Selenium WebDriver Handling Radio Buttons Using Java
Last Updated :
11 Mar, 2024
A platform-independent language that is used to build various applications is known as Java. Java can also be used to automate the web drivers. There are various automation tools available, out of which Selenium is the most common one. We can automate the opening of the website, clicking of push button, and enabling radio buttons or checkboxes. In this article, we will discuss how we can handle the radio buttons using a selenium web driver in Java.
Selenium WebDriver Handling Radio Buttons Using Java
For handling the radio buttons using the Selenium web driver in Java, we need to follow the respective steps:
Step 1: Setting up the Selenium in Java
Set up Selenium in Java by downloading the latest JARs from the official website, then add them to your project’s build path using “Configure Build Path” and selecting “Add External JARs” in Eclipse or a similar IDE.
Step 2: Installing the necessary modules
We need to install the By WebDriver and ChromeDriver modules.
- By: The By module is used to locate elements within the document and perform a specific action on them.
- WebDriver: The WebDriver is a remote control interface that is used to create robust browser-based regression test suites.
- ChromeDriver: An open-source tool that is used for automated testing of web apps is called ChromeDriver.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
Step 3: Initiating the web driver and navigate to the webpage
Now, we have defined the path where the Chrome driver is present and then initiated the Chrome driver by using the ChromeDriver module we imported. As Chrome is the most common browser used in daily life, thus we have used the ChromeDriver. You can use any other browser driver of your choice too. Here, we have opened the GeeksforGeeks website (link) for handling the radio buttons.
- get(): The get() function is used to open the URL in the browser. It will navigate to the webpage in which we want to handle the radio buttons using the get() function.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium4 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
}
}
|
Step 4: Finding an element
Further, we find the element using findElement, By.xpath, and contains functions available in Selenium.
- findElement: The function that is used to return the first matching occurrence on the webpage is called the findElement function.
- By.xpath: The crucial language that is used in navigation for HTML documents is known as Xpath, while the function that is used to locate the element based on Xpath is known as the By.xpath function.
- contains(): When we need to locate an element that contains a certain substring and not the complete string, we use the contains() function.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium4 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.findElement(By.xpath( "/html/body/div[1]/div/div[3]/div[2]/div/div[2]/div/div/div/button" )).click();
WebElement boolean_value = driver.findElement(By.xpath( "// *[contains(text(),'SDE Sheet')]" ));
}
}
|
Output:
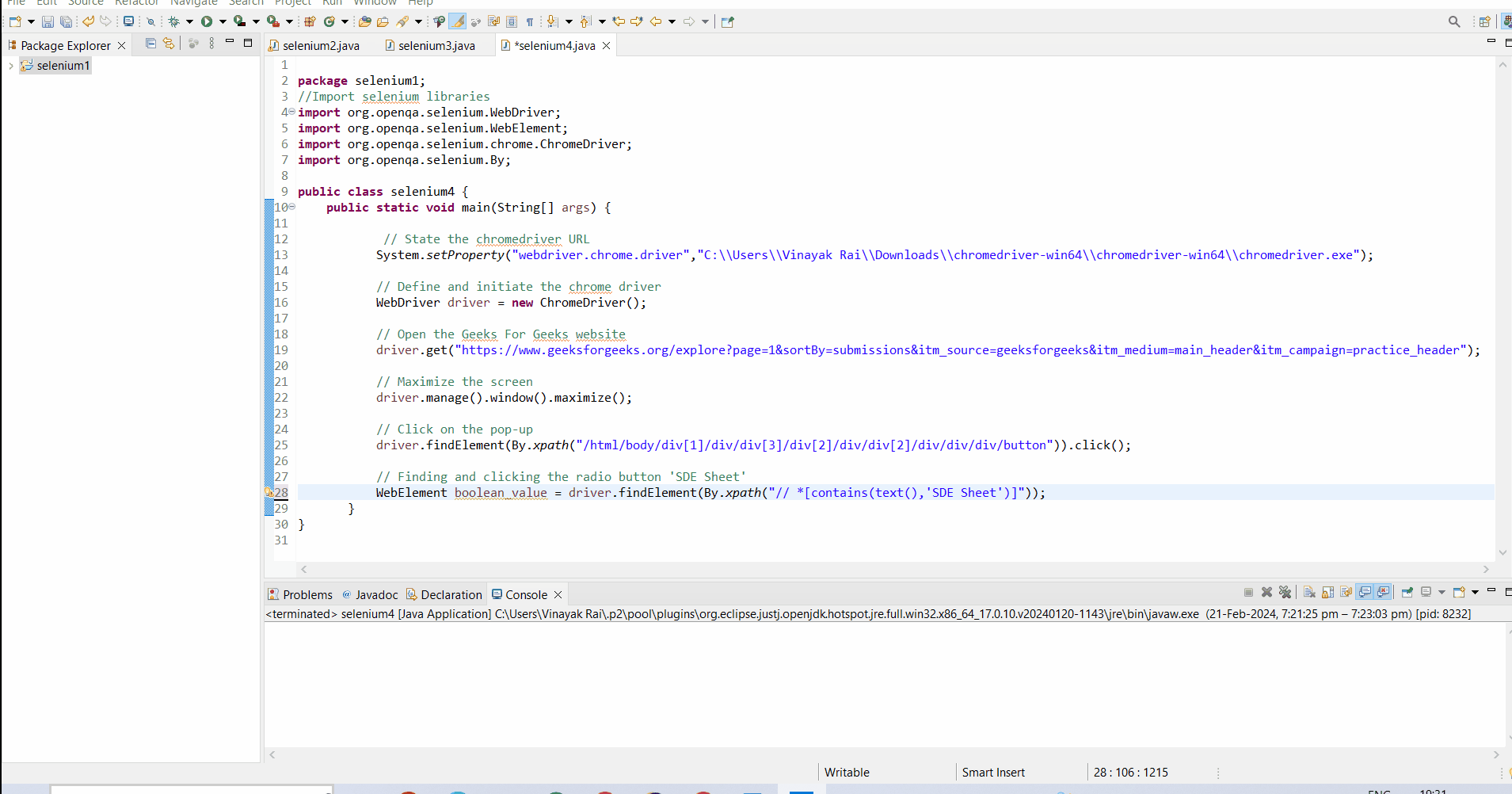
Step 5: Click on an element
Finally, we will click an element, i.e., the radio button name ‘SDE Sheet‘ found on the website using the click function.
