Understand Java Interface using Selenium WebDriver
Last Updated :
01 Dec, 2023
Java Interface is a blueprint of the class that contains static constants and abstract methods. It cannot have a method body. The interface is a mechanism to achieve abstraction. This article focuses on discussing the Selenium WebDriver Interface.
Example:
public interface Animal
{
void printAnimalName();
void printAnimalColor();
void printAnimalWeight();
}
In the above example, an Interface “Animal” is created and three non-implemented methods are declared. Now, it’s just a blueprint, there is no implementation. Any class that implements this interface must implement the non-implemented methods, or Java will throw an error. Here is what the implemented class looks like. In the below class “Cat”, we have implemented the three non-implemented methods.
public class Cat implements Animal
{
@Override
public void printAnimalName()
{
System.out.println(“Cat”);
}
@Override
public void printAnimalColor()
{
System.out.println(“Black”);
}
@Override
public void printAnimalWeight()
{
System.out.println(“50”);
}
}
Need for an Interface in Java
Selenium supports various web browsers for automation such as Chrome, Firefox, Safari, Edge, etc. It doesn’t depend on what browser we are choosing for our automation, we must have some common methods available to work with these browsers. For example:
- Load the URL: get() or navigate()
- Find a WebElement: findElement()
- Get the current URL: getCurrentURL()
- Close the browser: close() or quit()
We have different classes available to open different browsers, such as:
Now, let’s assume four developers are writing the code for these four classes, the developer who is writing code for ChromeDriver gives the method name to load the URL as getURL(), whereas another developer writing code for EdgeDriver gives the method name as loadURL(). Try to understand the problem for us as end users, we have to remember three different methods to load the URL, do you think it’s a good practice? Of course not, that’s when the importance of the Interface comes into the picture.
How does Selenium use Java Interface?
To overcome this problem, Selenium Developers have created an Interface called “WebDriver” and defined all the must-have methods inside it without implementing it.
- Any class implementing the WebDriver Interface now has to use and implement the same set of methods, or Java will throw an error.
- As a result, whether it’s Chrome, Edge, Firefox, or Safari, all the implementing classes must have the same set of methods with the same name.
This is how the Interface achieves abstraction, i.e. hiding all the implementing parts for various browsers, getting the method name, and using it in your code.
In the below image, the WebDriver interface and its methods are shown in the centre. These methods are not implemented yet. The classes are mentioned in the circle that implement the WebDriver interface.
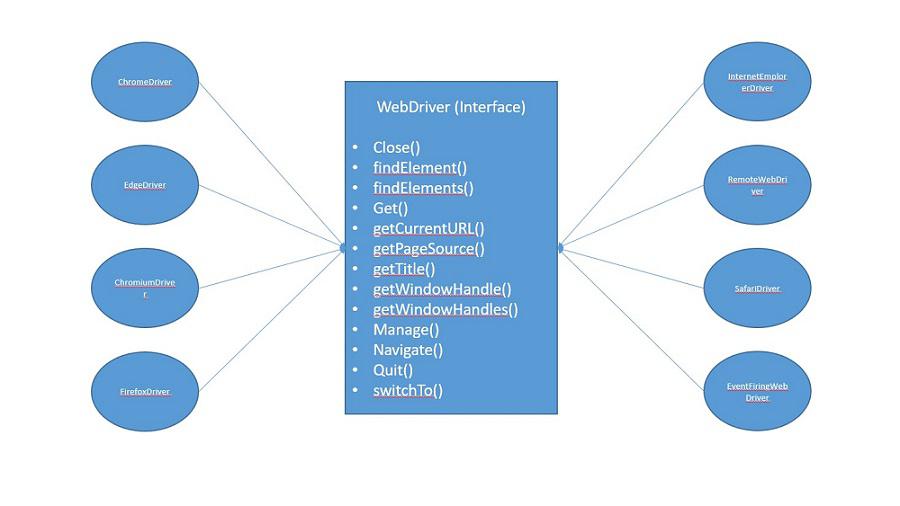
WebDriver Interface
Below is the Java program to implement interface:
Java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class InterfaceExample {
public static void main(String args[])
throws InterruptedException
{
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get(
WebElement element = driver.findElement(
By.xpath( "//input[@placeholder='First Name']" ));
element.sendKeys( "Selenium" );
driver.quit();
}
}
|
Explanation:
- WebDriver driver = new ChromeDriver(): Here WebDriver is an Interface and ChromeDriver is the implementing class.
- driver.get(): get() method is declared in WebDriver Interface. Hence the ChromeDriver class must define the method get(), which is used to open a URL on the Chrome Browser. Similarly, FirefoxDriver implements the method get() to open a URL on the Firefox Browser.
