
driver.get("https://www.geeksforgeeks.org/");
// Maximize the screen
driver.manage().window().maximize();
// State the hyperlink which you want to click
WebElement link_text = driver.findElement(By.linkText("Data Structures & Algorithms"));
// Clicking on the element
link_text.click();
}
}
Output:
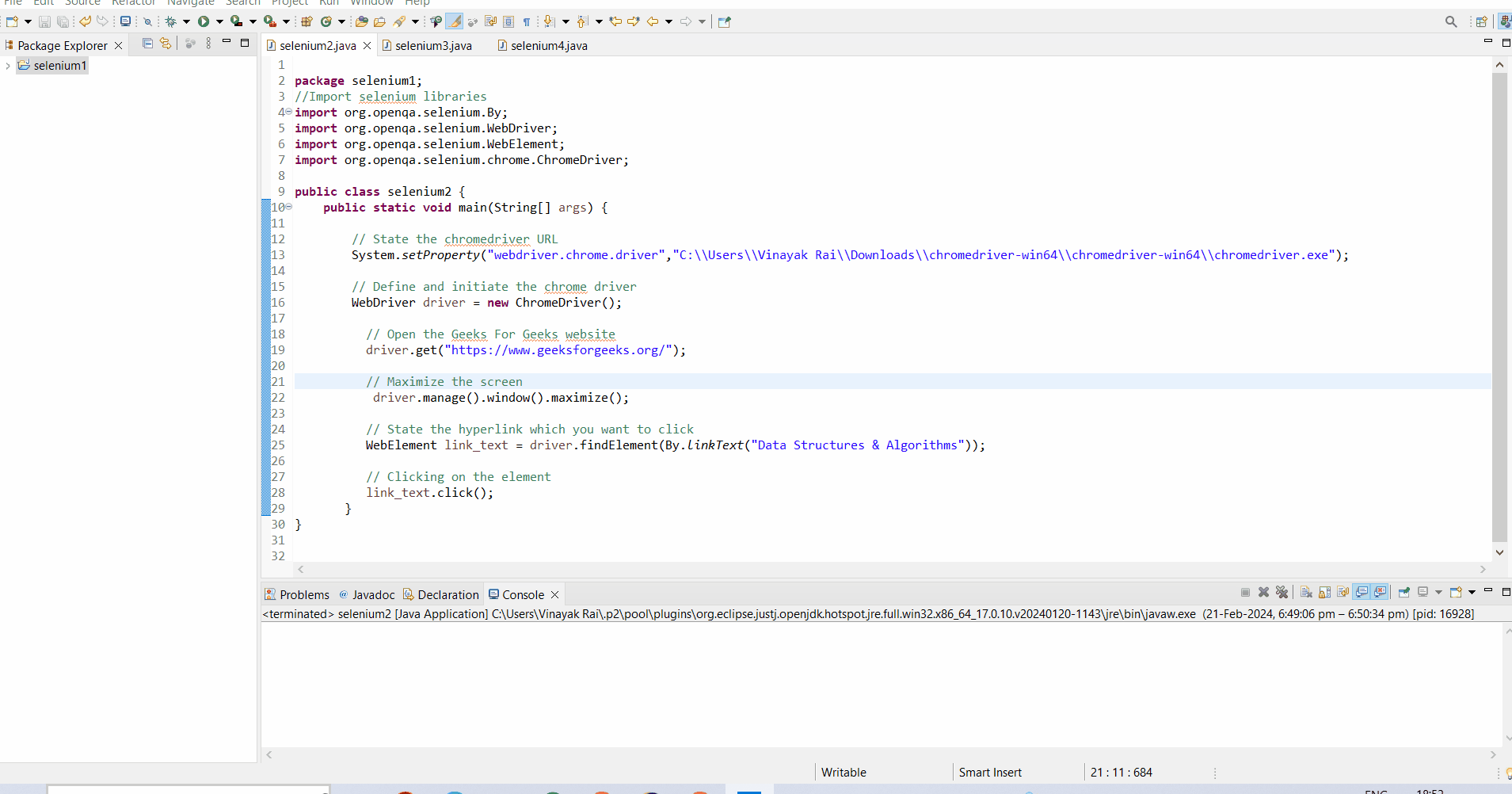
Conclusion
In conclusion, selenium is one of the most common tools used for automation in the market. It has the power to control all the necessary actions to be performed on the website. In this article, we have discussed the steps for clicking on a hyperlink using Selenium webdriver in Java. I hope, this is clear and it will help you in performing the necessary action.
Share your thoughts in the comments
Please Login to comment...