Puzzle | 4×4 Grid of Light Switches
Last Updated :
25 Apr, 2024
We are presented with a 4×4 grid of light switches, each capable of being in an on or off state. Toggling a switch alters its state and affects the states of its adjacent switches: those above, below, to the left, and the right. The goal is to discover a sequence of switch toggles that leads to all switches being turned off, starting from an initial arbitrary configuration.
Puzzle | 4×4 Grid of Light Switches
Below, is the code example of Puzzle | 4×4 grid of light switches in Python:
Install Necessary Library
First, we need to install a tkinter library for creating the Puzzle of a 4*4 grid of light switches. To install the tkinter use the below command.
pip install tkinter
Writing Code
In this example, the below code creates a simple GUI using Tkinter in Python, representing a light switch puzzle. It consists of a 5×5 grid of buttons initially displayed as black. Clicking on a button toggles its color between black and yellow, simulating turning a light on or off. The toggle_switch function updates the state of the switches, while the update_lights function visually updates the buttons based on the switch state.
Python3
import tkinter as tk
def toggle_switch(row, col):
if switches[row][col] == 0:
switches[row][col] = 1
else:
switches[row][col] = 0
update_lights()
def update_lights():
for row in range(5):
for col in range(5):
if switches[row][col] == 0:
lights[row][col].configure(bg='black')
else:
lights[row][col].configure(bg='yellow')
window = tk.Tk()
window.title("Light Switch Puzzle")
switches = [[0 for _ in range(5)] for _ in range(5)]
lights = [[None for _ in range(5)] for _ in range(5)]
for row in range(5):
for col in range(5):
switch_button = tk.Button(
window, width=10, height=5, bg='black', command=lambda r=row, c=col: toggle_switch(r, c))
switch_button.grid(row=row, column=col, padx=5, pady=5)
lights[row][col] = switch_button
window.mainloop()
Output:
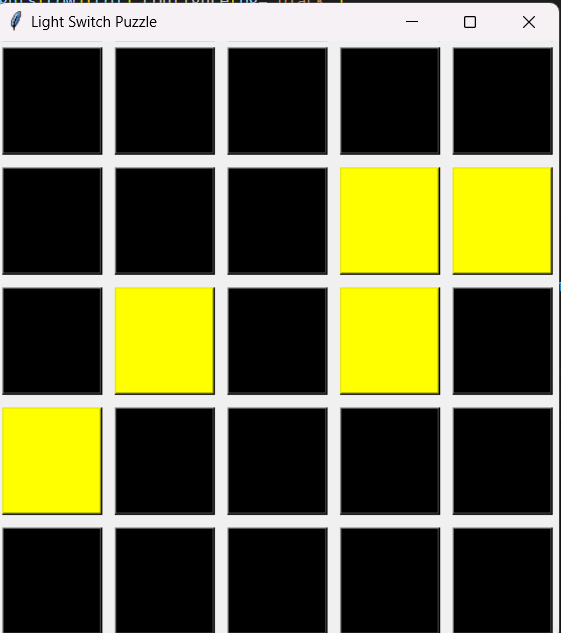
Puzzle | 4×4 grid of light switches
Share your thoughts in the comments
Please Login to comment...