In the world of SAP ABAP (Advanced Business Application Programming), the use of Constants and Literals is necessary for the effective handling of data. Literals are used to denote specific data types such as numbers, characters, strings, and boolean values.
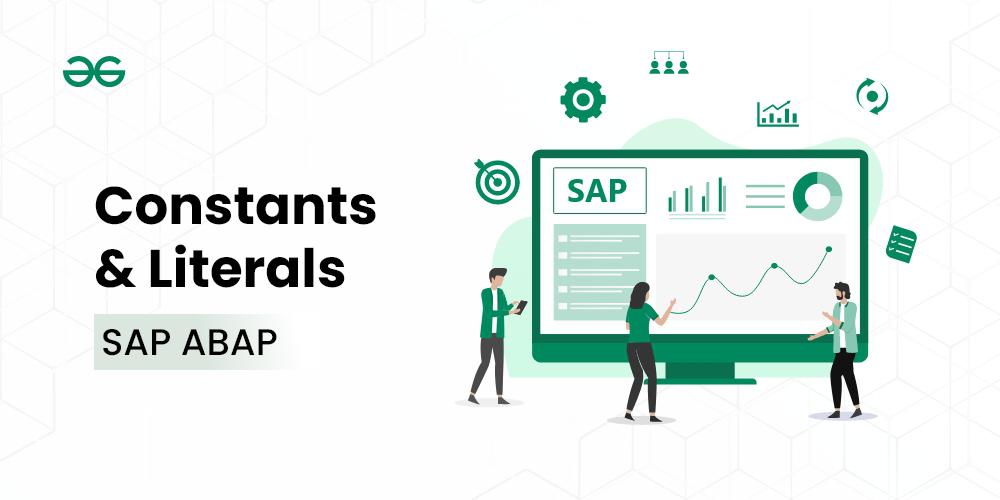
Constants & Literals in SAP
What are Literals?
Literals in programming refer to fixed values that are directly represented in the source code of a program. They are used to denote specific data types such as numbers, characters, strings, and boolean values. Literals are constant and do not change during the execution of the program.
There are 2 Types of Literals:
- Numeric literals
- Character literals
1. Numeric Literals
Numeric literals in SAP ABAP are constants that represent fixed numerical values in the program’s source code. These literals don’t need to be declared as variables or constants and can be integers (whole numbers), floating-point numbers (numbers with decimal points), and values in scientific notation. In SAP ABAP, numerical literals are used to specify numerical parameters, assign precise numerical values to variables, and carry out mathematical operations.
Note: Numeric literals value between -2147483648 (-231 + 1) and 2147483647 (232 + 1) are integer literals of data type (I). It can not have the values beyond this.
When attempting to activate the program object, an overflow error occurs if the specified numeric literal value is more than 2147483647 and less than -2147483648.
Numerical literals with a range outside of the one above are packed literals with the data type packed(p). A 16-byte numerical literal can have between 16 and 31 digits, whereas one that is 8 bytes long can accommodate up to 15 digits.
Some examples of numeric literals :
693.
+92.
-443.
Example of Overflow Condition
DATA: num TYPE i.
num = 10000000000000000000.
WRITE: num.
2. Character Literals
Character literals in SAP ABAP are important components for representing sequences of alphanumeric characters within the source code of a program. Text field literals and text string literals are the two different varieties of these literals, which are denoted by single quotation marks.
- Text Field Literals are predefined with the ABAP type ‘C’ and are identified by single quotation marks. Any trailing blanks are often disregarded since they indicate sequences of alphabetic letters. Text string literals
- Text String Literals are indicated by “back quotes” and have the ABAP type STRING. The quantity of characters is what determines the field length for both kinds.
Some examples of character literals.
Text field literals:
- They are enclosed in single quotation marks (‘ ‘).
- They have predefine ABAP data type ‘C’ .
- The field length is determined by the number of characters within the literal.
- Trailing blanks are usually ignored.
Note: The literal length of the text field must range from 1 to 255 characters.
Example of Text Field Literals
DATA name TYPE c LENGTH 10.
name = 'Duck'.
Text String literals:
- They are enclosed in back quotes(` `).
- They have Data Type ’STRING’.
- The field length is not fixed and can vary to accommodate the length of the literal.
- Trailing blanks are considered part of the string.
Note: The maximum length for a text string literal is 255 characters.
Example of String Field Literals
DATA description TYPE string.
description = `This is a string literal.`.
What are Constants?
