How to ask the Selenium-WebDriver to wait for few seconds in Java?
Last Updated :
04 Mar, 2024
An open-source framework that is used for automating or testing web applications is known as Selenium. There are some circumstances when the particular component takes some time to load or we want a particular webpage to be opened for much more duration, in that case, we ask the Selenium web driver to wait for a few seconds. In this article, we will discuss the same.
What is Selenium WebDriver?
An automated driver that provides a programming interface for interaction with elements on web pages, thus enabling automated testing of web applications across different browsers and platforms is known as a Selenium web driver. It allows users the features such as automation, cross-browser compatibility implicit and explicit wait, etc.
How to Ask the Selenium-WebDriver to Wait for a Few Seconds in Java?
There are 3 ways to ask the Selenium-WebDriver to wait for a few seconds in Java. These are:
1. Using Sleep Function
The function that stops the execution of the script for a specified amount of time is known as the sleep function. The worst part of the sleep function is that it introduces unnecessary delays in case the user is adding sleep just for loading of previous element. In this method, we will see how we can ask the Selenium web driver to wait using the sleep function.
Syntax:
Thread.sleep(milliseconds); Here, milliseconds: These are the number of milliseconds the user wants the Selenium webdriver to wait.
Example: In this example, we have opened the GeeksForGeeks website, and then we have asked the Selenium driver to wait for 5 seconds using the sleep function before closing the Chromedriver.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium5 {
public static void main(String[] args) throws InterruptedException {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
Thread.sleep( 5000 );
driver.quit();
}
}
|
Output:
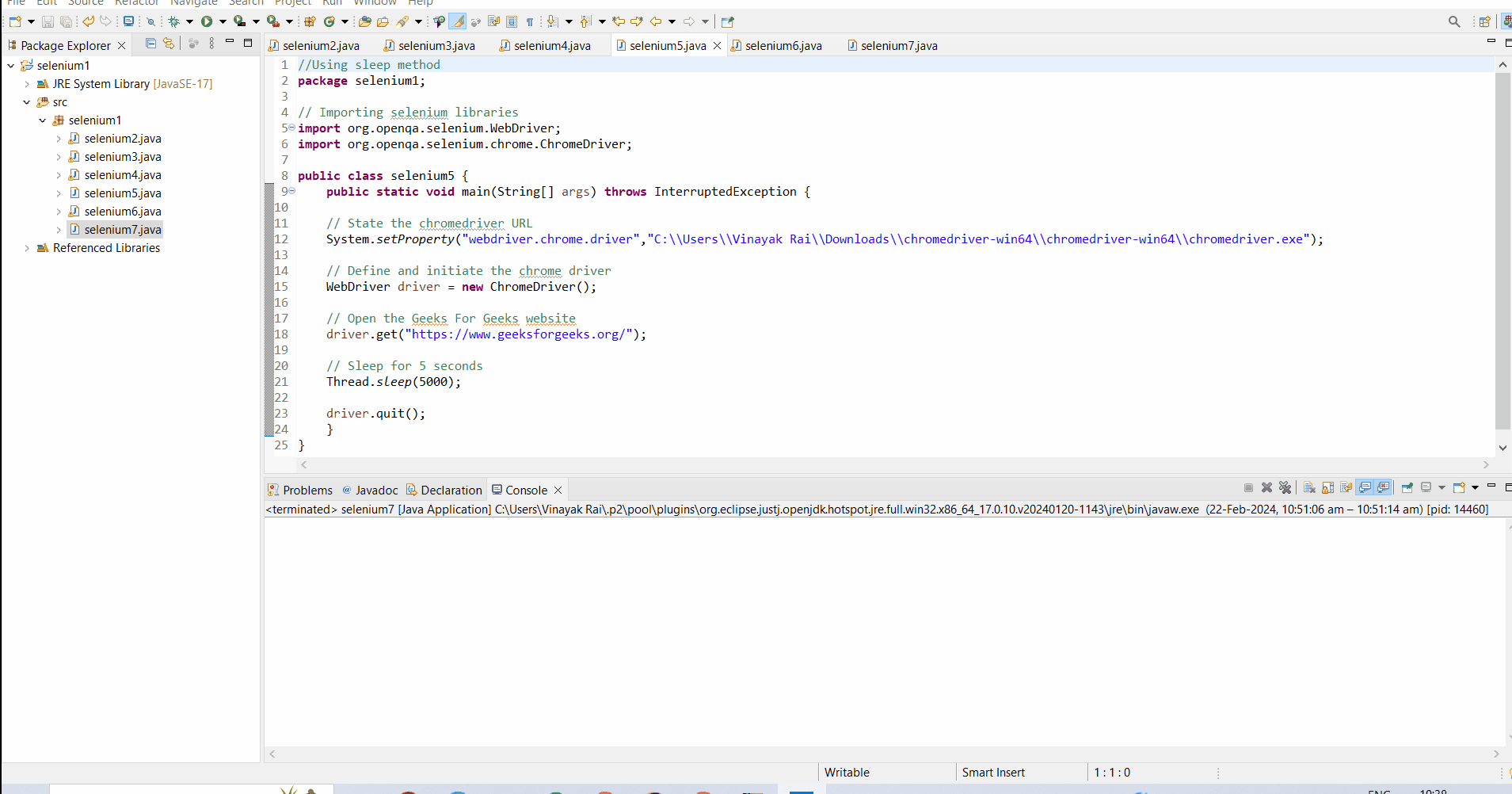
2. Using Implicit Wait
- A framework that lets the webdriver wait a certain amount of time before throwing the NoSuchElementException error is known as implicit wait. This can be achieved using the inbuilt functions in Selenium, i.e., implicitlyWait.
- The implicitwait function sets the amount of time the WebDriver instance should wait in case an element webdriver is finding if it’s not immediately available.
Syntax:
driver.manage().timeouts().implicitlyWait(seconds, TimeUnit.SECONDS); Here, seconds: These are the number of seconds the user want Selenium webdriver to wait.
Example: In this example, we have opened the GeeksForGeeks website and then asked the Selenium driver to wait for 5 seconds before throwing the NoSuchElementException error.
Java
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium6 {
