High-level programming languages like SAP ABAP (Advanced Business Application Programming) are used to create apps in the SAP environment. Operators are essential for the execution of many different operations in SAP ABAP, ranging from straightforward arithmetic computations to complex logical analyses. This article will examine the various SAP ABAP operator types and offer examples to show how to use them.
SAP ABAP Operators
In SAP ABAP, operators are symbols or special characters that apply particular operations on operands. Variables, constants, or expressions can all be considered operands. Numerous operator types are supported by SAP ABAP, such as bitwise, arithmetic, comparison, automated type adjustment, and character string operators.
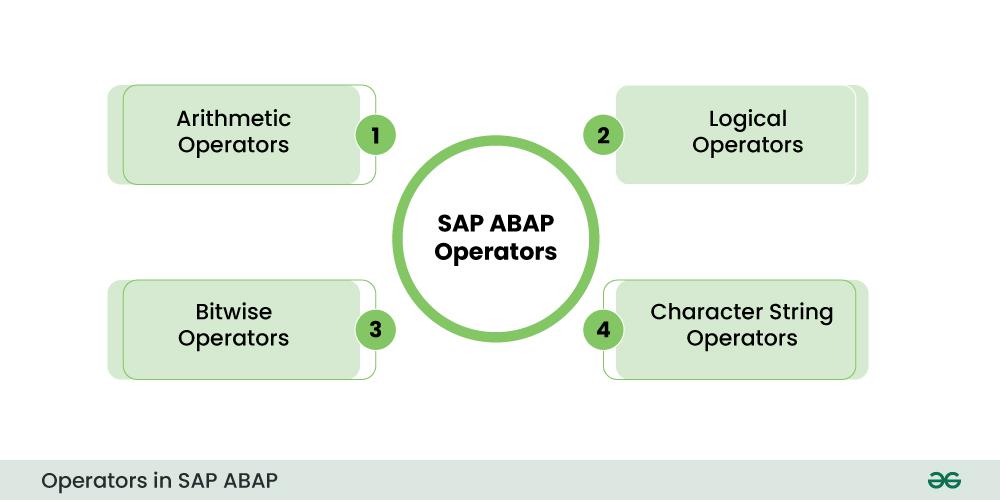
Operators in SAP ABAP
Arithmetic Operators in SAP ABAP
Arithmetic Operators are used to perform basic operations in mathematics that are used in algebra, Here we are sharing you a list of arithmetic operators in SAP ABAP. Basic mathematical operations in SAP ABAP are carried out using arithmetic operators. Addition (+), subtraction (-), multiplication (*), division (/), and modulus (MOD) are some of these operators. Here we are sharing you a table in which every arithmetic operator is discussed in detail.
Adds the operands
|
From left to Right
|
Subtract the operands
|
From left to Right
|
Multiply the operands
|
From left to Right
|
Divide the left operand by the right operand
|
From left to Right
|
integer parts after the division of the left operand by the right operand
|
From left to Right
|
Positive remainder of the division of the left operand by the right;
|
From left to Right
|
Raises the left Operand to the right Operand
|
From Right to left
|
Let’s examine an example:
DATA: num1 TYPE I VALUE 10,
num2 TYPE I VALUE 5,
result TYPE I.
result = num1 + num2. " Addition
result = num1 - num2. " Subtraction
result = num1 * num2. " Multiplication
result = num1 / num2. " Division
result = num1 MOD num2. " Modulus
Output:
15
5
50
2
0
Comparison operators in SAP ABAP are utilized to compare data and offer conclusions depending on the comparison’s results. These operators include equal to (EQ), not equal to (NE), less than (LT), less than or equal to(LT) , greater than(GT) , and greater than or equal to (GE). Here we are sharing you a table in which every comparison operator is discussed in detail.
returns true If two operands are equal to each other
|
returns true If two operands are not equal to each other
|
returns true If first operand is less than second.
|
returns true If first operand is greater than second.
|
returns true If first operand is less than or equal to second.
|
returns true If first operand is greater than or equal to second.
|
returns true if the variable declared is not modified
|
returns true if the variable declared is modified
|
returns true if a lies in between b and c
|
Here’s an example:
DATA: value1 TYPE I VALUE 10,
value2 TYPE I VALUE 20,
result TYPE C LENGTH 1.
