Python is known for its easy-to-code and intuitive syntax. And one-liners are like the cherry on the cake which makes Python a more beautiful and beloved programming language. There are numerous features in Python Language and one of its most loved features is its one-liners. Writing Code in Python is already easy compared to other programming languages and using the one-liners makes it more easier and cool. Now let’s see what are these Python one-liners.
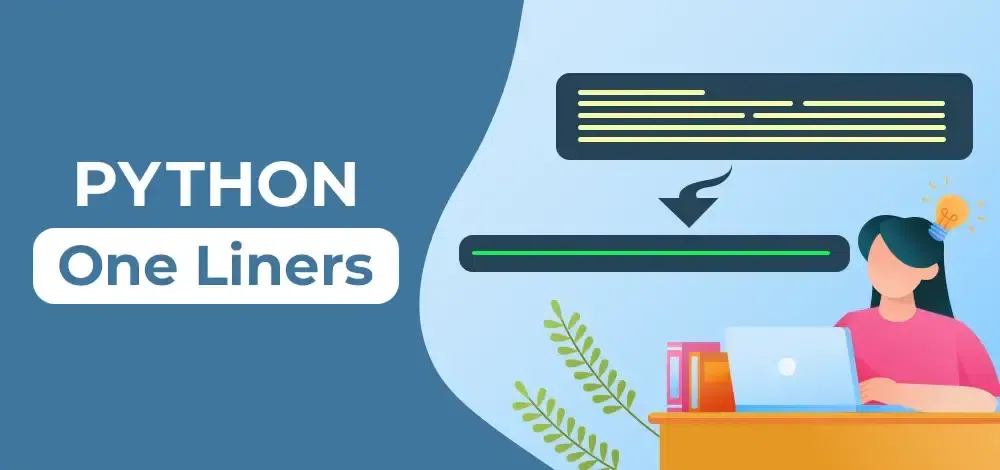
What are Python one-liners?
One-liners help to reduce the number of lines of code by providing alternative syntax which is both easier to read and concise. One-liners are the syntactic sugars, which by adding simplicity and readability to the code makes it “sweeter” for the users to understand and code. These are short but powerful programs to do the task in a single line of code. One-liners are not present in all programming languages thus, making Python stand out.
Why use Python one-liners?
One-liners help improve the code quality by conveying the same meaning in lesser lines of code. They reduce the unnecessary syntactic code which makes it more readable for others. Also, it helps save a lot of time and effort by allowing the programmers to focus more on logic implementation rather than syntax.
Here are 10 useful Python one-liners that come in very handy in a developer’s day-to-day life to code faster.
1. Swap two variables
Traditional Way
Python3
first = 1
second = 2
temp = first
first = second
second = temp
|
Here, we have used a variable temp to store the value of the variable first so that it doesn’t get lost while swapping
Python One Liner
Python3
first = 1
second = 2
first, second = second, first
print (first, second)
|
After the execution of the above code, the value of variable first will become what it was for second i.e. 2 and that of second will become 1.
2. List Comprehension
List comprehension is a shorthand method to create a new list by changing the elements of the already available iterable (like list, set, tuple, etc).
Syntax:
new_list = [expression for variable in iterable]
Traditional way
Python3
new_list = []
for variable in iterable:
new_list.append(expression)
|
How does it work?
We iterate over all the values in the iterable one by one and keep storing them in a variable. Now, based on the value of the variable, the expression is evaluated for each value and this expression is stored in the new_list created.
