Python print() function prints the message to the screen or any other standard output device. In this article, we will cover about print() function in Python as well as it’s various operations.
Python print() Function SyntaxÂ
Syntax : print(value(s), sep= ‘ ‘, end = ‘\n’, file=file, flush=flush)
Parameters:Â
- value(s): Any value, and as many as you like. Will be converted to a string before printed
- sep=’separator’ : (Optional) Specify how to separate the objects, if there is more than one.Default :’ ‘
- end=’end’: (Optional) Specify what to print at the end.Default : ‘\n’
- file : (Optional) An object with a write method. Default :sys.stdout
- flush : (Optional) A Boolean, specifying if the output is flushed (True) or buffered (False). Default: False
Return Type: It returns output to the screen.
Though it is not necessary to pass arguments in the print() function, it requires an empty parenthesis at the end that tells Python to execute the function rather than calling it by name. Now, let’s explore the optional arguments that can be used with the print() function.
Example
In this example, we have created three variables integer, string and float and we are printing all the variables with print() function in Python.
Python3
name = "John"
age = 30
print("Name:", name)
print("Age:", age)
How print() works in Python?
You can pass variables, strings, numbers, or other data types as one or more parameters when using the print() function. Then, these parameters are represented as strings by their respective str() functions. To create a single output string, the transformed strings are concatenated with spaces between them.
In this code, we are passing two parameters name and age to the print function.
Python3
name = "Alice"
age = 25
print("Hello, my name is", name, "and I am", age, "years old.")
OutputHello, my name is Alice and I am 25 years old.
Python print() Function with Examples
Python String Literals
String literals in Python’s print statement are primarily used to format or design how a specific string appears when printed using the print() function.
- \n: This string literal is used to add a new blank line while printing a statement.
- “”: An empty quote (“”) is used to print an empty line.
Example
This code uses \n to print the data to the new line.
Python3
print("GeeksforGeeks \n is best for DSA Content.")
OutputGeeksforGeeks
is best for DSA Content.
Python “end” parameter in print()
The end keyword is used to specify the content that is to be printed at the end of the execution of the print() function. By default, it is set to “\n”, which leads to the change of line after the execution of print() statement.
Example
In this example, we are using print() with end and without end parameters.
Python3
# This line will automatically add a new line before the
# next print statement
print ("GeeksForGeeks is the best platform for DSA content")
# This print() function ends with "**" as set in the end argument.
print ("GeeksForGeeks is the best platform for DSA content", end= "**")
print("Welcome to GFG")
OutputGeeksForGeeks is the best platform for DSA content
GeeksForGeeks is the best platform for DSA content**Welcome to GFG
Print Concatenated Strings
In this example, we are concatenating strings inside print() function in Python.
Python3
print('GeeksforGeeks is a Wonderful ' + 'Website.')
OutputGeeksforGeeks is a Wonderful Website.
Output formatting
In this example, we are formatting our output to make it look more attractive by using str.format() function.
Python3
a,b,=10,1000
print('The value of a is {} and b is {}'.format(a,b))
OutputThe value of a is 10 and b is 1000
Python Input
In this example, we are using print() and input() in Python to take user input and print it in the output.
Python3
n = input('Enter the Number: ')
print('Number Entered by User:',n)
print(type(n))
Output
Enter the Number: 20
Number Entered by User: 20
<class 'str'>
Flush parameter in Python with print() function
The I/Os in Python are generally buffered, meaning they are used in chunks. This is where flush comes in as it helps users to decide if they need the written content to be buffered or not. By default, it is set to false. If it is set to true, the output will be written as a sequence of characters one after the other. This process is slow simply because it is easier to write in chunks rather than writing one character at a time. To understand the use case of the flush argument in the print() function, let’s take an example.
