Explain Nested Routes in React
Last Updated :
05 Mar, 2024
Nested Routes are a type of routing in which one route is linked to another route. To set up routing on the client side in the React project and to enable navigation in our project, we will use the React Router library. React Router is a powerful library that helps in creating single-page applications (SPAs). In this article, we will be creating some routes of an e-commerce app to understand nested routes properly. So first of all let’s set up our react project and prepare the project’s basic UI.
Steps to set up React project with React Router:
Step 1: Create a react project folder, open the terminal, and write the following command.
# Run this to use npm
npx create-react-app foldername
# Or run this to use yarn
yarn create react-app foldername
Step 2: Navigate to the root directory of your project using the following command.
cd foldername
Step 3: Now, install the React Router library
npm install react-router-dom
or
yarn add react-router-dom
Project Structure:
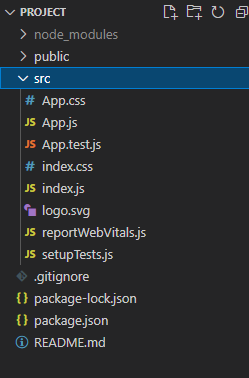
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
"react-router-dom": "^6.22.0"
}
Using of React Router v6:
Now we have all files set we just need to set up routing for the project. For this article, we are using React Router v6. So, we can create a route with a new React-Router
import { createBrowserRouter } from "react-router-dom";
const router = createBrowserRouter([
{
path: "/",
element: <App />
}
]);
But in our project category_id and product_id can be varied for each category and product, in this case, dynamic routes come in handy. No need to do much, just use the ‘:’ colon in front of the variable path name, for our project it will be something like this.
"/categories/:category_id" - Category Page
"/categories/:category_id/products/:product_id" - Product Page
1. Understanding “/”:
When nested routes are created, do not put “/” in the path of children routes, because React Router will assume the route from the very beginning, and by not putting “/” they will remain children of the parent.
Something like this
with "/"
"/:category_id" - considered as - "https://localhost:300/category_id"
without "/"
":category_id" - considered as - "https://localhost:300/category/category_id"
This is a hook given by React Router. useNavigate returns a function, and using this function we can navigate anywhere. You just need to enter the path where you want to navigate while invoking navigate function.
To get the ID from the URL in the webpage, we can use another hook provided by React Router which is useParams. useParams returns the id as an object with the same name passed while creating the router.
Now it’s time to revise what we have studied so far.
- Nested Routes are a type of routing in which one route is linked to another route.
- We can create a router using createBrowserRouter by passing an array of objects.
- Then we see the importance of “/ ” and two important hooks you must know while using React Router.
Explanation: We will create a simple e-commerce website in which there will be many categories on the home page and there will be many products in each category. On clicking on any category, all the products of that category will be shown, and on clicking on every product the product page will open.
"/" - Home page [All Categories]
"/categories/category_id" - Category Page
"/categories/category_id/products/product_id" - Product Page
Example: Below is an example of using next routes using react router v6.
