Reacting to React: Front-End Development in MERN
Last Updated :
27 Mar, 2024
In the ever-evolving landscape of web development, the MERN stack has emerged as a powerful toolkit for building dynamic and robust applications. Comprising MongoDB, ExpressJS, ReactJS, and NodeJS, the MERN stack offers a comprehensive ecosystem for full-stack development.
In this article, we’ll delve into the intricacies of front-end development using ReactJS within the MERN stack.
Introduction of MERN Stack:
The MERN stack represents a collection of technologies that work seamlessly together to enable the development of modern web applications. It consists of:
- MongoDB: A NoSQL database for storing data in a flexible, JSON-like format. MongoDB is an open-source document-oriented database that is designed to store a large scale of data and also allows you to work with that data very efficiently.
- ExpressJS: Express is a small framework that works on top of Node web server functionality to simplify its APIs and add helpful new features. It makes it easier to organize your application’s functionality with middleware and routing. It adds helpful utilities to Node HTTP objects and facilitates the rendering of dynamic HTTP objects.
- ReactJS: It is a popular JavaScript library for building user interfaces. It is also referred to as a front-end JavaScript library. It was developed by Facebook and is widely used for creating dynamic and interactive web applications. In this article, we’ll explore the key concepts of React.
- NodeJS: Node is a JavaScript runtime environment and platform that enables the execution of code on the server side. It allows developers to execute JavaScript code outside of a web browser, enabling the development of scalable and efficient network applications.
Introduction to MVC Architecture:
MVC (Model-View-Controller) is a software design pattern commonly used in web development. It separates an application into three interconnected components:
- Model: Represents the data and business logic of the application.
- View: Presents the user interface to the user, often in the form of HTML pages.
- Controller: Handles user input and updates the model and view accordingly.
- View in MVC: Key Characteristics and Responsibilities:
The view in MVC is responsible for presenting the user interface to the user. Key characteristics and responsibilities include:
- Rendering data from the model to the user interface.
- Handling user interactions and events.
- Ensuring a responsive and intuitive user experience.
React Components and JSX:
ReactJS adopts a component-based architecture, where UIs are built using reusable components. Key concepts include:
- Components: Modular, self-contained units of UI.
- JSX (JavaScript XML): JSX is a syntax extension that allows developers to write HTML-like code within JavaScript, simplifying the creation of React components.
State Management in React:
State Management in React involves managing the internal state of components. Key concepts include:
- State: Data that is local to a component and can change over time.
- setState(): A method used to update the state of a component.
- Hooks: Functions that enable functional components to use state and other React features.
Routing with React Router:
React Router is a popular library for handling routing in React applications. Key features include:
- Declarative Routing: Defining routes using JSX syntax.
- Dynamic Routing: Supporting dynamic route parameters and nested routes.
- History Management: Utilizing browser history API for navigation.
Steps to Create a React Application:
Step 1: Create a new ReactJS project using the following command
npx create-react-app <<Project_Name>>
Step 2: Change to the project directory.
cd <<Project_Name>>
Example: Below is an example of Reacting to React.
JavaScript
import React from 'react';
function HelloWorld() {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
}
export default HelloWorld;
Output:
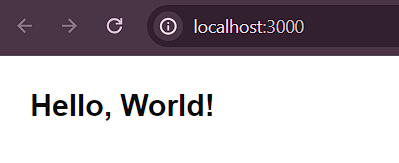
Conclusion:
Front-end development in the MERN stack offers a rich and rewarding experience for developers. By harnessing the power of ReactJS, developers can build dynamic and responsive user interfaces with ease. Understanding the MVC architecture, React components, state management, routing, and leveraging the MERN stack empowers developers to create modern web applications that meet the demands of today’s users.
Share your thoughts in the comments
Please Login to comment...