Example
Imagine you are building a countdown timer, which appends the remaining time to the same line every second. It would look something like below:
3>>>2>>>1>>>Start
The initial code for this would look something like below as follows:Â
Python3
import time
count_seconds = 3
for i in reversed(range(count_seconds + 1)):
if i > 0:
print(i, end='>>>')
time.sleep(1)
else:
print('Start')
So, the above code adds text without a trailing newline and then sleeps for one second after each text addition. At the end of the countdown, it prints Start and terminates the line. If you run the code as it is, it waits for 3 seconds and abruptly prints the entire text at once. This is a waste of 3 seconds caused due to buffering of the text chunk as shown below :
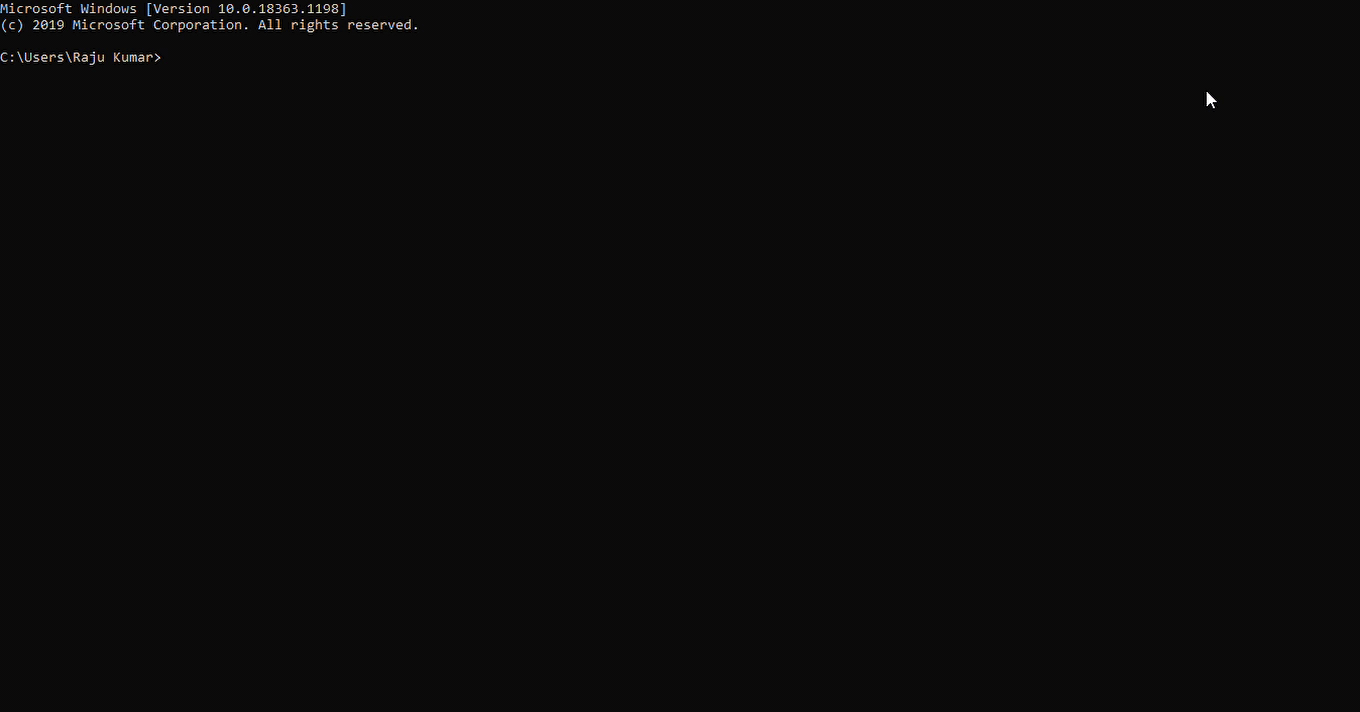
Though buffering serves a purpose, it can result in undesired effects as shown above. To counter the same issue, the flush argument is used with the print() function. Now, set the flush argument as true and again see the results.
Python3
import time
count_seconds = 3
for i in reversed(range(count_seconds + 1)):
if i > 0:
print(i, end='>>>', flush = True)
time.sleep(1)
else:
print('Start')
Output
.gif)
Python print() flush argument
Python “sep” parameter in print()
The print() function can accept any number of positional arguments. To separate these positional arguments, the keyword argument “sep” is used.
Note: As sep, end, flush, and file are keyword arguments their position does not change the result of the code.Â
Example
This code is showing that how can we use the sep argument for multiple variables.
Python3
a=12
b=12
c=2022
print(a,b,c,sep="-")
Example
Positional arguments cannot appear after keyword arguments. In the below example 10, 20 and 30 are positional arguments where sep=’ – ‘ is a keyword argument.
Python3
print(10, 20, sep=' - ', 30)
Output
File "0b97e8c5-bacf-4e89-9ea3-c5510b916cdb.py", line 1
print(10, 20, sep=' - ', 30)
^
SyntaxError: positional argument follows keyword argument
File Argument in Python print()
Contrary to popular belief, the print() function doesn’t convert messages into text on the screen. These are done by lower-level layers of code, that can read data(message) in bytes. The print() function is an interface over these layers, that delegates the actual printing to a stream or file-like object. By default, the print() function is bound to sys.stdout through the file argument.Â
With IO Module
This code creates a dummy file using the io module in Python. It then adds a message “Hello Geeks!!” to the file using the print() function and specifies the file parameter as the dummy file.
Python3
import io
# declare a dummy file
dummy_file = io.StringIO()
# add message to the dummy file
print('Hello Geeks!!', file=dummy_file)
# get the value from dummy file
print(dummy_file.getvalue())
Writing to a File with Python’s print() Function
This code is writing the data in the print() function to the text file.Â
Python3
print('Welcome to GeeksforGeeks Python world.!!', file=open('Testfile.txt', 'w'))
Output
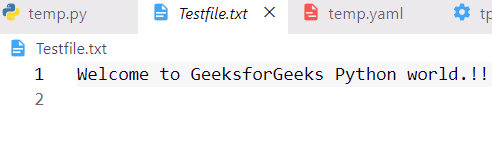
Python Print()
Share your thoughts in the comments
Please Login to comment...