- driver.findElement(): findElement() is another method that is declared in the WebDriver interface and all the classes that implement the WebDriver interface must define its definition. The same goes for driver.quit() as well.
- element.sendKeys(): Enter a value in the text field.
Output:
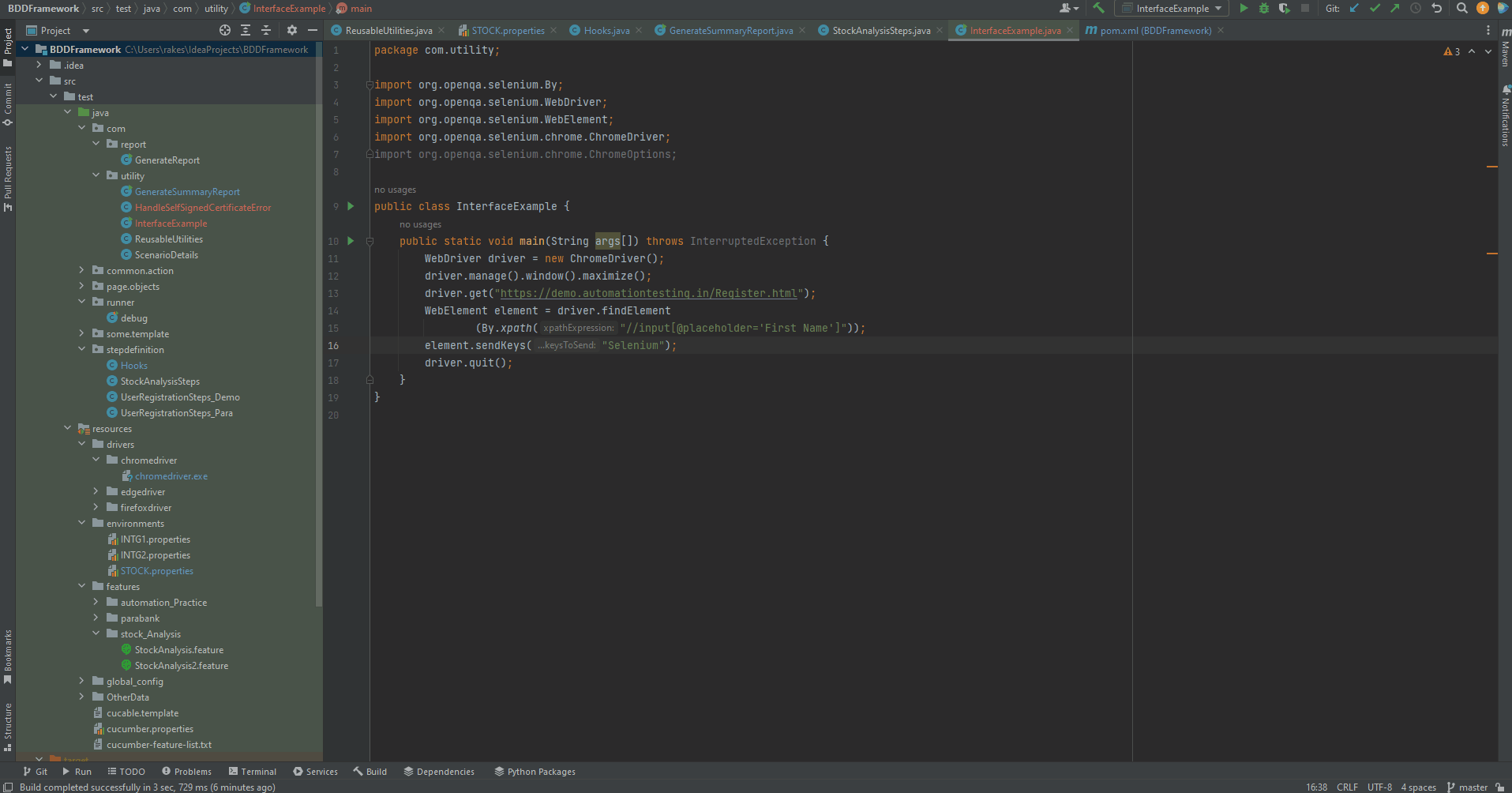
Output
Conclusion
While learning Java Interface we always tend to see some common and easy examples either from blogs or from books which are good for beginners. But as we progress in our careers, we should understand the real-life applications of Java Interface. Selenium is a popular tool today in the Test Automation Industry. From this article, we have learned how Selenium WebDriver uses the Java Interface concept internally and what is the importance of Java Interface. Without the Selenium WebDriver interface, it’s tough to manage multiple browser-implementing classes to have similar features.
Share your thoughts in the comments
Please Login to comment...