React MUI Popper Util
Last Updated :
24 May, 2023
MUI or Material-UI is a UI library providing predefined robust and customizable components for React for easier web development. The MUI design is based on top of Material Design by Google.
In this article, we will discuss the React MUI Popper. The Popper provides additional context for an element whenever clicked or hovered on it in form of a pop-up.
Import Popper:
import Popper from '@mui/material/Popper'
// or
import { Popper } from '@mui/material'
Â
React MUI Popper Props:
- open: It is a boolean value. It determines whether the popper should be open or not.
- anchorEl: It is a function or an element that determines the position of the element.
- children: It refers to the popper render function or node.
- components: The components used for the root node. Either a string to use an HTML element or a component.
- componentsProps: Â It refers to the props used for each slot.
- container: It is a function or an element that will have the portal children appended to it.
- disablePortal: It is a boolean value. It determines whether the children will be under the DOM hierarchy of the parent component or not. It is false by default.
- keepMounted: It is a boolean value. It determines whether there to keep the children in the DOM or not. It is false by default.
- modifiers: It is a function that helps to compute the position of the popper.Â
- placement: It determines the placement of the popper.
- popperOptions: Different options for the pooper component.
- popperRef: It is referred to as the used popper ref.
- slotProps: props used for each slot inside the Popper.
- slots: It is either a string to use an HTML element or a component, used for each slot inside the Popper.
- sx: The prop to override and add styles.
- transition: It is a boolean value. It determines whether there should be any transition or not. It is false by default.
Syntax:
<Popper ></Popper>
Creating React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: After creating the ReactJS application, Install the required module using the following command:
npm install @mui/materialÂ
npm install @emotion/reactÂ
npm install @emotion/styled
Project Structure: It will look like the following.
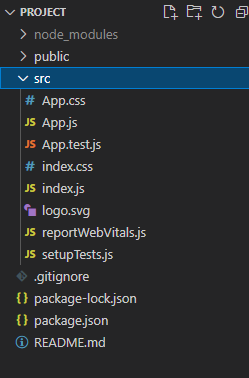
Â
Example 1: We are creating a state named open using react hook useState. We are creating an onMouseover function named as handleOnMouseOver that changes the state. We are adding the popper element and a button that triggers the handleOnMouseOver function on click.
App.js
Javascript
import { Popper } from '@mui/material' ;
import React from 'react' ;
export default function App() {
const [anchorEl, setAnchorEl] = React.useState( null );
const open = Boolean(anchorEl);
const handleOnMouseOver = (e) => {
setAnchorEl(anchorEl ? null : e.currentTarget)
}
return (
<div style={{ display: 'block' , padding: 30 }}>
<h1 style={{ color: 'green' }}>GeeksforGeeks</h1>
<h4>React MUI Popper Util </h4>
<button type= "button"
onMouseOver={handleOnMouseOver}>
click me
</button>
<Popper
open={open}
anchorEl={anchorEl}
placement= 'bottom-start'
>
<h3 style={{ color: "red" }}>Welcome User!</h3>
<p>placement: bottom-start</p>
</Popper>
</div>
);
}
|
Step to Run Application: Run the application using the following command from the root directory of the project.
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output.
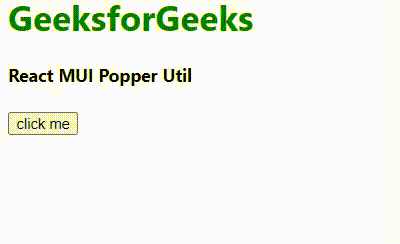
Â
Example 2: In this example, we are creating a state using react hook useState naming anchorEl. We are then passing the boolean value to open. We are adding a function named handleClick which changes the state. To the Popper component, we are passing the open, anchorEl, and placement prop.
App.js
Javascript
import { Popper } from '@mui/material' ;
import React from 'react' ;
function App() {
const [anchorEl, setAnchorEl] = React.useState( null );
const open = Boolean(anchorEl);
const handleClick = (e) => {
setAnchorEl(anchorEl ? null : e.currentTarget)
}
return (
<div style={{ display: 'block' , padding: 30 }}>
<h1 style={{ color: 'green' }}>GeeksforGeeks</h1>
<h4>React MUI Popper Util </h4>
<button type= "button" onClick={handleClick}>
click me
</button>
<Popper
open={open}
anchorEl={anchorEl}
placement= 'bottom-start'
>
<h3 style={{ color: "red" }}>Welcome User!</h3>
<p>placement: bottom-start</p>
</Popper>
</div>
);
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project.
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output.
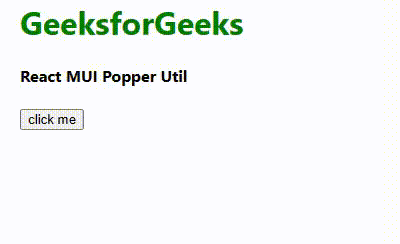
Â
Reference: https://mui.com/material-ui/api/popper/
Share your thoughts in the comments
Please Login to comment...