What is the purpose of providedIn in Angular services?
Last Updated :
16 Apr, 2024
When building Angular applications, you’ll often need to share data and logic across different components. This is where services come into play. Services are an easy way to encapsulate functionality and make it available wherever it’s needed in your app. But as your application grows, managing the scope and lifetime of these services becomes crucial. That’s where the providedIn property comes into the picture.
In this article, we will learn more about providedln in detail.
Prerequisites
Understanding the providedIn
The providedIn property is part of the metadata object passed to the @Injectable decorator when defining a service.
Syntax:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root' // Or specify a module: 'my-module'
})
export class MyService {
// Service logic
}
Property Values:
It can take one of the following values:
Value | Description |
---|
root | When set to 'root' , the service is registered in the root injector, making it available throughout the entire application. This means that there will be a single instance of the service shared across all components and modules. |
platform | This value is used for services that need to be registered with the platform injector, which is the injector used for platform-level services. |
Module Name | By providing the name of a specific module (e.g., my-module ), the service is registered only within that module and its child modules. This means that each instance of the module will have its own instance of the service. |
If the providedIn property is not set, the service must be manually registered in the providers array of the module where it will be used.
Features of providedln:
- Scoping: The providedIn property allows you to control the scope of a service, determining where it can be injected and used.
- Singleton: Services registered with providedIn: ‘root’ are singletons, means there is only one instance shared across the entire application.
- Module-level services: By specifying a module name in providedIn, you can create module-level services, which are only available within that module and its child modules.
- Lazy-loaded modules: When using lazy-loaded modules, services can be registered within those modules, ensuring they are not loaded until the module is required.
Steps to Create Angular Application
Step 1: Create a new Angular project
ng new my-app
Step 2: Generate a service
ng generate service my-service
Step 3: Generate a new module using the below command
ng generate module my-module
Folder Structure: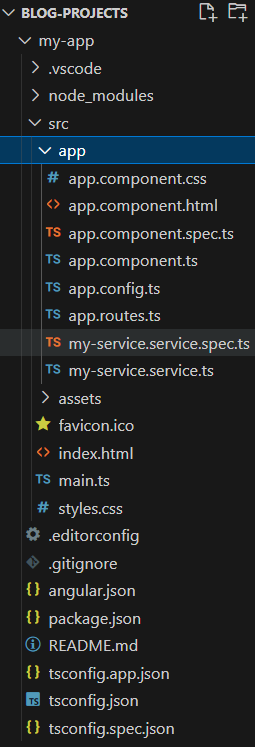
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Example:
JavaScript
//app.component.ts
import { Component } from '@angular/core';
import { MyServiceService } from './my-service.service';
@Component({
selector: 'app-root',
template: `
<h1>{{ message }}</h1>
`
})
export class AppComponent {
message: string;
constructor(private myService: MyServiceService) {
this.message = myService.getMessage();
}
}
JavaScript
//my-module.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { MyServiceService } from '../my-service.service';
@NgModule({
declarations: [],
imports: [
CommonModule
],
providers: [MyServiceService]
})
export class MyModuleModule { }
JavaScript
//my-service.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class MyServiceService {
getMessage() {
return 'Hello from MyServiceService';
}
}
Output:
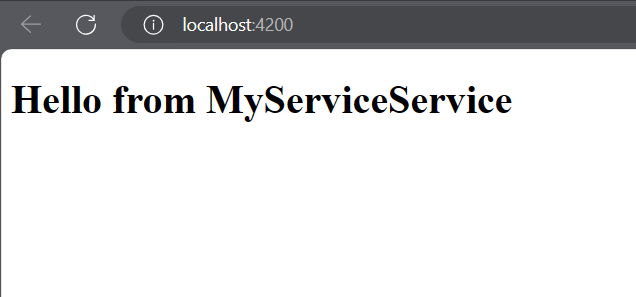
Share your thoughts in the comments
Please Login to comment...