public static void main(String[] args) throws InterruptedException {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait( 5 , TimeUnit.SECONDS);
driver.quit();
}
}
|
Output:
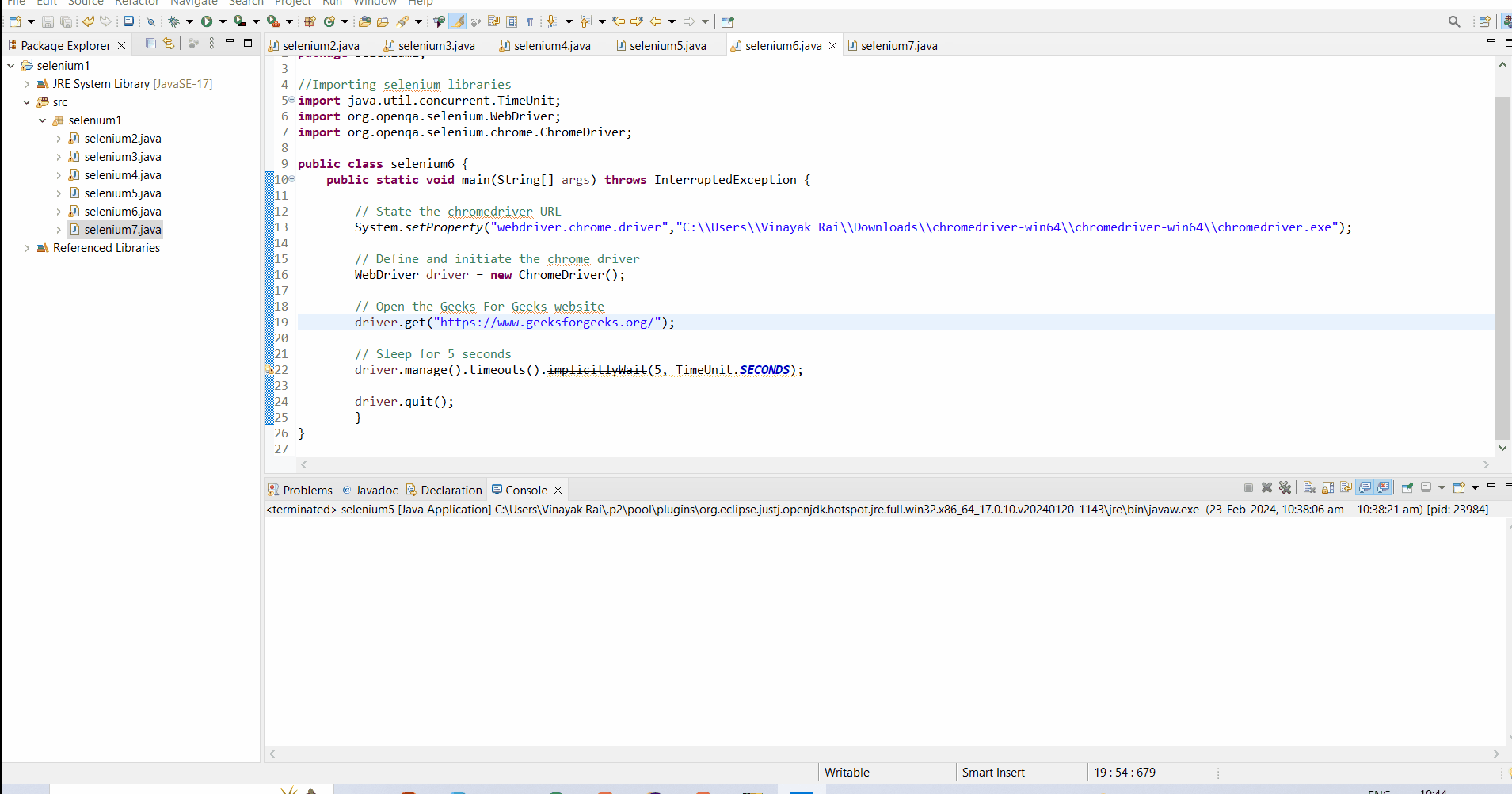
3. Using Explicit Wait
The way of telling Selenium webdriver to wait for a certain amount of time before throwing any exception is known as explicit wait. It is the most efficient way of asking Selenium webdriver to wait until the element is loaded. It can be achieved using the inbuilt functions of Selenium:
1. WebDriverWait: The method that lets the webdriver wait for a certain condition to be met before execution of the next element is known as the WebDriverWait function.
Syntax:
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(seconds)); Here, seconds: These are the number of seconds the user want Selenium webdriver to wait.
2. ExpectedConditions.visibilityOfElementLocated: It lets the chromedriver wait for a certain time before the specific element is visible to the webdriver or user.
Syntax:
wait.until(ExpectedConditions.visibilityOfElementLocated(By.linkText(content))); Here, content: It is the content that needs to be loaded before execution of the next element.
Example: In this example, we have opened the GeeksForGeeks website and then asked the Selenium driver to wait for 5 or more seconds before finding an element.
