Link Component in React Router
Last Updated :
05 Mar, 2024
React Router is a powerful library in ReactJS that is used to create SPA (single-page applications) seamlessly. One of the components of the React Router is Link.
In this article, we will be seeing the working of the Link component, various props, and usage of the Link component within React Router in developing Single Page Applications.
Prerequisites:
Working of Link Component:
The link component is used for Routing from one page to another page without loading the page itself. This happens with the help of the react-router-dom module which contains (Browser Router, Routes, and Route) along with the Link component. So, when a user clicks on a link to navigate to another page, then that path is captured by the link component and compared with the path of the components wrapped inside the router. If it matches, then that page is rendered without any reload.
What are Props ?
In react, the components have some properties which are known as props to customize their behavior. The Link components have several props that help to control their behavior during navigation. let us see the different types of props and their examples.
What is ‘to’ prop ?
This is the most used props for routing. It helps in specifying the target location to which the link should navigate. It can be a string representing the path name or an object with path name. When you click on Dashboard you will be redirected to that component.
Syntax of ‘to’ prop:
<Link to = "/dashboard">
Dashboard
</Link>
What is ‘reloadDocument’ prop ?
This prop controls whether a full page reload should occur when the link is clicked. setting it true will reload the page which can be useful in certain cases.
Syntax of ‘reloadDocument’ prop:
<Link to = "/contactUs" reloadDocument = {true}>
Contact
</Link>
What is ‘replace’ prop ?
When you use this prop with the link component, it means that when the user clicks on the link, the current page history in the browser will be replaced by the new one. means that the current page won’t be remembered in the history, assuming as if the user never visited that page ever.
Syntax of ‘replace’ prop:
<Link to = "/aboutUs" replace = {true} >
About
</Link>
This prop is used to overcome the default behavior of scrolling to the top position if navigated to another component. If suppose the user is reading a blog and he clicks on comment section with ‘preventScrollReset = {true}’ then the page will navigate to the comment section without resetting the scroll position. without ‘preventScrollReset’ the default behavior will scroll the user back to the top page when they navigate to a new location.
Syntax of ‘preventScrollReset’:
<Link to = "/blog" preventScrollReset = {true}>
Go to Blog
</Link>
Parsing Attributes
Sometimes it is important to pass additional attributes to the Link component. We can achieve this by passing the props directly to the Link Component. Here in the below example, I have added two additional props such as className for styling the link and target attribute to navigate to the location in new tab.
Syntax to pass attributes:
<Link to = "/home" className="home" target="_blank">
Home
</Link>
Use of Link Component:
- First create the components that you want to navigate to. for example, Home.jsx
- In your App.jsx, wrap the components inside a Router and define the routes using the Route component. Mention the path and element name inside the route for that respective component.
- Create one more component (for example: Navbar.jsx) and render it inside Home.jsx, About.jsx and Contact.jsx this component contains the Link tag which has the exact same path (declared in variable “to”) as the route has in it.
Example: Below is an example of link component in React Router.
Javascript
import {
BrowserRouter as Router,
Routes,
Route
} from "react-router-dom" ;
import Home from "./Home" ;
import About from "./About" ;
import Contact from "./Contact" ;
function App() {
return (
<>
<Router>
<Routes>
<Route path= "/" element={<Home />} />
<Route path= "/about" element={<About />} />
<Route path= "/contact" element={<Contact />} />
</Routes>
</Router>
</>
);
}
export default App;
|
Javascript
import React from "react" ;
import { Link } from "react-router-dom" ;
const Navbar = () => {
return (
<>
<div
className= "nav"
style={{
display: "flex" ,
justifyContent: "space-evenly" ,
marginTop: "1rem" ,
color: "green" ,
fontWeight: "bolder" ,
fontSize: "1rem" ,
}}
>
<Link to= "/" >Home</Link>
<Link to= "/about" >About</Link>
<Link to= "/contact" >Contact Us</Link>
</div>
</>
);
};
export default Navbar;
|
Javascript
import React from "react" ;
import Navbar from "./Navbar" ;
const Home = () => {
return (
<>
<Navbar /> <br />
<div style={{
display: "flex" ,
justifyContent: "center"
}}>
This is
<span style={{ color: "green" }}>
GeeksForGeeks
</span>
Home
Page
</div>
</>
);
};
export default Home;
|
Javascript
import React from "react" ;
import Navbar from "./Navbar" ;
const About = () => {
return (
<>
<Navbar /> <br />
<div style={{
display: "flex" ,
justifyContent: "center"
}}>
This is
<span style={{ color: "green" }}>
GeeksForGeeks
</span>
About Page
</div>
</>
);
};
export default About;
|
Javascript
import React from "react" ;
import Navbar from "./Navbar" ;
const Contact = () => {
return (
<>
<Navbar /> <br />
<div style={{
display: "flex" ,
justifyContent: "center"
}}>
This is <span style={{ color: "green" }}>
GeeksForGeeks
</span>
Contact Page
</div>
</>
);
};
export default Contact;
|
Output:
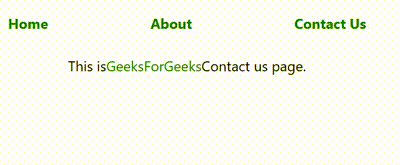
Share your thoughts in the comments
Please Login to comment...