What are Protected Routes in React JS ?
Last Updated :
15 Mar, 2024
In web development, security is critical when building React applications, especially those handling sensitive data or functionalities, it’s crucial to restrict access to certain parts of the application to authorized users only. This is where protected routes come into play.
In this article, we will learn in detail about Protected Routes in React Router.
Prerequisites:
What are Protected Routes ?
Protected routes are routes within a React application that require authentication or authorization before allowing access to specific components or views. Let’s take a closer look at the syntax and explanation of implementing protected routes using React Router:
const PrivateRoute = ({ component: Component, isAuthenticated, ...rest }) => (
<Route
{...rest}
render={(props) => (
isAuthenticated ?
<Component {...props} /> : <Redirect to="/login" />
)}
/>
);
Authentication and Authorization
At the core of protected routes lie two key concepts: authentication and authorization.
- Authentication: Authentication is the process of verifying the identity of users attempting to access the application. This is commonly achieved through mechanisms such as username-password authentication, social login (e.g., OAuth), or multi-factor authentication (MFA). Upon successful authentication, users are granted a session token or a cookie, which signifies their authenticated state.
- Authorization: Authorization determines whether authenticated users have the necessary permissions to access specific resources or perform certain actions within the application. Authorization mechanisms typically involve roles, permissions, or access control lists (ACLs). Administrators can define access policies dictating which users or groups are authorized to access particular routes or functionalities.
Features of Protected Routes:
- Authentication Control: Protected routes offer authentication control, ensuring that only authenticated users can access certain parts of the application.
- Redirection: Unauthorized users attempting to access protected routes are automatically redirected to designated pages, such as a login page.
- Customization: Developers have the flexibility to customize the authentication logic and redirection behavior according to the specific requirements of the application.
- Granular Access Control: Protected routes enable granular access control, allowing developers to define which users or user roles have access to specific routes or components.
- Error Handling: They provide built-in error handling mechanisms to handle authentication failures and ensure a smooth user experience.
Steps to Implement Protected Routes:
Step 1: Create the react application by the following command.
npx create-react-app protected-router
Step 2: Install the required dependencies
npm i react-router-dom
Folder Structure:
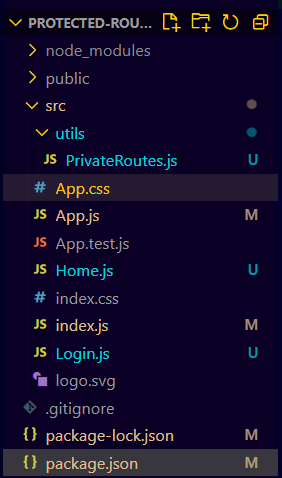
package.json:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.3",
"web-vitals": "^2.1.4"
}
Example: Create the required files as seen on the folder structure and add the following codes.
JavaScript
//App.js
import {
BrowserRouter as Router,
Routes, Route
} from 'react-router-dom'
import Home from './Home'
import Login from './Login'
import PrivateRoutes from './utils/PrivateRoutes'
function App() {
return (
<div className="App">
<Router>
<Routes>
<Route element={<PrivateRoutes />}>
<Route element={<Home />}
path="/" exact />
</Route>
<Route element={<Login />}
path="/login" />
</Routes>
</Router>
</div>
);
}
export default App;
JavaScript
//utils/PrivateRoutes.js
import {
Outlet,
Navigate
} from 'react-router-dom'
const PrivateRoutes = () => {
let auth = { 'token': false }
return (
auth.token ?
<Outlet /> :
<Navigate to="/login" />
)
}
export default PrivateRoutes
JavaScript
//Home.js
import React from 'react'
const Home = () => {
return (
<h1>Home</h1>
)
}
export default Home
JavaScript
//Login.js
import React from 'react'
const Login = () => {
return (
<h1>Login</h1>
)
}
export default Login
To start the applicrion run the following command.
npm start
Output:
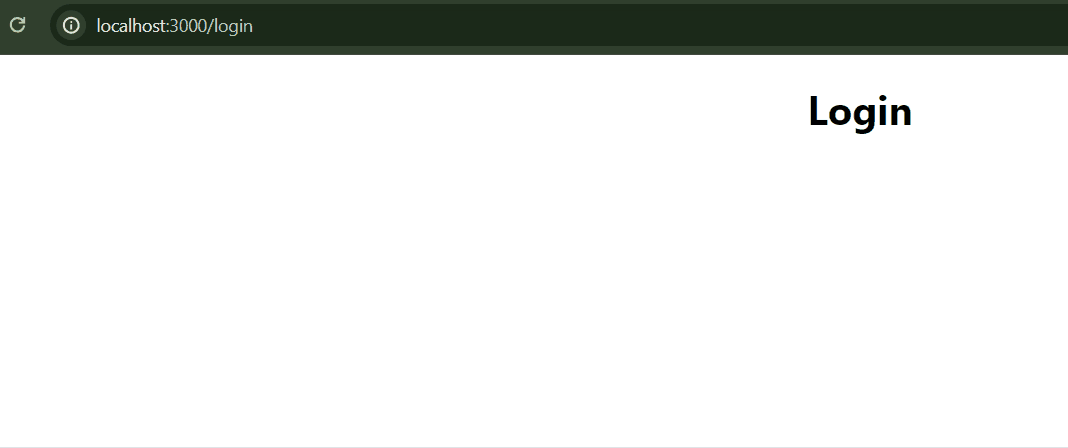
Benefits of Protected Routes:
- Enhanced Security: By restricting access to sensitive areas of the application, protected routes enhance security and mitigate the risk of unauthorized access or data breaches.
- Improved User Experience: Redirecting unauthorized users to appropriate pages, such as a login or access denied page, improves the overall user experience by providing clear feedback and guidance.
- Regulatory Compliance: Protected routes help ensure compliance with regulatory requirements, such as data protection laws, by enforcing access controls and protecting sensitive data.
- Simplified Development: Implementing protected routes using frameworks like React Router simplifies development by providing a standardized approach to access control and authentication.
- Scalability and Maintainability: As applications grow and evolve, protected routes facilitate scalability and maintainability by providing a structured mechanism for managing access control logic and handling authentication-related tasks.
Share your thoughts in the comments
Please Login to comment...