IF value1 EQ value2.
result = 'X'. " True
ELSE.
result = ' '. " False
ENDIF.4
Output:
False
Bitwise Operators in SAP ABAP
In SAP ABAP, bitwise operators are used to manipulate data at the bit level. Bitwise AND (&), bitwise OR (|), bitwise XOR (^), and bitwise NOT (~) are some examples of these operators. In ABAP, bitwise operators can be helpful in some situations and are frequently utilized in low-level programming.
Here we are sharing you a table in which every Bitwise operator is discussed in detail.
1
|
0
|
0
|
0
|
0
|
1
|
1
|
0
|
1
|
1
|
0
|
0
|
0
|
1
|
1
|
0
|
1
|
1
|
1
|
0
|
The bitwise AND operator is used in the following example:
DATA: num1 TYPE I VALUE 10,
num2 TYPE I VALUE 7,
result TYPE I.
result = num1 & num2. " Bitwise AND
Character String in SAP ABAP
Here we are sharing you a table in which every Character string operator is explained:
returns true if A consists of only the characters in B.
|
returns true if A consists contains at least one character not present in B.
|
returns true if A contains at least one character of B.
|
returns true if A does not contain any character present in B
|
returns true if A contains the entire string B
|
returns true if A does not contains the entire string B
|
returns true if A contains the pattern in B
|
returns true if A does not contain the pattern in B
|
Example:
. DATA: P(10) TYPE C VALUE 'GFG',
Q(10) TYPE C VALUE 'GEEKS'.
IF P CA Q.
WRITE: / 'P contains at least one character of Q'.
ENDIF.
Output:
P contains at least one character of Q
Automatic Type Adjustment in SAP ABAP
The data type of an operand can be automatically changed during an operation in SAP ABAP using automated type adjustment operators. For example, you can concatenate strings and add numeric values with the ‘+’ symbol. Here’s one example:
DATA: num TYPE I VALUE 10,
text TYPE STRING VALUE '20',
result TYPE STRING.
result = num + text. " Automatic type adjustment: Result is '1020'
String Operators in SAP ABAP
In SAP ABAP, strings are worked with using character string operators. These operators include pattern matching (contains, find, find regex), substring (substring, OFFSET), and concatenation (concatenate, &&).
This is an example of concatenation:
DATA: first_name TYPE STRING VALUE 'John',
last_name TYPE STRING VALUE 'Doe',
full_name TYPE STRING.
full_name = first_name && last_name. " Concatenation
Output:
John Doe
FAQS on Operators in SAP ABAP:
1. What are logical operators in SAP ABAP?
Answer: Logical Operators are used in SAP ABAP for comparing the data. these are also known as conditional or relational operators. These operators include equal to (EQ), not equal to (NE), less than (LT), less than or equal to(LE) , greater than(GT) , and greater than or equal to (GE). Comparison operators in SAP ABAP are utilized to compare data and offer conclusions depending on the comparison’s results. Please refer the above mentioned table for more details.
2. What are Reduce Operator in SAP ABAP?
Answer: Reduce Operator in SAP ABAP is used to reduce a list of objects. it is generally used to reduce sets of data objects to a single data objects.
3. What are VALUE Operator in SAP ABAP?
Answer: VALUE operator in SAP ABAP is used to initialize the variables of any non-generic data types. It can also controls the data type of a variable. we can also use VALUE operator to initailze complex data objects(structures or internal tables.)
4. Whar are filter Operator in SAP ABAP?
Answer: Filter Operator in SAP ABAP is used to select particular data from a large dataset using some specific WHERE clause. it works similar to VALUE Operator , means for non -generic data types, that means data types should be defined before used.
5. What are Ternary Operator in SAP ABAP?
Answer: There is no any Ternary Operator in SAP ABAP , but we have an alternative for doing this operation. by using the below syntax we can achieve this operation:
COND type( [let_exp]
WHEN log_exp1 THEN [ let_exp] result1
[ WHEN log_exp2 THEN [ let_exp] result2 ]
...
[ ELSE [ let_exp] resultn ] ) ...
Conclusion
For a variety of tasks in SAP ABAP, from basic arithmetic computations to data manipulations, operators are necessary. For developers working with SAP ABAP, comprehending these operators is essential to effectively implementing business logic and data processing within the SAP ecosystem. An overview of the various SAP ABAP operator types has been given in this article, along with usage examples. Learning how to use these operators will be an important programming skill as you work with SAP ABAP further.
Share your thoughts in the comments
Please Login to comment...