SAP ABAP (Advanced Business Application Programming) is a high-level programming language created by the German software company SAP SE. ABAP is primarily used for developing and customizing applications within the SAP ecosystem, which includes enterprise resource planning (ERP) systems and other business software solutions. C++ is used to implement the ABAP kernel. A procedural and object-oriented programming model are both supported by the hybrid programming language ABAP.
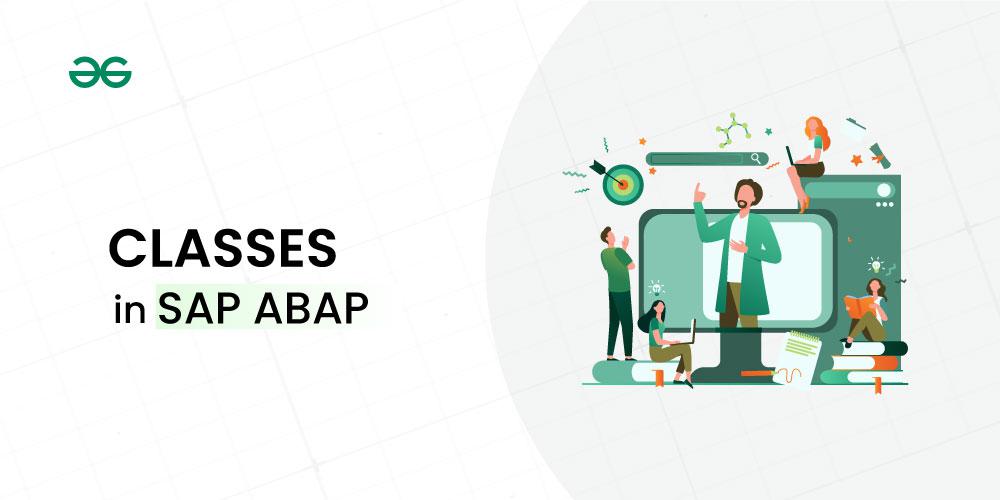
Classes in SAP ABAP
Introduction to Classes in SAP ABAP
A class is a blueprint or template in SAP ABAP, a class defines the properties and behavior of objects. it encapsulates data – including the methods that act on this data. This encapsulation allows for modular code structures. reusable entities can be created with ease. Implementing various Object-Oriented Programming (OOP) concepts in SAP ABAP such as encapsulation, inheritance, and polymorphism on classes functioning as their foundation. Developers can create complex, structured applications through their use of these tools. this process not only facilitates superior code management but also enhances reusability.
Syntax for defining a class in SAP ABAP
Various sections public, protected, and private constitute the syntax for defining a class in SAP ABAP; within these sections, attributes and methods declare their respective visibility. The general syntax is as follows:
CLASS <class_name> DEFINITION.
PUBLIC SECTION.
" Define public attributes and methods.
PROTECTED SECTION.
" Define protected attributes and methods.
PRIVATE SECTION.
" Define private attributes and methods.
ENDCLASS.
- <class_name>: This is the name of the class being defined. It should be unique and should follow SAP ABAP naming conventions.
- PUBLIC SECTION: section contains attributes and methods that can be accessed from outside the class.
- PROTECTED SECTION: Attributes and methods declared in this section are accessible within the class and its subclasses.
- PRIVATE SECTION: section includes attributes and methods that are only accessible within the class itself.
Syntax for implementing a class in SAP ABAP
After defining the class, one must implement it to actual logic and functionality upon declared attributes and methods.The implementation section is where the methods are defined and the logic for the behavior of the class is written. Below is the basic syntax for implementing a class:
CLASS <class_name> IMPLEMENTATION.
" Implement methods and logic here.
ENDCLASS.
- <class_name>: should match the name of the class that was defined earlier.
- IMPLEMENTATION: signifies the beginning of the implementation section for the specified class.
- ENDCLASS: signifies the end of the implementation section for the class.
Attributes in SAP ABAP Classes
Within a class in SAP ABAP, attributes serve as variables, they hold data and state information. We can classify these attributes into two types:
- instance attributes
- static attributes.
Instance Attributes in SAP ABAP
Specific to each object or instance of the class, instance attributes uniquely hold values for every object instance, thus permitting diverse objects to preserve separate states. When creating an object of the class, initialization occurs automatically enabling access and modification through its respective object instance, this is where these unique characteristics become operative.
CLASS lcl_class_definition DEFINITION.
PUBLIC SECTION.
DATA: instance_attribute TYPE data_type.
ENDCLASS.
In the example above, instance_attribute is an instance attribute that holds a specific value for each object of the class lcl_class_definition.
Static Attributes in SAP ABAP
All instances of the class share static attributes. Throughout the entire execution of the program, these attributes maintain their value and any modifications made to them impact all instances simultaneously. Sharing a value across all class instances is particularly useful for these specific attributes.
CLASS lcl_class_definition DEFINITION.
PUBLIC SECTION.
CLASS-DATA static_attribute TYPE data_type.
ENDCLASS.
In this case, static_attribute is a static attribute that is shared among all instances of the class lcl_class_definition.
Methods in SAP ABAP Classes
Methods in SAP ABAP represent the functions or procedures a class defines to execute specific actions or operations. They manipulate data and execute various functionalities by accessing the class attributes. Therefore, it is crucial to comprehend method definition within a class and attribute/method access for effective implementation of desired behavior in that particular class.
Defining Methods in SAP ABAP Classes:
In SAP ABAP, methods are declared within the class definition and implemented in the implementation section.