- click(): The function which is used for interacting with the clickable elements, like buttons, link, etc is called the click function.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium4 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" , "C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.findElement(By.xpath( "/html/body/div[1]/div/div[3]/div[2]/div/div[2]/div/div/div/button" )).click();
WebElement boolean_value = driver.findElement(By.xpath( "// *[contains(text(),'SDE Sheet')]" ));
boolean_value.click();
}
}
|
Output:
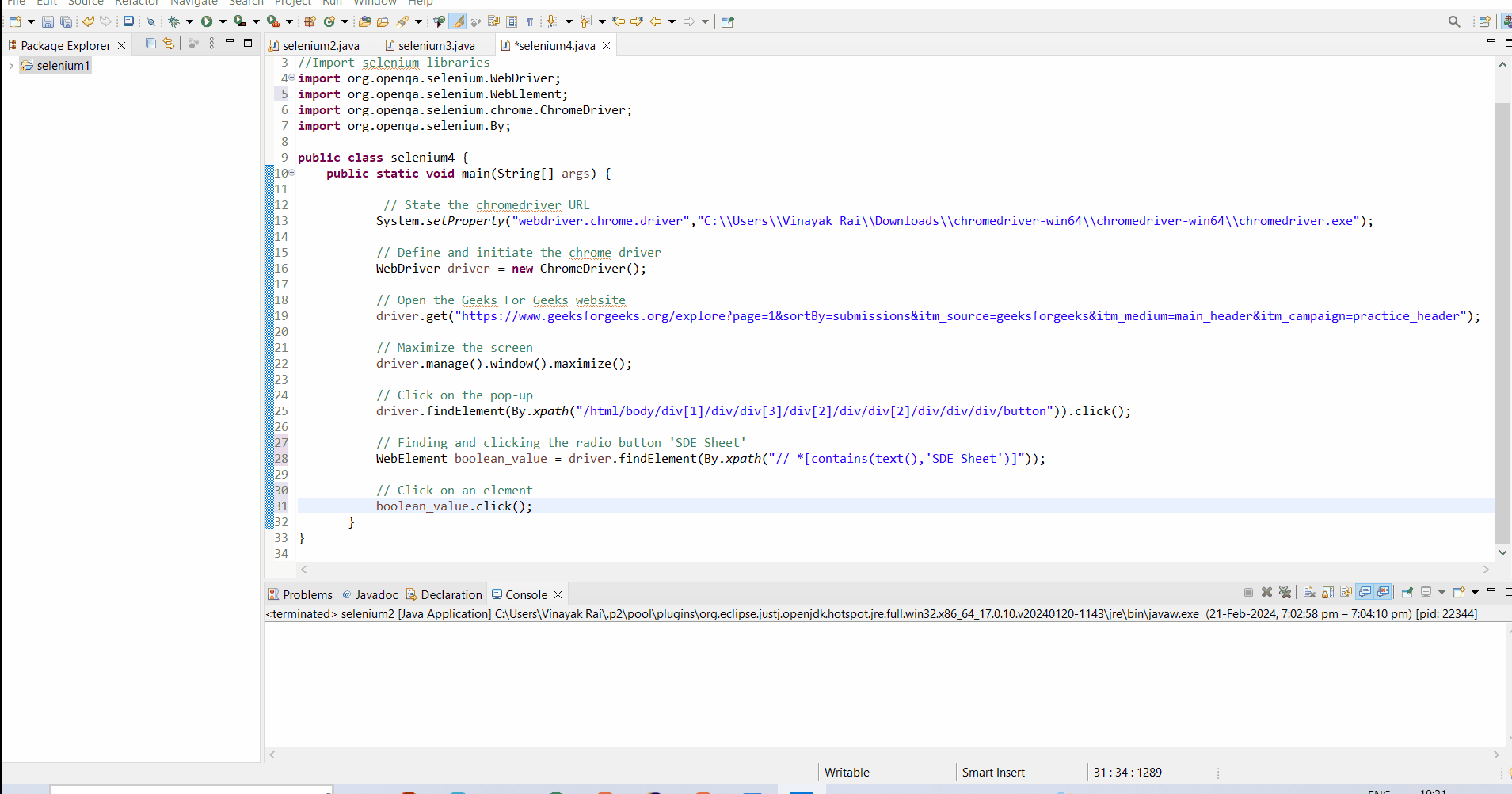
Conclusion
In conclusion, handling radio buttons using Selenium WebDriver in Java involves setting up Selenium, installing necessary modules like By WebDriver and ChromeDriver, initializing the WebDriver, navigating to the webpage, finding the radio button element, and clicking on it using Selenium functions. This allows the automation of interactions with radio buttons on web pages, enhancing the efficiency of web testing and interaction processes.
Share your thoughts in the comments
Please Login to comment...