React MUI Button Input
Last Updated :
03 Jan, 2023
React MUI is a UI library that provides fully-loaded components, bringing our own design system to our production-ready components. MUI is a user interface library that provides predefined and customizable React components for faster and easy web development, these Material-UI components are based on top of Material Design by Google.
React MUI Button Input is a button that allows users to take actions or make choices only with the help of a single tap/click.
React MUI Button Variants:
- Basic button: A basic button has three variants: text, contained, and outlined.Â
- Handling clicks: Every button accepts the onClick() handler.
- Color: There are three colors available for buttons.Â
- Sizes: Buttons are available in small, medium, and large.Â
- Upload button: A upload button is used for uploading any files.
- Buttons with icons and label: Buttons are also used along with the icons and text labels.
- Icon button: These buttons allow a single choice to be selected or deselected.
- Customization: Buttons can be customized with custom styles.
- Complex button: use ButtonBase to build custom button interactions.
- Third-party routing library: The ButtonBase component provides the component prop to handle the navigation on the client only.
- Limitations: There are limitations like the cursor not being allowed in buttons.
- Unstyled: React MUI provides the unstyled buttons.
- API: The <Button />, <ButtonBase />, <IconButton />, <LoadingButton /> APIs are used.
Â
Syntax:
<Button variant="text">Name</Button>
Creating React Project:
Step 1: To create a react app, you need to install react modules through the npm command.
npm create-react-app project name
Step 2: After creating your react project, move into the folder to perform different operations.
cd project name
Step 3: After creating the ReactJS application, Install the required module using the following command:
npm install @mui/material @emotion/react @emotion/styled
Project Structure:
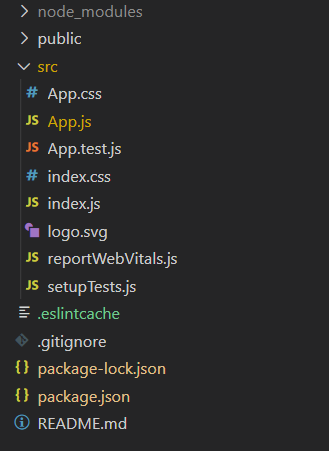
Â
Step to Run Application:
npm start
Example 1: Below example demonstrates the React MUI buttons of different variants, sizes, and colors.
Javascript
import React from "react" ;
import Button from "@mui/material/Button" ;
import { Stack } from "@mui/system" ;
function App() {
return (
<center>
<div>
<h1 style={{ color: 'green' }}>
GeeksforGeeks</h1>
<h2>React MUI Button Input</h2>
</div>
<Stack spacing={1} direction= 'row'
justifyContent= "center" >
<Button color= "error" size= "small"
variant= "text" >Click</Button>
<Button color= "secondary" size= "medium"
variant= "contained" >Click</Button>
<Button color= "success" size= "large"
variant= "outlined" >Click</Button>
</Stack>
</center>
);
}
export default App;
|
Output:
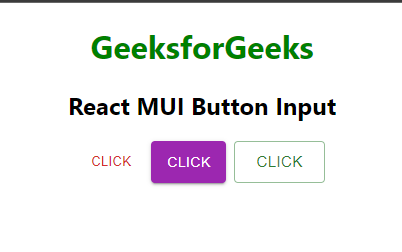
Â
Example 2: Below example demonstrates the React MUI buttons with icons.
Javascript
import React from "react" ;
import Button from "@mui/material/Button" ;
import { Stack } from "@mui/system" ;
import FacebookIcon from '@mui/icons-material/Facebook' ;
import { Login } from "@mui/icons-material" ;
function App() {
return (
<center>
<div>
<h1 style={{ color: 'green' }}>
GeeksforGeeks</h1>
<h2>React MUI Button Input</h2>
</div>
<Stack spacing={1} direction= 'row'
justifyContent= "center" >
<Button size= "large" variant= "contained"
startIcon={<FacebookIcon />}>Facebook</Button>
<Button size= "large" variant= "outlined"
endIcon={<Login />}>Log in </Button>
</Stack>
</center>
);
}
export default App;
|
Output:
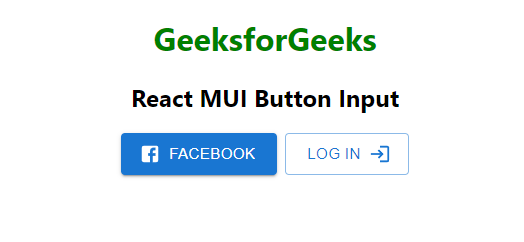
Â
Reference: https://mui.com/material-ui/react-button/
Share your thoughts in the comments
Please Login to comment...