CLASS <class_name> DEFINITION.
PUBLIC SECTION.
METHODS method_name.
ENDCLASS.
CLASS <class_name> IMPLEMENTATION.
METHOD method_name.
" Method logic goes here.
ENDMETHOD.
ENDCLASS.
- <class_name>: Represents the name of the class.
- method_name: Refers to the name of the method being defined.
Accessing Attributes and Methods in SAP ABAP:
An object instance of a class allows access to its attributes and methods specifically, we use the arrow operator (->) for this purpose. Consider this illustration:
DATA: obj TYPE REF TO <class_name>.
obj = NEW #( ). " Create an instance of the class.
obj->method_name( ). " Call the method.
WRITE: obj->attribute_name. " Access the attribute.
- <class_name>: name of the class.
- obj: object instance of the class.
- method_name: method being called.
- attribute_name: attribute being accessed.
Static Attributes in SAP ABAP
In SAP ABAP, class-level attributes share among all instances of the class are known as static attributes. Throughout the program execution, they maintain their values, this proves particularly useful for globally accessible storing data within the class.
Declaring Static Attributes in SAP ABAP:
In SAP ABAP, static attributes are declared within the class definition using the CLASS-DATA statement.
CLASS <class_name> DEFINITION.
PUBLIC SECTION.
CLASS-DATA static_attribute TYPE data_type.
ENDCLASS.
- <class_name>: name of the class.
- static_attribute: name of the static attribute being declared.
- data_type: data type of the static attribute.
Accessing Static Attributes in SAP ABAP:
Static attributes can be accessed directly using the class name and the => operator.
<CLASS_NAME>=><ATTRIBUTE_NAME>.
- <CLASS_NAME>: name of the class.
- <ATTRIBUTE_NAME>: name of the static attribute being accessed.
Constructors in SAP ABAP
A constructor, as a special method within a class in SAP ABAP, triggers automatically upon creation of an object. In essence, a constructor is responsible for allocating memory space for an object; it sets initial values–either user-defined or system defaults–to instance variables within that object.
Role of Constructors in SAP ABAP:
Constructors primarily undertake the essential setup or initialization tasks upon object instantiation. They initialize the attributes of an object to either default values, or values passed during its creation as parameters. By ensuring proper configuration and readiness for use, constructors avert any subsequent operations from yielding unexpected behavior or errors.
Triggering Constructors in SAP ABAP:
In SAP ABAP, constructors are automatically triggered when an object is instantiated using the CREATE OBJECT statement.
DATA: obj TYPE REF TO <class_name>.
CREATE OBJECT obj.
- <class_name>: ame of the class.
- obj: object instance of the class being created.
ME operator in SAP ABAP methods
Within SAP ABAP, methods utilize the ME operator to reference the current instance of a class. This facilitates method access and manipulation of class attributes and other methods without necessitating an explicit reference to any specific object instance.
Using ME Operator to access class attributes:
The ME operator, within a method of a class, facilitates direct reference to the current instance of that particular class. This functionality permits unfettered accessibility – sans any external object reference – to both attributes and methods belonging to said class.
CLASS <class_name> DEFINITION.
PUBLIC SECTION.
DATA: attribute_name TYPE data_type.
METHODS: method_name.
ENDCLASS.
CLASS <class_name> IMPLEMENTATION.
METHOD method_name.
" Accessing class attribute using ME operator
ME->attribute_name = value. " Modifying the attribute
ENDMETHOD.
ENDCLASS.
- <class_name>: name of the class.
- attribute_name: name of the class attribute being accessed.
- value: value being assigned to the attribute.
Examples of Classes in SAP ABAP
Here are two sample programs that illustrate the usage of classes in SAP ABAP.
Program 1: A Simple Class and Object Creation
REPORT demo_class_usage.
CLASS demo_class DEFINITION.
PUBLIC SECTION.
METHODS:
display_name.
PRIVATE SECTION.
DATA:
name TYPE string.
ENDCLASS.
CLASS demo_class IMPLEMENTATION.
METHOD display_name.
WRITE: / 'Name:', name.
ENDMETHOD.
ENDCLASS.
START-OF-SELECTION.
DATA: obj TYPE REF TO demo_class.
CREATE OBJECT obj.
obj->name = 'John Doe'.
obj->display_name( ).
Output :
Name: John Doe
Program 2: Using Static Attribute and Method
REPORT demo_static_class.
CLASS demo_static_class DEFINITION.
PUBLIC SECTION.
CLASS-DATA: counter TYPE i.
CLASS-METHODS: increment_counter, display_counter.
ENDCLASS.
CLASS demo_static_class IMPLEMENTATION.
METHOD increment_counter.
counter = counter + 1.
ENDMETHOD.
METHOD display_counter.
WRITE: / 'Counter:', counter.
ENDMETHOD.
ENDCLASS.
START-OF-SELECTION.
demo_static_class=>increment_counter( ).
demo_static_class=>display_counter( ).
Output :
Counter: 1
Conclusion :
Concluding, implementing modular and scalable applications, leveraging OOP principles require integral SAP ABAP classes. These classes facilitate encapsulation, inheritance and polymorphism to enhance code reusability and maintenance. Developers can secure data handling by defining attributes, methods and visibility sections effectively. Developers can create ef ficient, adaptable, and robust solutions within the SAP ecosystem by leveraging static attributes and constructors to streamline data management and object initialization.
Share your thoughts in the comments
Please Login to comment...