Routes Component in React Router
Last Updated :
08 Mar, 2024
Routes are an integral part of React Router, facilitating the navigation and rendering of components based on URL patterns.
In this article, we’ll delve into the concept of routes, understanding their role in React Router, and how to define and manage routes effectively.
What are Routes ?
In the context of React Router, routes define the mapping between URL patterns and the corresponding React components to render. They serve as the backbone of client-side navigation in single-page applications (SPAs), allowing users to navigate between different views without full-page reloads.
Why Routes Are Essential ?
Routes play a crucial role in building dynamic and interactive web applications. Here’s why they are essential:
- URL-Based Navigation: Routes enable navigation based on URLs, providing users with a familiar browsing experience.
- Component Rendering: By mapping URLs to specific components, routes determine which components to render based on the current URL.
- Dynamic Content: Routes facilitate the rendering of different content or views based on the user’s navigation, enhancing the overall user experience.
- Nested Routing: React Router supports nested routes, allowing for the creation of complex navigation hierarchies and multi-level layouts within an application.
Defining Routes:
In React Router, routes are typically defined using the Route component. Here’s the basic structure of a route definition:
<Route path="/example" component={ExampleComponent} />
- path: Specifies the URL pattern that the route should match.
- component: Specifies the React component to render when the URL matches the defined path.
Managing Routes
React Router provides various components and utilities for managing routes effectively:
- Router: The top-level component that wraps the application and provides routing context.
- Switch: Renders the first matching Route or Redirect within its children, ensuring that only one route is rendered at a time.
- Link: A component for declarative navigation, allowing users to navigate to different routes by clicking on links.
- Redirect: Redirects users from one route to another based on predefined conditions.
Steps to implement Routes Component:
Step 1: Create react application with the help of following command.
npx create-react-app react-router
cd react-router
Step 2: Install the required dependencies.
npm I react-router-dom
Folder Structure:
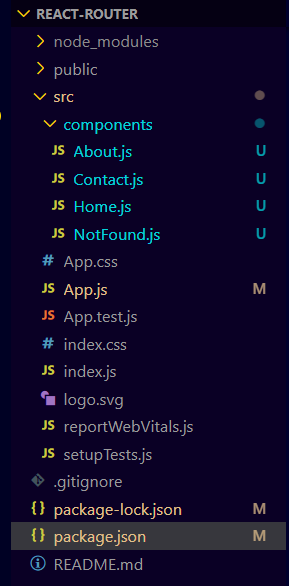
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.1",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example of Routes Component
Code: Let’s consider an example to demonstrate the usage of routes in React Router:
Javascript
import React from 'react' ;
import {
BrowserRouter as Router,
Route, Routes
} from 'react-router-dom' ;
import Home from './components/Home' ;
import About from './components/About' ;
import Contact from './components/Contact' ;
import NotFound from './components/NotFound' ;
function App() {
return (
<Router>
<div>
<Routes>
<Route path= "/" element={<Home />} />
<Route path= "/about" element={<About />} />
<Route path= "/contact" element={<Contact />} />
<Route path= "*" element={<NotFound />} />
</Routes>
</div>
</Router>
);
}
export default App;
|
Javascript
import React from 'react'
const Home = () => {
return (
<h1>Home</h1>
)
}
export default Home
|
Javascript
import React from 'react'
const Contact = () => {
return (
<h1>Contact</h1>
)
}
export default Contact
|
Javascript
import React from 'react'
const About = () => {
return (
<h1>About</h1>
)
}
export default About
|
Javascript
import React from 'react'
const NotFound = () => {
return (
<h1>NotFound</h1>
)
}
export default NotFound
|
Output:
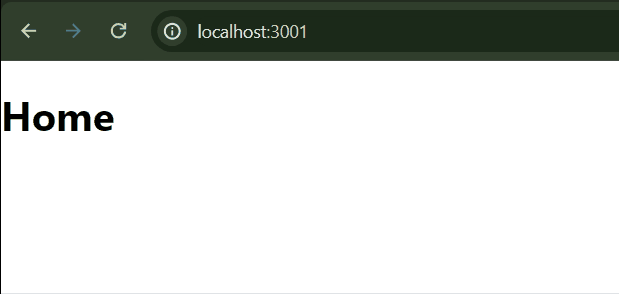
Explanation of Output:
- We define routes for the Home, About, and Contact components using the Route component.
- The Switch component ensures that only one route is rendered at a time, preventing multiple routes from matching.
- If none of the defined routes match the current URL, the NotFound component is rendered.
Conclusion:
Routes are the backbone of client-side navigation in React Router, providing a powerful mechanism for defining URL-based navigation and rendering. Understanding how to define and manage routes effectively is essential for building dynamic and interactive React applications with seamless navigation capabilities.
Share your thoughts in the comments
Please Login to comment...