SAP ABAP | Polymorphism
Last Updated :
30 Nov, 2023
Polymorphism in SAP ABAP is a core principle in Object-Oriented Programming (OOP) that enables different classes to be handled as instances of a shared class via a common interface. This allows developers to apply techniques in various ways while storing a constant user interface. In SAP ABAP, the integration of polymorphism is pivotal for augmenting code flexibility and ensuring ease of maintenance.
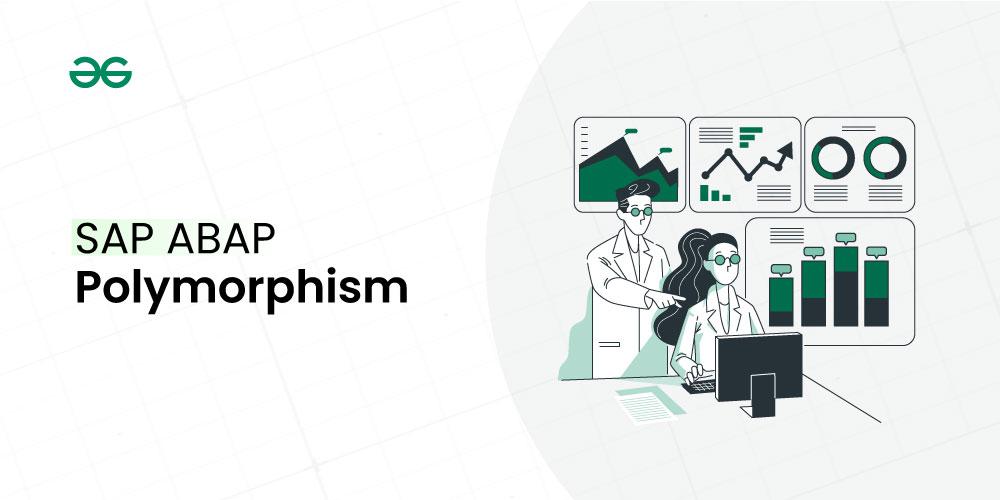
SAP ABAP | Polymorphism
What is Polymorphism in SAP ABAP?
In SAP ABAP (Advanced Business Application Programming), polymorphism refers to the ability of a single function or method to operate on different types of data or objects. Polymorphism is a fundamental concept in object-oriented programming (OOP) and is one of the four pillars of OOP, along with encapsulation, inheritance, and abstraction.
Types of Polymorphism in SAP ABAP:
In SAP ABAP, polymorphism is a fundamental concept that allows different objects to be treated as instances of the same class, enabling flexibility and code reuse. There are two main types of polymorphism in SAP ABAP:
1. Compile-Time Polymorphism (Static Binding) in SAP ABAP:
- Also called method overloading or early binding.
- Occurs at compile time.
- Multiple methods in the same class have the same name but different parameters (different numbers or types of parameters).
- The correct method to be executed is determined at compile time-based on the method signature.
Example:
CLASS compile_time_polymorphism DEFINITION.
PUBLIC SECTION.
METHODS print_message IMPORTING im_message TYPE string.
METHODS print_message IMPORTING im_message TYPE string im_count TYPE i.
ENDCLASS.
CLASS compile_time_polymorphism IMPLEMENTATION.
METHOD print_message.
WRITE: 'Message:', im_message.
ENDMETHOD.
METHOD print_message.
WRITE: 'Message:', im_message, 'Count:', im_count.
ENDMETHOD.
ENDCLASS.
DATA(obj) = NEW compile_time_polymorphism( ).
obj->print_message( im_message = 'Hello' ). " Calls the first method
obj->print_message( im_message = 'Hi' im_count = 3 ). " Calls the second method
Output:
Message: Hello
Message: Hi Count: 3
Explanation:
- The first method print_message is called with the parameter im_message set to ‘Hello’. It prints the message “Message: Hello.”
- The second method print_message is called with two parameters: im_message set to ‘Hi’ and im_count set to 3. It prints “Message: Hi Count: 3.”
2. Run-Time Polymorphism (Dynamic Binding) in SAP ABAP:
- Also called method overriding or late binding.
- Occurs at runtime.
- Involves the use of abstract classes and methods, allowing subclasses to provide their own implementations for abstract methods.
- The correct method to be executed is determined at runtime based on the actual type of the object.
Example:
CLASS abstract_shape DEFINITION ABSTRACT.
PUBLIC SECTION.
METHODS calculate_area ABSTRACT.
ENDCLASS.
CLASS circle DEFINITION INHERITING FROM abstract_shape.
PUBLIC SECTION.
METHODS calculate_area REDEFINITION.
ENDCLASS.
CLASS square DEFINITION INHERITING FROM abstract_shape.
PUBLIC SECTION.
METHODS calculate_area REDEFINITION.
ENDCLASS.
CLASS polymorphic_example DEFINITION.
PUBLIC SECTION.
METHODS display_area FOR EVENT handler.
ENDCLASS.
CLASS polymorphic_example IMPLEMENTATION.
METHOD display_area FOR EVENT handler.
DATA(shape) = CAST abstract_shape( handler->get_data( ) ).
shape->calculate_area( ).
ENDMETHOD.
ENDCLASS.