Let’s have a look at an example:
Traditional way
Python3
nums = [ 1 , 2 , 3 , 4 , 5 ]
squares = []
for val in nums:
squares.append(val * val)
print (squares)
|
One Liner
Python3
nums = [ 1 , 2 , 3 , 4 , 5 ]
squares = [val * val for val in nums]
print (squares)
|
List comprehension with conditionals
We can also use conditionals in list comprehension. For example, if we need to create a list containing only even numbers from a given list:
Traditional way
Python3
nums = [ 1 , 2 , 3 , 4 , 5 ]
even_nums = []
for val in nums:
if val % 2 = = 0 :
even_nums.append(val)
print (even_nums)
|
One liner
Python3
nums = [ 1 , 2 , 3 , 4 , 5 ]
even_nums = [val for val in nums if val % 2 = = 0 ]
print (even_nums)
|
List comprehension and nested loops
If we are given two lists one containing the x coordinates and the other containing the y coordinates and we have to create a list containing unordered pairs from these:
Traditional way
Python3
x = [ 1 , 2 , 3 ]
y = [ 4 , 5 , 6 ]
coordinates = []
for xcor in x:
for ycor in y:
coordinates.append((xcor, ycor))
print (coordinates)
|
One liner
Python3
x = [ 1 , 2 , 3 ]
y = [ 4 , 5 , 6 ]
coordinates = [(xcor, ycor) for xcor in x for ycor in y]
print (coordinates)
|
Similarly, we can also perform comprehensions in sets, dictionaries, etc
3. Lambda function
Lambda functions are anonymous functions i.e. functions without names. This is because they are mostly one-liners or are only required to be used once.
Syntax:
lambda argument(s): expression
lambda keyword is used to define an anonymous function in python. Arguments are the variables that are required to evaluate the expression. Expression is the body of the function. After evaluation, the expression is returned.
The lambda function can also be stored in a variable for future use.
Traditional way
Python3
def sum (a, b):
return a + b
sum ( 1 , 5 )
|
One Liner
Python3
def sum (a, b): return a + b
|
The above lambda function returns the sum of two values passed in arguments. The function is stored in a variable named, sum
To call the function:
sum(30, 12)
We can also directly call the lambda function where it is defined:
Python3
print (( lambda a, b: a + b)( 1 , 5 ))
|
where the first set of parenthesis contains the definition of the function and the second one contains the parameters passed.
4. map(), filter() and reduce()
Map function takes in a function and an iterable as arguments and applies the function on each value of iterable and returns themes.
Syntax:
map(function, iterable)
Traditional way
Python3
nums = [ 1 , 2 , 3 , 4 ]
def double(n):
return 2 * n
twice = []
for val in nums:
twice.append(double(val))
print (twice)
|
One Liner
Python3
nums = [ 1 , 2 , 3 , 4 ]
def double(n):
return 2 * n
twice = list ( map (double, nums))
print (twice)
|
Often, lambda functions are used in the map():
Python3
nums = [ 1 , 2 , 3 , 4 ]
twice = list ( map ( lambda x: 2 * x, nums))
print (twice)
|
Filter function also takes in a function (which may also be a lambda function) and an iterable and returns an iterator to an iterable object which contains values filtered according to the logic of the passed function.
Syntax:
filter(function, iterable)
Examples
Python3
nums = [ 1 , 20 , 3 , 5 , 15 ]
five_multiples = list ( filter ( lambda x: x % 5 = = 0 , nums))
print (five_multiples)
|
Reduce function takes in an iterable and a function (which may also be a lambda function) and returns a single value as a result thus, in a way reducing the entire iterable into a single value according to the logic of the passed function.
Syntax:
reduce(function, iterable)
Python3
from functools import reduce
nums = [ 2 , 3 , 5 , 15 ]
sum = reduce ( lambda x, y: x + y, nums)
print ( sum )
|
Python3
from functools import reduce
nums = [ 2 , 3 , 5 , 15 ]
max = reduce ( lambda x, y: x if x > = y else y, nums)
print ( max )
|
We can see that reduce takes two values at a time and compute their result according to the function definition.
E.g. to calculate the max value, the first 2 and 3 will be compared and the maximum of the two i.e. 3 is returned which is then compared with the subsequent number 5, and so on. Reduce function also works when the iterable contains only one element but will give an error when it is empty.