A constant in programming is a named, unchanging value that is assigned a fixed name like a variable. The key difference is that a constant’s value, once declared, does not change during programme execution. It has a value that was assigned at declaration time, is kept in programme memory, and cannot be changed without the risk of a runtime error. Constants are ideal for representing fixed values like the mathematical constant pi (e.g., 3.14) within a program, serving as literal, unchangeable references.
CONSTANTS Statements
The CONSTANTS statement is used to declare named data objects.
Syntax for ABAP Constants:
CONSTANTS constantName TYPE dataType VALUE constantValue.
constantName: specifies a name for the constant.
dataType: represents the data type
constantValue: assigns an initial value to the declared constant name constantName.
Example of ABAP Constants:
CONSTANTS gfgID TYPE I VALUE 1.
In this ABAP example, we declare a constant named ‘gfgID’ of type ‘I’ (integer). It is assigned the value ‘1’, representing a unique identifier for a data record.
Practical Example of ABAP Constants:
A payroll application is one realistic example of using constants in SAP ABAP. Imagine a situation where you must determine an employee’s bonus depending on a number of factors, such as years of service. A constant might be defined as follows:
CONSTANTS cBonusMultiplier TYPE P DECIMALS 2 VALUE '0.05'.
In this instance, the bonus multiplier, denoted by the constant ‘cBonusMultiplier’, is 5% (0.05) of the employee’s pay. Then, you may reliably compute bonuses across the whole application by using this constant in your ABAP code. For instance:
DATA lvEmployeeSalary TYPE P DECIMALS 2.
DATA lvBonus TYPE P DECIMALS 2.
lvEmployeeSalary = 5000.00. " Example employee salary
lvBonus = lvEmployeeSalary * cBonusMultiplier.
WRITE: 'Employee Salary:', lvEmployeeSalary, 'Bonus:', lvBonus.
The bonus computation is kept consistent across the programme by utilising the constant cBonusMultiplier, and if the bonus % ever has to change, you only need to adjust it in one place (the constant declaration) rather than scouring the whole codebase. This lowers the possibility of mistakes and improves the maintainability of your code.
Type of Constants:
There are 3 types of Constants
- Elementary constants
- Complex constants
- Reference constants
1. Elementary Constants
The CONSTANTS statement is used to declare immutable data objects known as elementary constants. Any kind of data, including numeric, character-like, and date/time data, may be stored using them.
For storing quantities that are used repeatedly throughout a programme, such as pi or the speed of light, elementary constants are helpful. Additionally, they may be utilised to construct constants for certain reasons, such as the maximum number of customers allowed in a database table or the default value for a field.
Declaration of an Elementary constant for the value of pi:
CONSTANTS pi TYPE p DECIMALS 10 VALUE '3.1415926536'.
Example of Elementary Constants
DATA: radius TYPE p DECIMALS 10 VALUE 5.0.
DATA: area TYPE p DECIMALS 10.
area = pi ✖ radius ✖ radius.
WRITE: / 'The area of the circle is:', area.
2. Complex Constants
Complex constants in SAP ABAP are collections of elementary constants, such as strings or integers, put together to represent organised data, improving the readability and maintenance of the code.
Declaration of Complex constants
CONSTANTS: BEGIN OF complex-constant-name,
…
END OF complex-constant-name.
Example of Complex constants
CONSTANTS:
BEGIN OF address,
name TYPE c LENGTH 20 VALUE 'Alfred Milestone',
street TYPE c LENGTH 20 VALUE '14 Avenue',
number TYPE p VALUE 10,
postcode TYPE n LENGTH 5 VALUE 98665,
city TYPE c LENGTH 20 VALUE 'Las Vegas'
END OF address.
Input
WRITE: / address-street.
Output
14 Avenue
3. Reference Constants
A constant that references another data item is known as a reference constant in SAP ABAP. It means that while the value of the data object that a reference constant references can be modified, the value of the reference constant itself cannot.
Any type of data item, including elementary data types, structures, internal tables, and objects, can be represented using reference constants.
Declaration of Reference constants
CONSTANTS:
ref_constant TYPE REF TO object_name VALUE IS INITIAL.
Conclusion
This article goes through the basic concepts of Constants and Literals in SAP ABAP. We looked at Character Literals and Numeric Literals, the basic building blocks for expressing certain values directly in code. Our next goal was to comprehend constants and how to use them via the CONSTANTS declaration. We also looked into real-world use cases to show how Constants and Literals improve readability and flexibility of code. Finally, grasping these fundamental ideas will enable ABAP developers to produce reliable, effective, and maintainable programmes in the SAP environment.
Share your thoughts in the comments
Please Login to comment...