Explain lifecycle of ReactJS due to all call to this.setState() function
Last Updated :
16 Nov, 2023
In React, the states are used to store data for specific components. These states can be updated accordingly using this.setState function. Updating of state leads to the rendering of UI. Different lifecycle methods are called during these renderings.
Lifecycle methods called when the app is loaded first:
- Constructor()
- render()
- componentDidMount()
Lifecycle methods are called when this.setState is called:
- render()
- componentDidUpdate()
Prerequisites:
Approach:
To understand the lifecycle of ReactJS due to all calls to this.setState() function we will create a UI that sends a call to this.setState. Users can interact with the UI and click on the button to trigger an event to call this.setState through this. Users can view all the lifecycle methods mentioned above in the console view.
Steps to Create React Application:
Step 1: To create a react app you need to install react modules through npx command. “npx” is used instead of “npm” because you will be needing this command in your app’s lifecycle only once.
npx create-react-app project_name
Step 2: After creating your react project move into the folder to perform different operations.
cd project_name
Project Structure:
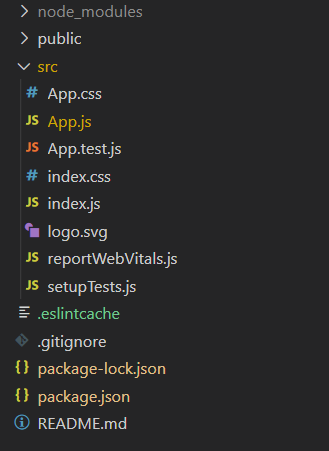
Project_Structure
Example: This example implements a button that sends a call to update the state through this.setState.
Javascript
import React from "react" ;
class App extends React.Component {
constructor() {
super ();
this .state = {
message: "initial state" ,
};
console.log( "Constructor called" );
}
componentDidMount() {
console.log( "componentDidMount called" );
}
componentDidUpdate() {
console.log( "componentDidUpdate called" );
}
call() {
this .setState({ message: "state updated" });
}
render() {
console.log( "render called" );
return (
<div style={{ margin: 100 }}>
<button
onClick={() => {
this .call();
}}
>
Click to update state!
</button>
<p>{ this .state.message}</p>
</div>
);
}
}
export default App;
|
Step to run the application: Open the terminal and type the following command.
npm start
Output: Open your browser. It will by default open a tab with localhost running (http://localhost:3000/) and you can see the output shown in the console. Appropriate functions are called when the state is updated.
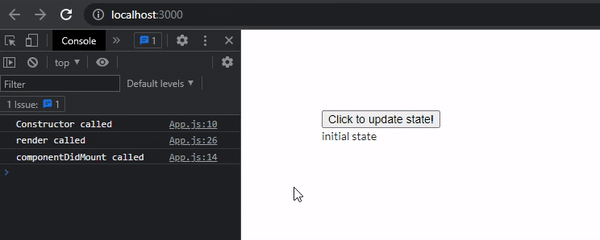
React State Update
Share your thoughts in the comments
Please Login to comment...