Java
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class selenium7 {
public static void main(String[] args) throws InterruptedException {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds( 5 ));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.linkText( "Data Structures & Algorithms" )));
driver.quit();
}
}
|
Output:
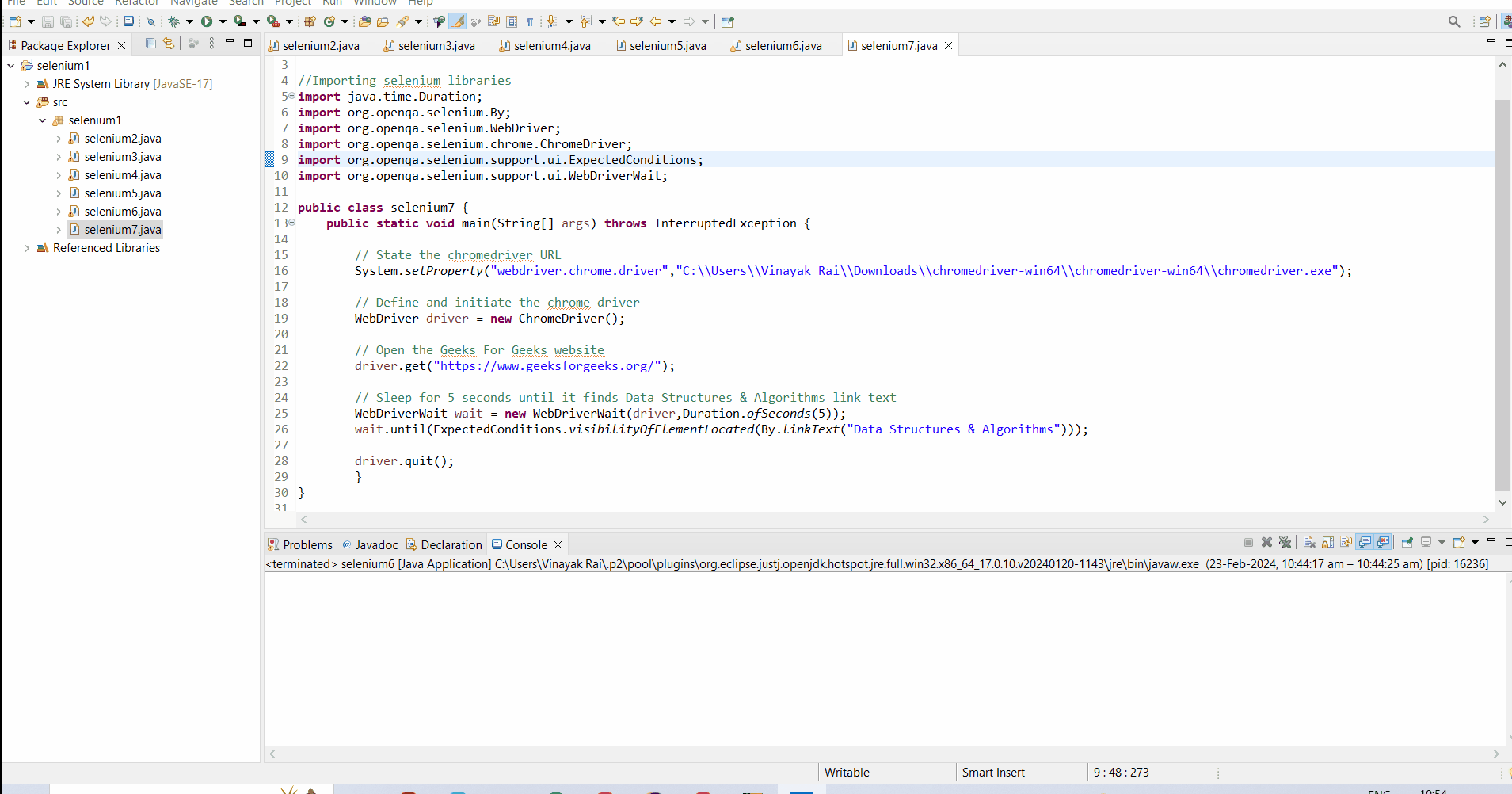
Comparison of Different Wait Methods
In the following table, we have compared the various sleep methods, implicit wait, explicit wait, and sleep function.
