Python Nested Loops
Last Updated :
05 Apr, 2023
In Python programming language there are two types of loops which are for loop and while loop. Using these loops we can create nested loops in Python. Nested loops mean loops inside a loop. For example, while loop inside the for loop, for loop inside the for loop, etc.
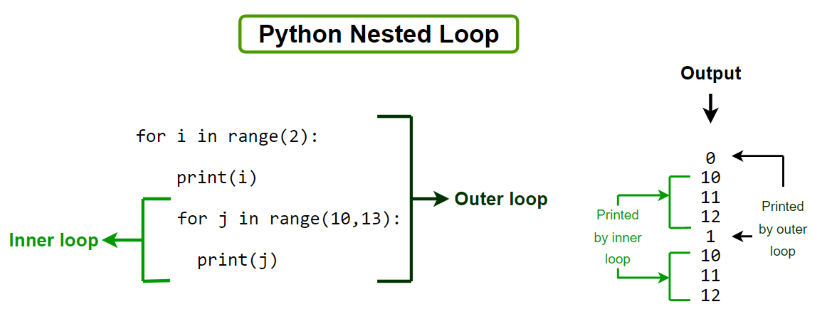
Python Nested Loops
Python Nested Loops Syntax:
Outer_loop Expression:
Inner_loop Expression:
Statement inside inner_loop
Statement inside Outer_loop
Python Nested Loops Examples
Example 1: Basic Example of Python Nested Loops
Python3
x = [ 1 , 2 ]
y = [ 4 , 5 ]
for i in x:
for j in y:
print (i, j)
|
Output:
1 4
1 5
2 4
2 5
Python3
x = [ 1 , 2 ]
y = [ 4 , 5 ]
i = 0
while i < len (x) :
j = 0
while j < len (y) :
print (x[i] , y[j])
j = j + 1
i = i + 1
|
Time Complexity: O(n2)
Auxiliary Space: O(1)
Example 2: Printing multiplication table using Python nested for loops
Python3
for i in range ( 2 , 4 ):
for j in range ( 1 , 11 ):
print (i, "*" , j, "=" , i * j)
print ()
|
Output:
2 * 1 = 2
2 * 2 = 4
2 * 3 = 6
2 * 4 = 8
2 * 5 = 10
2 * 6 = 12
2 * 7 = 14
2 * 8 = 16
2 * 9 = 18
2 * 10 = 20
3 * 1 = 3
3 * 2 = 6
3 * 3 = 9
3 * 4 = 12
3 * 5 = 15
3 * 6 = 18
3 * 7 = 21
3 * 8 = 24
3 * 9 = 27
3 * 10 = 30
Time Complexity: O(n2)
Auxiliary Space: O(1)
In the above example what we do is take an outer for loop running from 2 to 3 for multiplication table of 2 and 3 and then inside that loop we are taking an inner for loop that will run from 1 to 10 inside that we are printing multiplication table by multiplying each iteration value of inner loop with the iteration value of outer loop as we see in the below output.
Example 3: Printing using different inner and outer nested loops
Python3
list1 = [ 'I am ' , 'You are ' ]
list2 = [ 'healthy' , 'fine' , 'geek' ]
list2_size = len (list2)
for item in list1:
print ( "start outer for loop " )
i = 0
while (i < list2_size):
print (item, list2[i])
i = i + 1
print ( "end for loop " )
|
Output:
start outer for loop
I am healthy
I am fine
I am geek
end for loop
start outer for loop
You are healthy
You are fine
You are geek
end for loop
Time Complexity: O(n2)
Auxiliary Space: O(1)
In this example, we are initializing two lists with some strings. Store the size of list2 in ‘list2_Size’ using len() function and using it in the while loop as a counter. After that run an outer for loop to iterate over list1 and inside that loop run an inner while loop to iterate over list2 using list indexing inside that we are printing each value of list2 for every value of list1.
Using break statement in nested loops
It is a type of loop control statement. In a loop, we can use the break statement to exit from the loop. When we use a break statement in a loop it skips the rest of the iteration and terminates the loop. let’s understand it using an example.
Code:
Python3
for i in range ( 2 , 4 ):
for j in range ( 1 , 11 ):
if i = = j:
break
print (i, "*" , j, "=" , i * j)
print ()
|
Output:
2 * 1 = 2
3 * 1 = 3
3 * 2 = 6
Time Complexity: O(n2)
Auxiliary Space: O(1)
The above code is the same as in Example 2 In this code we are using a break statement inside the inner loop by using the if statement. Inside the inner loop if ‘i’ becomes equals to ‘j’ then the inner loop will be terminated and not executed the rest of the iteration as we can see in the output table of 3 is printed up to two iterations because in the next iteration ‘i’ becomes equal to ‘j’ and the loop breaks.
Using continue statement in nested loops
A continue statement is also a type of loop control statement. It is just the opposite of the break statement. The continue statement forces the loop to jump to the next iteration of the loop whereas the break statement terminates the loop. Let’s understand it by using code.
Python3
for i in range ( 2 , 4 ):
for j in range ( 1 , 11 ):
if i = = j:
continue
print (i, "*" , j, "=" , i * j)
print ()
|
Output:
2 * 1 = 2
2 * 3 = 6
2 * 4 = 8
2 * 5 = 10
2 * 6 = 12
2 * 7 = 14
2 * 8 = 16
2 * 9 = 18
2 * 10 = 20
3 * 1 = 3
3 * 2 = 6
3 * 4 = 12
3 * 5 = 15
3 * 6 = 18
3 * 7 = 21
3 * 8 = 24
3 * 9 = 27
3 * 10 = 30
Time Complexity: O(n2)
Auxiliary Space: O(1)
In the above code instead of using a break statement, we are using a continue statement. Here when ‘i’ becomes equal to ‘j’ in the inner loop it skips the rest of the code in the inner loop and jumps on the next iteration as we see in the output “2 * 2 = 4” and “3 * 3 = 9” is not printed because at that point ‘i’ becomes equal to ‘j’.
Single line Nested loops using list comprehension
To convert the multiline nested loops into a single line, we are going to use list comprehension in Python. List comprehension includes brackets consisting of expression, which is executed for each element, and the for loop to iterate over each element in the list.
Syntax of List Comprehension:
newList = [ expression(element) for element in oldList if condition ]
Code:
Python3
list1 = [[j for j in range ( 3 )]
for i in range ( 5 )]
print (list1)
|
Output:
[[0, 1, 2], [0, 1, 2], [0, 1, 2], [0, 1, 2], [0, 1, 2]]
In the above code, we are storing a list inside the list using list comprehension in the inner loop of list comprehension [j for j in range(3)] to make a list [0, 1, 2] for every iteration of the outer loop “for i in range(5)”.
Time Complexity: O(n2) It is faster than nested loops
Auxiliary Space: O(n)
Share your thoughts in the comments
Please Login to comment...