React MUI Radio Input
Last Updated :
03 Jan, 2023
React MUI is a UI library that provides fully-loaded components, bringing our own design system to our production-ready components. MUI is a user interface library that provides predefined and customizable React components for faster and easy web development, these Material-UI components are based on top of Material Design by Google.
React MUI Radio Group Input is a group radio button that allows the user to select one option from a set. It must consist of the most commonly used option selected by default.
Radio Input variants:
- Radio group: It is a wrapper used to group multiple radio components.
- Standalone radio buttons: The radio buttons can be used standalone, without the RadioGroup wrapper.
- Size: The size prop is used to control the radio sizes.
- Color: The color prop is used to add the radio colors like secondary, success, and default.
- Label placement: The label placement can be changed with the FormControlLabel component’s labelPlacement prop.
- Show error: An error will be displayed if no value is selected when the form is submitted.
- Customization: A radio can be customized with custom styles.
- useRadioGroup: A useRadioGroup() hook is used for advanced customization.Â
- Accessibility: To make it accessible make sure to have all form controls like having labels, etc.
- API: The <FormControl />, <FormControlLabel />, <FormLabel />, <Radio />, <RadioGroup /> APIs are used.
Â
Syntax:
<FormControl>
<FormLabel>Label</FormLabel>
<RadioGroup>
<FormControlLabel control={<Radio />}
label="option1" />
<FormControlLabel control={<Radio />}
label="option2" />
</RadioGroup>
</FormControl>
Creating React Project:
Step 1: To create a react app, you need to install react modules through the npm command.
npm create-react-app project name
Step 2: After creating your react project, move into the folder to perform different operations.
cd project name
Step 3: After creating the ReactJS application, Install the required module using the following command:
npm install @mui/material @emotion/react @emotion/styled
Project Structure:
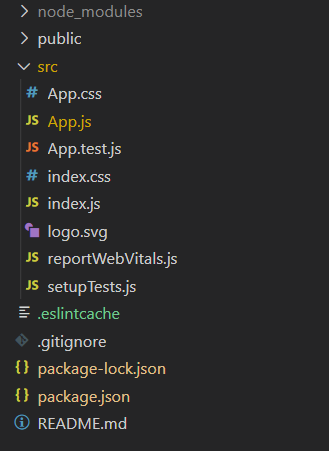
Â
Step to Run Application:
npm start
Example 1: Below example demonstrates the React MUI basic radio button input of different sizes and colors.
Javascript
import React from "react" ;
import Radio from '@mui/material/Radio' ;
import RadioGroup from '@mui/material/RadioGroup' ;
import FormControlLabel from '@mui/material/FormControlLabel' ;
import FormControl from '@mui/material/FormControl' ;
import FormLabel from '@mui/material/FormLabel' ;
function App() {
return (
<center>
<div>
<h1 style={{ color: 'green' }}>
GeeksforGeeks</h1>
<h2>React MUI Radio Input</h2>
</div>
<FormControl>
<FormLabel>Do you like GeeksforGeeks?</FormLabel>
<RadioGroup
defaultValue= "yes"
name= "radio-buttons-group"
>
<FormControlLabel value= "yes"
control={<Radio size= "small"
color= "success" />} label= "Yes" />
<FormControlLabel
value= "no"
control={<Radio color= "secondary" />}
label= "No" />
</RadioGroup>
</FormControl>
</center>
);
}
export default App;
|
Output:
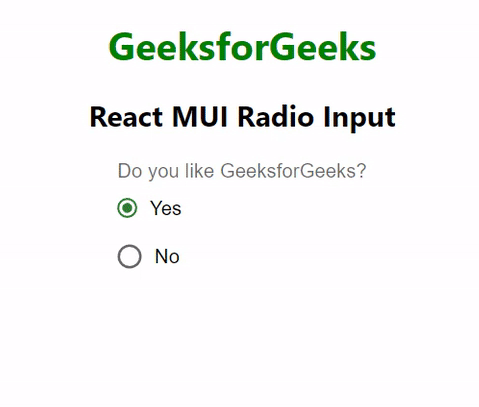
Â
Example 2: Below example demonstrates the React MUI radio button with different label placements.
Javascript
import React from "react" ;
import Radio from '@mui/material/Radio' ;
import RadioGroup from '@mui/material/RadioGroup' ;
import FormControlLabel from '@mui/material/FormControlLabel' ;
import FormControl from '@mui/material/FormControl' ;
import FormLabel from '@mui/material/FormLabel' ;
function App() {
return (
<center>
<div>
<h1 style={{ color: 'green' }}>GeeksforGeeks</h1>
<h2>React MUI Radio Input</h2>
</div>
<FormControl>
<FormLabel>Rate GFG</FormLabel>
<RadioGroup
row
defaultValue= "vm"
name= "radio-buttons-group"
>
<FormControlLabel labelPlacement= "start"
value= "vm" control={<Radio size= "small"
color= "success" />} label= "Very much" />
<FormControlLabel labelPlacement= "top"
value= "av" control={<Radio color= "secondary" />}
label= "Average" />
<FormControlLabel labelPlacement= "bottom"
value= "no" control={<Radio color= "secondary" />}
label= "Not much" />
<FormControlLabel labelPlacement= "end"
value= "vb" control={<Radio color= "secondary" />}
label= "Very bad" />
</RadioGroup>
</FormControl>
</center>
);
}
export default App;
|
Output:
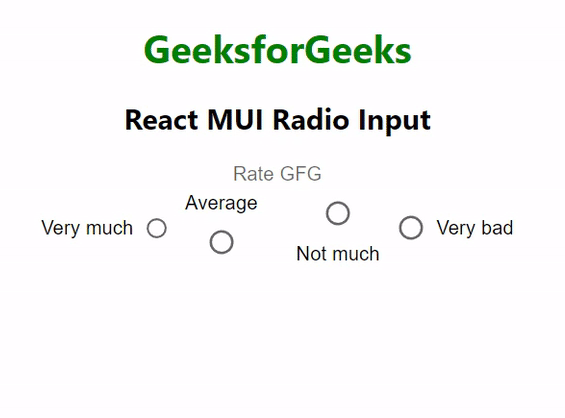
Â
Reference: https://mui.com/material-ui/react-radio-button/
Share your thoughts in the comments
Please Login to comment...