Route Component in React Router
Last Updated :
08 Mar, 2024
React Router is a popular library used for managing routing in React applications. At its core lies the Route component, which plays a pivotal role in defining the relationship between URLs and corresponding components.
In this article, we’ll delve into the intricacies of the Route component, exploring its purpose, syntax, and practical usage.
What is the Route Component?
The Route component in React Router serves as a declarative way to render UI components based on the URL. It allows developers to specify which component to render when the application’s URL matches a certain path. Essentially, the Route acts as a bridge between the URL and the UI, enabling dynamic content rendering based on the user’s navigation.
Why Use the Route Component?
The Route component provides several key benefits:
- Declarative Routing: Route allows developers to define routing logic declaratively, making it easier to understand and manage application navigation.
- Dynamic Content Rendering: By mapping URLs to specific components, Route enables the rendering of different UI elements based on the current URL, facilitating a more interactive user experience.
- Nested Routing: Route supports nested routing, allowing for the creation of hierarchical URL structures and complex routing configurations.
- Integration with React Ecosystem: Route seamlessly integrates with other React components and libraries, enabling the creation of sophisticated single-page applications (SPAs) with ease.
Structure (Syntax) of Route Component
The syntax of the Route component is straightforward and follows a predefined structure:
<Route path="/example" element={<ExampleComponent/>} />
- path: Specifies the URL pattern that the route should match. When the current URL matches this path, the associated component will be rendered.
- component: Specifies the React component to render when the URL matches the defined path.
Breakdown of the Route Component in React Router:
- Path Matching: The Route component specifies a path prop, which defines the URL pattern that the route should match. When the current URL matches the specified path, the associated component will be rendered.
- Component Rendering: The Route component renders the specified component when the URL matches the defined path. It takes a component prop or a render prop to determine what to render. The component prop accepts a React component, while the render prop accepts a function that returns a React element.
- Route Props: The Route component passes three props to the rendered component:
- match: Contains information about how the current URL matches the route path, including URL parameters and whether the route is exact.
- location: Contains information about the current URL location.
- history: Provides methods for programmatic navigation and interacting with the browser history.
- Exact Matching: By default, Route components perform partial matching of paths. If a route’s path is a prefix of the URL, the route will match. To require an exact match, you can use the exact prop. This ensures that the route is rendered only when the URL matches the path exactly.
- Nested Routes: Route components can be nested within each other to create nested routing structures. This allows for more complex routing configurations where different components are rendered based on nested URL paths.
- Dynamic Routes: Route components support dynamic URL segments using parameters specified within the path using a colon : followed by the parameter name. For example, /users/:id would match any URL with a path /users/ followed by a dynamic segment representing the user’s ID.
Steps to Implement Route Component:
Step 1: Create react application with the help of following command.
npx create-react-app react-router
cd react-router
Step 2: Install the required dependencies.
npm i react-router-dom
Folder Structure:
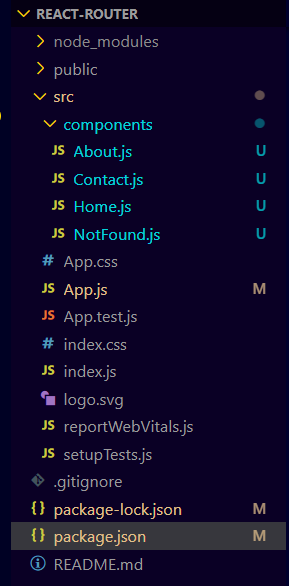
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.1",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example of Route Component
Code: Let’s consider a simple example to illustrate the usage of the Route component in React Router:
Javascript
import React from 'react' ;
import {
BrowserRouter as Router,
Route, Routes
} from 'react-router-dom' ;
import Home from './components/Home' ;
import About from './components/About' ;
import Contact from './components/Contact' ;
import NotFound from './components/NotFound' ;
function App() {
return (
<Router>
<div>
<Routes>
<Route path= "/" element={<Home />} />
<Route path= "/about" element={<About />} />
<Route path= "/contact" element={<Contact />} />
<Route path= "*" element={<NotFound />} />
</Routes>
</div>
</Router>
);
}
export default App;
|
Javascript
import React from 'react'
const Home = () => {
return (
<h1>Home</h1>
)
}
export default Home
|
Javascript
import React from 'react'
const About = () => {
return (
<h1>About</h1>
)
}
export default About
|
Javascript
import React from 'react'
const Contact = () => {
return (
<h1>Contact</h1>
)
}
export default Contact
|
Javascript
import React from 'react'
const NotFound = () => {
return (
<h1>NotFound</h1>
)
}
export default NotFound
|
Output:
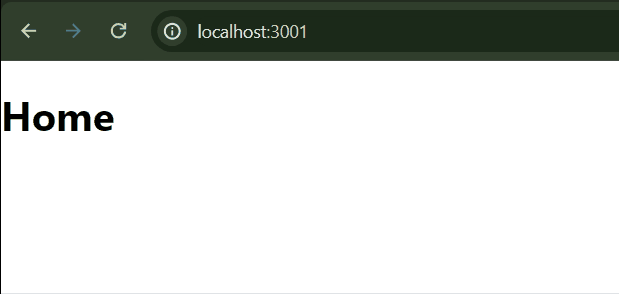
output
Explanation of Output:
- We import the Route component from ‘react-router-dom’.
- Inside the Router component, we define three routes using Route.
- Each Route specifies a path and the corresponding component to render when the URL matches that path.
Conclusion:
The Route component in React Router is a fundamental tool for managing routing in React applications. By providing a declarative approach to defining URL-based navigation, Route empowers developers to create dynamic and interactive user experiences. Understanding the syntax and usage of the Route component is essential for building robust and navigable React applications.
Share your thoughts in the comments
Please Login to comment...