React MUI Container API
Last Updated :
03 Nov, 2022
Material-UI is a user interface library that provides predefined and customizable React components for faster and easy web development, these Material-UI components are based on top of Material Design by Google. In this article let’s discuss the Container API in the Material-UI library.
The container is used to center content horizontally. It’s the most basic layout element. In Material UI we have a <Container /> component used for several purposes through its props.
Props List:
- classes: It is used to add or override the styles of the component.
- component: It is mainly used for the root node.
- disableGutters: The padding is removed from the left and right if it is set to true.
- fixed: It tries to set the max-width to the min-width of the current breakpoint.
- maxWidth: It is used to set the max-width of the container because the container width increases with the size of the screen. You can set it to false to disable maxWidth.
- sx: It can be used to override the system and additional CSS styles also.
Fluid Container: A fluid container width is bounded by the maxWidth prop value.
Syntax:
<Container>This is container</Container>
Installing React App:
Step1: Create a React app using the following command.
npx create-react-app container-example
Step 2: Now get into the project directory.
cd container-example
Installing Material-UI: Installing Material-UI’s source files via npm/yarn, and they take care of injecting the CSS needed.
npm install @material-ui/core
OR
yarn add @material-ui/core
Project Structure:
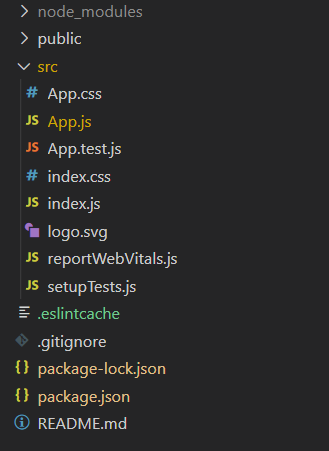
project structure
Importing Container:
import Container from '@mui/material/Container';
Example 1: In this example, we will see the container width grows with the size of the screen.
Javascript
import * as React from 'react' ;
import CssBaseline from '@mui/material/CssBaseline' ;
import Box from '@mui/material/Box' ;
import Container from '@mui/material/Container' ;
export default function SimpleContainer() {
return (
<React.Fragment>
<CssBaseline />
<Container maxWidth= "sm" >
<Box sx={{ bgcolor: '#ffe8dc' ,
height: '100vh' }} >
<h1>GFG Container API</h1>
<h2>Fluid Container</h2>
</Box>
</Container>
</React.Fragment>
);
}
|
Output:
Fixed Container: We use this when we want to design for a fixed set of sizes.
Example 2: In this example, we will set the max-width to match the min-width of the current breakpoint.
Javascript
import * as React from 'react' ;
import CssBaseline from '@mui/material/CssBaseline' ;
import Box from '@mui/material/Box' ;
import Container from '@mui/material/Container' ;
export default function FixedContainer() {
return (
<React.Fragment>
<CssBaseline />
<Container fixed>
<Box sx={{ bgcolor: '#cfe8fc' , height: '100vh' }} >
<h1>GFG Container API</h1>
<h2>Fixed Container</h2>
</Box>
</Container>
</React.Fragment>
);
}
|
Output:
Reference: https://mui.com/material-ui/api/container/
Share your thoughts in the comments
Please Login to comment...