" Usage
DATA(event_handler) = NEW cl_gui_custom_container( ).
SET HANDLER polymorphic_example=>display_area FOR event_handler.
event_handler->set_data( NEW circle( ) ).
event_handler->fire_event_by_id( id = 1 ).
event_handler->set_data( NEW square( ) ).
event_handler->fire_event_by_id( id = 1 ).
Output:
Area Calculation for Circle
Area Calculation for Square
Explanation:
- The polymorphic_example class defines the method display_area that uses an event handler to get an object of type abstract_shape and calls its calculate_area method.
- The event_handler is set with an object of type circle, and the fire_event_by_id method is called to trigger the event. This calls the calculate_area method of the circle class, displaying “Area Calculation for Circle.”
- The event_handler is then set with an object of type square, and the fire_event_by_id method is called again. This calls the calculate_area method of the square class, displaying “Area Calculation for Square.”
Abstract Class and Subclass in SAP ABAP
In SAP ABAP, an abstract class is a class that cannot be instantiated on its own and serves as a blueprint for its subclasses. It typically contains abstract methods, which are methods without an implementation in the abstract class. Subclasses should provide strong implementations for these abstract methods.
Sample Code Example:
Let’s consider an example of an abstract class representing different shapes, with an abstract method for calculating the area. Subclasses (e.g., Circle and Square) will provide their specific implementations.
CLASS abstract_shape DEFINITION ABSTRACT.
PUBLIC SECTION.
METHODS calculate_area ABSTRACT.
ENDCLASS.
CLASS abstract_shape IMPLEMENTATION.
METHOD calculate_area.
WRITE: 'Area Calculation Not Defined'.
ENDMETHOD.
ENDCLASS.
CLASS circle DEFINITION INHERITING FROM abstract_shape.
PUBLIC SECTION.
METHODS calculate_area REDEFINITION.
ENDCLASS.
CLASS circle IMPLEMENTATION.
METHOD calculate_area.
DATA radius TYPE i VALUE 5.
DATA area TYPE i.
area = COND #( WHEN sy-index = 1 THEN 3.14 * radius * radius
ELSE 0 ).
WRITE: / 'Area of Circle:', area.
ENDMETHOD.
ENDCLASS.
CLASS square DEFINITION INHERITING FROM abstract_shape.
PUBLIC SECTION.
METHODS calculate_area REDEFINITION.
ENDCLASS.
CLASS square IMPLEMENTATION.
METHOD calculate_area.
DATA side_length TYPE i VALUE 4.
DATA area TYPE i.
area = COND #( WHEN sy-index = 1 THEN side_length * side_length
ELSE 0 ).
WRITE: / 'Area of Square:', area.
ENDMETHOD.
ENDCLASS.
Output:
DATA(shape) = NEW abstract_shape( ).
shape->calculate_area( ). " Output: Area Calculation Not Defined
shape = NEW circle( ).
shape->calculate_area( ). " Output: Area of Circle: 78.5
shape = NEW square( ).
shape->calculate_area( ). " Output: Area of Square: 16
Explanation:
- The abstract_shape class has an abstract method calculate_area without an implementation.
- Subclasses circle and square inherit from abstract_shape and provide their own implementations for calculate_area.
- Instantiating an object of type abstract_shape directly is not allowed.
- The calculate_area method dynamically calls the appropriate implementation based on the actual type of the object.
In this example, abstract classes allow for the creation of a common interface while letting subclasses define their specific behavior. The output shows how polymorphism works in the context of an abstract class in SAP ABAP.
Dynamic Type Binding in SAP ABAP
Dynamic type binding is a concept related to polymorphism in SAP ABAP, specifically in the context of method calls. It involves determining the actual type of an object at runtime and dynamically binding the appropriate method based on that type. This is crucial for achieving runtime polymorphism.
In SAP ABAP, when you have a reference variable of a superclass type pointing to an object of a subclass, the system determines the actual type of the object at runtime. Dynamic type binding allows the system to invoke the correct method based on the actual type of the object being referred to.
CLASS superclass DEFINITION.
PUBLIC SECTION.
METHODS display_data.
ENDCLASS.
CLASS subclass DEFINITION INHERITING FROM superclass.
PUBLIC SECTION.
METHODS display_data REDEFINITION.
ENDCLASS.
START-OF-SELECTION.
DATA obj TYPE REF TO superclass.
CREATE OBJECT obj TYPE subclass.
" Dynamic type binding
CALL METHOD obj->display_data( ).
Explanation:
- We have a superclass superclass with a method display_data.
- We have a subclass subclass that inherits from superclass and redefines the display_data method.
- We create an object obj of type subclass but assign it to a reference variable of type superclass.
When we call obj->display_data( ), dynamic type binding comes into play. The system recognizes that the actual type of the object referred to by obj is a subclass object. As a result, the overridden method in the subclass is invoked at runtime.
Conclusion:
Polymorphism in SAP ABAP facilitates code reuse, flexibility, and adaptability. By allowing different classes to be treated as instances of the same class, polymorphism contributes to the development of more modular and maintainable software systems. Understanding dynamic type binding is crucial for harnessing the full power of polymorphism in SAP ABAP.
Share your thoughts in the comments
Please Login to comment...