5. Walrus
The Walrus operator(:=) allows the user to create a variable as well as assign a value to it in the middle of an expression.
Traditional way
Python3
review = "good"
n = len (review)
if n < 10 :
print ( "Minimum 10 characters required" )
|
One Liner
Python3
review = "good"
if (n: = len (review)) < 10 :
print ( "Minimum 10 characters required" )
|
Here, the length of the string review is stored in a variable n which is defined and declared inside the if statement only.
6. Ternary operator
The ternary operator is shorthand for if else
Syntax:
expression1 if condition1 else expression2
Traditional way
Python3
age = 18
eligible = False
if age > = 18 :
eligible = True
else :
eligible = False
|
One Liner
Python3
age = 18
eligible = True if age > = 18 else False
|
If the condition in the if statement is true, the first expression is returned otherwise expression in the else part is returned.
7. Iterable or value Unpacking
Iterable or value unpacking allows to assign various variables on the left the values from an iterable or simply multiple values mentioned.
Traditional Way
Python3
a, b, c = [ 1 , 2 , 3 ]
print (a, b, c)
|
One Liner
Python3
a, * b, c = [ 1 , 2 , 3 , 4 , 5 ]
print (a)
print (b)
print (c)
|
Here, it is mandatory to assign values to a and c therefore, the values left for b are 2, 3 and 4.
ValueError while Unpacking
Python3
a, * b, c, d = [ 1 , 2 , 3 ]
print (a)
print (b)
print (c)
print (d)
|
Here, b is empty because it is mandatory to assign values to a, c, and d.
While the below code would give an error because of not having sufficient values to assign to a, c, d, and e.
Python3
a, * b, c, d, e = [ 1 , 2 , 3 ]
|
ValueError: not enough values to unpack (expected at least 4, got 3)
8. Space-separated integers to a list
It is a very common scenario while coding in Python to obtain integers from a string containing space-separated integers because the input in Python is by default in the form of a string.
Traditional way
Python3
values = input ().split()
nums = []
for i in values:
nums.append( int (i))
|
One Liner
Python3
nums = list ( map ( int , input ().split()))
|
Here, we have taken the input using the input() function which returns a string. This string is then split about spaces to get a list of strings containing integers as strings. This list is then passed to the map function along with the int() as the function to convert the string to integers. These integer values returned are then stored as a list in variable nums
Though this one-liner is a combination of the previous ones, this list would have been incomplete without mentioning it because this is a very common use case.
9. Leveraging print() to fullest
If we need to print the values of a list in a single line separated by spaces, we can do it simply by passing an iterator to the list in print() as a parameter.
Traditional way
Python3
nums = [ 1 , 2 , 3 ]
for i in nums:
print (i, end = " " )
|
One Liner
Python3
nums = [ 1 , 2 , 3 ]
print ( * nums)
|
This is because the definition of the print interface in Python documentation itself specifies that if we pass an iterator to an object in the print function, the values will be printed separated by a space and will end with a newline
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
10. Printing variable name along with value using fstring
Traditional way
Python3
number1 = 5
number2 = 7
print (f "number1={number1} number2={number2}" )
|
One Liner
Python3
number1 = 5
number2 = 7
print (f "{number1=} {number2=}" )
|
This can come in handy while debugging when we need to print the variable name along with its value.
Conclusion
One-liners no doubt make life easier but when used too much might also complicate things. Thus, it is always advised not to overuse them because the main purpose of one-liners is to improve the code quality and readability.
FAQs on Python One-liners
Q1. What is Python One-liners?
Answer:
One-liners help to reduce the number of lines of code by providing alternative syntax which is both easier to read and concise.
Q2. Is it necessary to use one-liners?
Answer:
No, they are just the add-ons. We can always code without using any one liners.
Q3. When to not use one-liners?
Answer:
When the code becomes large enough to be contained within a single line, try using the traditional methods. Else it becomes difficult to read.
Share your thoughts in the comments
Please Login to comment...