React MUI Text Field Input
Last Updated :
03 Jan, 2023
React MUI is a UI library that provides fully-loaded components, bringing our own design system to our production-ready components. MUI is a user interface library that provides predefined and customizable React components for faster and easy web development, these Material-UI components are based on top of Material Design by Google.
React MUI Text Field Input is a text field that allows the users to input the text and edit it. They mostly appear in forms and dialog boxes.
React MUI TextField variants:
- Basic TextField: This is the default text field which includes label, input, text, etc.
- Form props: Form attributes like required, disabled, type, etc., and a helperText are used.
- Validation: Errors are validated through helperText prop.
- Multiline: It is defined by a prop that transforms the text field into a TextareaAutosize element.
- Select: It is defined by a prop select that makes the text field use the Select component internally.
- Icons: It is defined to display the icon within the input field.
- Sizes: There are two sizes available to change the input field.
- Margin: It is defined by a prop that is used to change the vertical spacing of the text field.
- Full width: A prop can be used to make the input take up the full width of its container.
- Uncontrolled vs. Controlled: An input can be controlled or uncontrolled.
- Components: TextField is composed of smaller components that can be styled accordingly.
- Inputs: Input elements are also used to take input as like a textfield.
- Color: A color of the text field has defined a prop that changes the highlight color of the text field when focused.
- Customization: Textfield can be customized as per the choice.
- useFormControl: It is a hook that returns the context value of the parent FormControl component.
- Limitations: It is used to set the limitations on the input field such as shrink, floating label, type, or helper text.
- Integration with 3rd party input libraries: The third-party libraries can be added to format an input.
- Accessibility: To make the text field accessible, the input should be linked to the label and the helper text.
- Unstyled: An unstyled version of React MUI textfield is also available to use.
- API: The used APIs are <FilledInput />, <FormControl />, <FormHelperText />, <Input />, <InputAdornment />, <InputBase />, <InputLabel />, <OutlinedInput />, and <TextField />.
Â
Syntax:
<TextField label="Enter you input" variant="outlined" />
Creating React Project:
Step 1: To create a react app, you need to install react modules through the npm command.
npm create-react-app project name
Step 2: After creating your react project, move into the folder to perform different operations.
cd project name
Step 3: After creating the ReactJS application, Install the required module using the following command:
npm install @mui/material @emotion/react @emotion/styled
Project Structure:
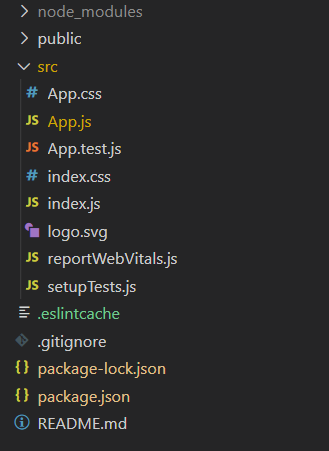
Â
Step to Run Application:
npm start
Example 1: Below example demonstrates the React MUI basic text input field of different sizes and colors.
Javascript
import { TextField } from "@mui/material" ;
import React from "react" ;
function App() {
return (
<center>
<div>
<h1 style={{ color: "green" }}>
GeeksforGeeks</h1>
<h2>React MUI Text Field Input</h2>
<div>
<TextField
label= "Small Outlined Input"
variant= "outlined"
size= "small"
color= "warning"
style={{ marginRight: 10 }}
/>
<TextField
label= "Normal Filled Input"
variant= "filled"
color= "secondary"
style={{ marginRight: 10 }}
/>
<TextField
label= "Normal Standard Input"
variant= "standard"
color= "success"
/>
</div>
</div>
</center>
);
}
export default App;
|
Output:
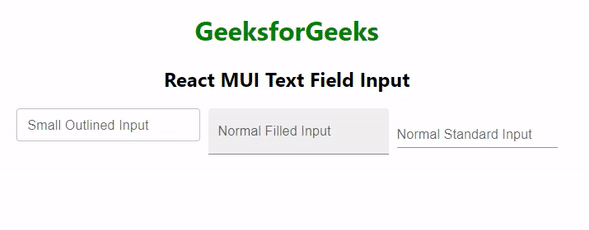
Â
Example 2: Below example demonstrates the React MUI text input field using a full-width select component with icons.
Javascript
import React, { useState } from "react" ;
import { TextField } from "@mui/material" ;
import InputAdornment from "@mui/material/InputAdornment" ;
import { Source } from "@mui/icons-material" ;
const data = [
{
value: "Stack" ,
},
{
value: "Queue" ,
},
{
value: "Linked List" ,
},
{
value: "Array" ,
},
];
function App() {
const [dataStructure, setDataStructure] = useState( "Array" );
const handleChange = (e) => {
setDataStructure(e.target.value);
};
return (
<center>
<div>
<h1 style={{ color: "green" }}>GeeksforGeeks</h1>
<h2>React MUI Text Field Input</h2>
<div style={{ maxWidth: "98%" }}>
<TextField
fullWidth
select
label= "Select"
value={dataStructure}
variant= "outlined"
onChange={handleChange}
SelectProps={{
native: true ,
}}
helperText= "Select a Data Structure"
InputProps={{
startAdornment: (
<InputAdornment position= "start" >
<Source />
</InputAdornment>
),
}}
>
{data.map((option) => (
<option key={option.value} value={option.value}>
{option.value}
</option>
))}
</TextField>
<TextField
fullWidth
select
label= "Select"
value={dataStructure}
variant= "filled"
style={{ marginTop: 10 }}
onChange={handleChange}
SelectProps={{
native: true ,
}}
helperText= "Select a Data Structure"
InputProps={{
startAdornment: (
<InputAdornment position= "start" >
<Source />
</InputAdornment>
),
}}
>
{data.map((option) => (
<option key={option.value}
value={option.value}>
{option.value}
</option>
))}
</TextField>
<TextField
fullWidth
select
label= "Select"
value={dataStructure}
variant= "standard"
style={{ marginTop: 10 }}
onChange={handleChange}
SelectProps={{
native: true ,
}}
helperText= "Select a Data Structure"
InputProps={{
startAdornment: (
<InputAdornment position= "start" >
<Source />
</InputAdornment>
),
}}
>
{data.map((option) => (
<option key={option.value}
value={option.value}>
{option.value}
</option>
))}
</TextField>
</div>
</div>
</center>
);
}
export default App;
|
Output:
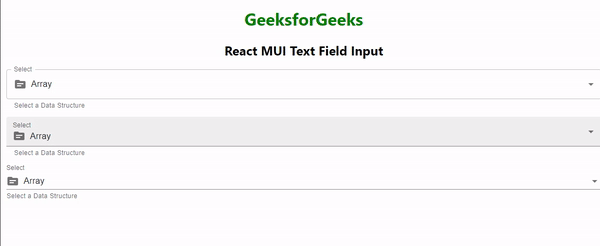
Â
Reference: https://mui.com/material-ui/react-text-field
Share your thoughts in the comments
Please Login to comment...