How to use useReducer within a custom hook?
Last Updated :
21 Feb, 2024
useReducer
is one of the fundamental built-in hooks in React, primarily used for managing complex state logic within functional components. Using useReducer
within a custom hook is quite similar to using it in a regular functional component.
Understanding the concept of useReducert within a custom hook:
- Create your custom hook: First, you define a JavaScript function that will be your custom hook. This function can have any name you want and should typically start with “use” to follow the convention. Inside this function, you’ll use
useReducer
to manage state.
- Define your reducer function: Next, you’ll need to define a reducer function. This function takes the current state and an action as arguments and returns the new state based on the action type.
- Call
useReducer
inside your custom hook: Within your custom hook function, you call useReducer
and pass in your reducer function along with an initial state. useReducer
returns the current state and a dispatch function that you can use to dispatch actions to update the state.
- Return state and dispatch from the custom hook: Finally, you return the current state and the dispatch function from your custom hook so that components using the hook can access them.
Example: Below is an example of using useReducer within a custom hook.
Javascript
import React from 'react' ;
import useCounter from './components/useCounter' ;
const CounterComponent = () => {
const { count, increment, decrement } = useCounter(0);
return (
<div>
<h1>Counter App</h1>
<span>{count}</span><br></br>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default CounterComponent;
|
Javascript
import { useReducer } from 'react' ;
const reducer = (state, action) => {
switch (action.type) {
case 'INCREMENT' :
return { count: state.count + 1 };
case 'DECREMENT' :
return { count: state.count - 1 };
default :
return state;
}
};
const useCounter = (initialCount) => {
const [state, dispatch] = useReducer(reducer,
{ count: initialCount });
return {
count: state.count, increment:
() => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' })
};
};
export default useCounter;
|
Output:
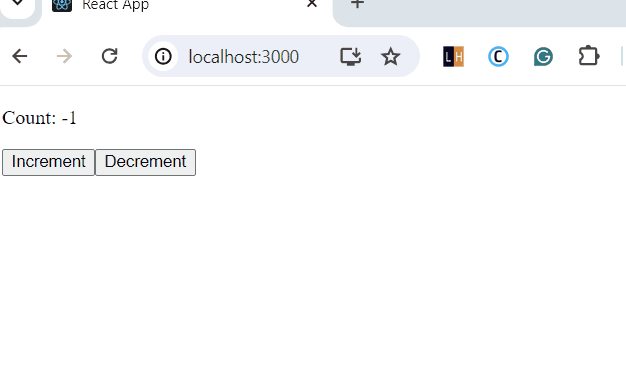
Output
Share your thoughts in the comments
Please Login to comment...