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
import Home from "./Home" ;
import {
RouterProvider,
createBrowserRouter
} from "react-router-dom" ;
import CategoryPage from "./CategoryPage" ;
import ProductPage from "./ProductPage" ;
const router = createBrowserRouter([
{
path: "/" ,
element: <Home />,
children: [
{
path: "category/:category_id" ,
element: <CategoryPage />,
},
{
path: "category/:category_id/product/:product_id" ,
element: <ProductPage />,
},
],
},
]);
ReactDOM.createRoot(document.getElementById( "root" )).render(
<RouterProvider router={router}></RouterProvider>
);
|
Javascript
import {
Link,
Outlet,
useNavigate
} from "react-router-dom" ;
const Categories = [
{ label: "Fashion" , path: "fashion" },
{ label: "Electronics" , path: "electronics" },
{ label: "Sports & Fitness" , path: "sports-n-fitness" },
{ label: "Health & Beauty" , path: "health-n-beauty" },
{ label: "Books & Media" , path: "books-n-media" },
];
const Home = () => {
const navigate = useNavigate();
return (
<div>
<h1>Categories</h1>
<ul>
{Categories.map((category) => (
<li key={category.path}>
<p>{category.label}</p>
<button
onClick={() =>
navigate( "category/" + category.path)}>
View all Products
</button>
</li>
))}
</ul>
<Outlet />
</div>
);
};
export default Home;
|
Javascript
import { Link, useParams } from "react-router-dom" ;
const products = [
{ label: "Product 1" , path: "product-1" },
{ label: "Product 2" , path: "product-2" },
{ label: "Product 3" , path: "product-3" },
{ label: "Product 4" , path: "product-4" },
];
const CategoryPage = () => {
const { category_id } = useParams();
return (
<div>
<h2>Products belongs to: {category_id}</h2>
<ul>
{products.map((product) => (
<li key={product.path}>
<Link to={`/category/${category_id}/product/${product.path}`}>
{product.label}
</Link>
</li>
))}
</ul>
</div>
);
};
export default CategoryPage;
|
Javascript
import { useParams } from "react-router-dom"
const ProductPage = () => {
const { category_id, product_id } = useParams();
return (
<p>Category id:
{category_id},
Product id: {product_id}
</p>
)
}
export default ProductPage
|
Start your application using following command.
npm start
or
yarn start
Output:
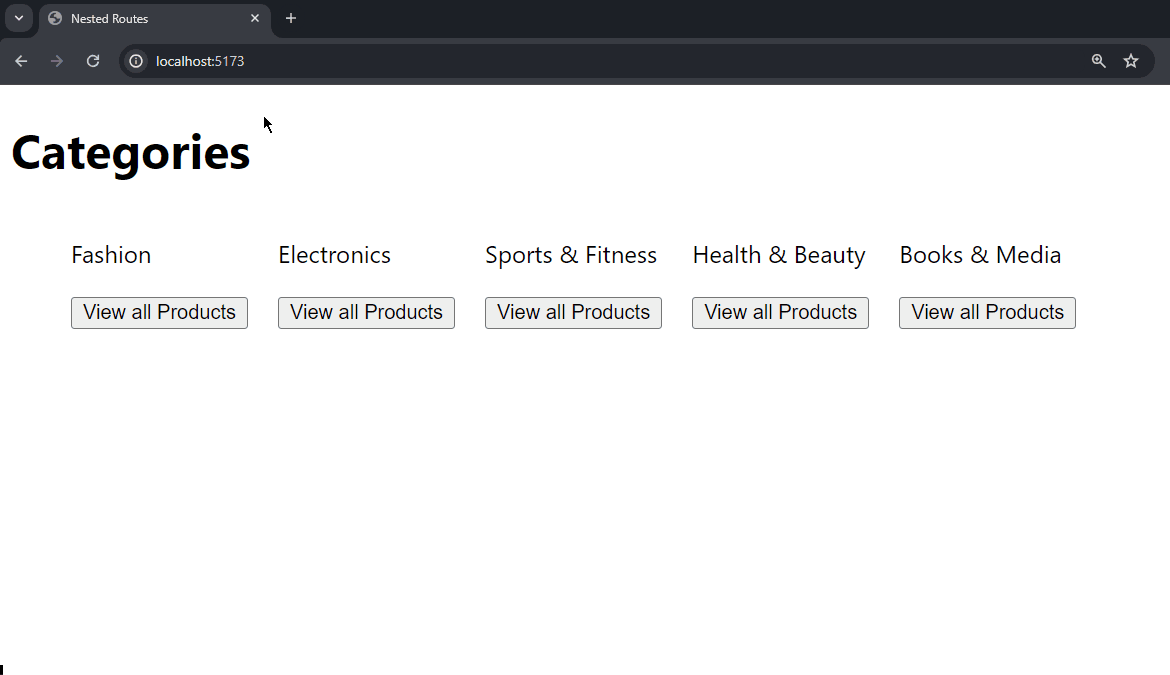
Output
Share your thoughts in the comments
Please Login to comment...