Parameters
|
Sleep Function
|
Implicit Wait
|
Explicit Wait
|
Wait time
|
It makes the web driver wait for the specified time, irrespective of whether the element is found or not.
|
It does not make web drivers wait for the complete duration of time. If the element is found before the duration specified, it moves on to the next line of code execution.
|
It makes the web driver stop the execution of the script based on a certain condition for a specified amount of time.
|
Loading of element
|
As you don’t know how much time will the element take to load so you have to predict the sleep function time unless that works.
|
In implicit wait, you can enter the maximum time you think an element will take to load, it loads earlier, and then the next line will be executed automatically.
|
In explicit wait, it is not necessary to enter the time, once the element is loaded, the next line is automatically executed.
|
NoSuchElementException error
|
It throws a NoSuchElementException error in case the element is not found after the sleep of the web driver is completed.
|
The NoSuchElementException error is thrown in case the element is not found in the specified time set by the user.
|
There is no chance of a NoSuchElementException error here as the next line is executed only when the element has been loaded properly.
|
Best Practices for Waiting in Selenium-WebDriver
- Avoid sleep function: As the sleep function does not execute the next lines of code until the specified time is over, thus it adds unnecessary delays. Therefore, it is better to avoid sleep function.
- Use Explicit Waits Wisely: The explicit wait is the best waiting technique, still, it is recommended to use it wisely as excessive use of waits can slow down your tests.
- Optimize Timeout Values: It is very crucial to set proper time with sleep and implicit wait function depending on factors like network, loading of website, etc.
- Constantly Review and Enhance Waiting Techniques: The user should review the automation script at regular intervals and modify the waiting techniques, if necessary.
- Use Page Object Model: In case of the huge scripts, it is necessary to follow the page object model as it encapsulates waiting logic within page objects and makes tests more readable and maintainable.
Handling Dynamic Elements with Waiting Strategies
- Explicit Waits: In the case of dynamic elements, explicit wait works best as it makes the script wait until it is necessary to make that element visible and clickable.
- Identify Stable Locators: It is suggested to use the locators that are expected not to change to find an element. This will reduce your effort of changing the script again and again and make it more dynamic.
- Retry Mechanisms: If you are executing a script on a large scale, then don’t forget to add a retry mechanism too in the script as it will reduce the failure of the script.
- Fluent Waits: This wait is almost similar to explicit wait, but it is useful while polling as it provides users the flexibility in defining polling intervals.
- Custom Wait Conditions: In case, you don’t wait to use any of the above-stated waiting methods, then you can create your waiting method by using if-else conditions for checking if the element is loaded or not.
- Impact on Test Execution Time: As the user wants the script to be executed in minimum time with much efficiency, thus it is necessary to optimize the wait time. This can be done by reducing the wait or giving the necessary time to wait only.
- Network Latency: This is one of the most crucial factors as less network latency increases the script execution time. Thus, it’s better to connect to a good internet for running the test.
- Resource Consumption: As implicit and explicit waits continuously keep on checking if the element is found or not, thus it consumes many resources. Therefore, it is recommended to monitor resource usage during test execution.
- Browsing Cache: The temporary files which get stored in the browser are known as browsing cache. It can be very crucial as the data already loaded once will not be loaded again, thus increasing performance.
- Parallel Execution: The multiple scripts can be run at the same time across multiple threads to reduce overall test execution time.
Conclusion
We can say, most commonly, the user wants the selenium web driver to wait until the element is loaded, thus the explicit wait is the best approach as it lets the web driver wait only until the element is found. Also, it eliminates the chance of the web driver throwing the NoSuchElementException error. I hope the various ways mentioned in the above article will help you do your work smoothly.
Frequently Asked Questions
1. Is it possible to set a default wait time for all elements in Selenium WebDriver?
Yes, this is certainly possible by using implicit wait which lets web driver wait for a certain amount of time when trying to find an element. You can use the following line of code.
driver.manage().timeouts().implicitlyWait()
2. Can we set a maximum timeout for waiting operations in Selenium WebDriver?
Yes, a maximum timeout for waiting operations can be set by specifying a timeout duration. This can be done by creating WebDriverWait instances, that ensure that the tests don’t wait indefinitely if the expected condition is not met.
3. If I want to continuously repeat my script after every 1 minute, then how can I do that?
You can do that by creating a loop of how many times you want to execute the script, by adding the Thread.sleep function, which makes the web driver wait for a specified time irrespective of the loading of the element.
Share your thoughts in the comments
Please Login to comment...